We read every piece of feedback, and take your input very seriously.
To see all available qualifiers, see our documentation.
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
抄一下书
liu-jianhao/Cpp-Design-Patternsgithub.com
动态(组合)地给一个对象增加一些额外的职责。就增加功能而言,Decorator模式比生成子类(继承)更为灵活(消除重复代码 & 减少子类个数)。 ——《设计模式》GoF
https://gist.github.com/Petelin/a7bf8ccfd657536d46aaa355c6dc421agist.github.com
// with interface. which we need create a `point` first. and use it later package main import "fmt" type FibI interface { Fib(n int) int Wrap(fib FibI) FibI } type Fib struct { Wrapper FibI } func (this *Fib) Fib(n int) int { wrapper := this.Wrapper if this.Wrapper == nil { wrapper = this } if n == 0 { return 0 } if n == 1 { return 1 } // call son return wrapper.Fib(n-1) + wrapper.Fib(n-2) } func (this *Fib) Wrap(fib FibI) FibI { this.Wrapper = fib return this } type CacheFib struct { Wrapper FibI cache map[int]int } func (this *CacheFib) Wrap(fib FibI) FibI { this.Wrapper = fib return this } func (this *CacheFib) Fib(n int) int { if this.cache == nil { this.cache = make(map[int]int) } if ans, ok := this.cache[n]; ok { return ans } ans := this.Wrapper.Fib(n) this.cache[n] = ans return ans } type CounterFib struct { Wrapper FibI Counter int } func (this *CounterFib) Wrap(fib FibI) FibI { this.Wrapper = fib return this } func (this *CounterFib) Fib(n int) int { this.Counter++ return this.Wrapper.Fib(n) } func main() { fib := new(Fib) fmt.Println("result fib", fib.Fib(10)) cacheFib := new(CacheFib) counterFib := new(CounterFib) counterCacheFib := cacheFib.Wrap(counterFib.Wrap(fib.Wrap(cacheFib))) fmt.Println("result cache:counter:fib", counterCacheFib.Fib(10)) fmt.Printf("count: %d, cache: %v", counterFib.Counter, cacheFib.cache) }
function 版本
package main import "fmt" type FibI func(int) int var Fib FibI func BaseFib() FibI { return func(n int) int { if n == 0 { return 0 } if n == 1 { return 1 } return Fib(n-1) + Fib(n-2) } } func CounterFib(counter *int, f FibI) FibI { return func(n int) int { *counter++ return f(n) } } func CacheFib(cache map[int]int, f FibI) FibI { return func(n int) int { if ans, ok := cache[n]; ok { return ans } ans := f(n) cache[n] = ans return ans } } func main() { var counter int var cache = make(map[int]int) Fib = CacheFib(cache, CounterFib(&counter, BaseFib())) fmt.Println(Fib(10), counter, cache) }
我们原始需求是写一个fib函数.
现在为了统计调用了多少次需要加一个 counter
为了快一点我们需要加一个cache
...
实现这个有这么几种方式
装饰器模式就是这里的最后一种.
go语言和别的不一样的地方在于, 他没有提供面向对象编程, 在这个例子里就是缺少super , this 机制. 在Java里很容易实现的原因是
functionWrapper1 -> 做自己的事情, 然后通过super 向上传递 -> functionWrapper2 -> 最后类通过this call自己
this这里会变成functionWrape会动态的指向当前对象, 也就是functionWraper1. 而golang没有这个机制, 所以就需要我们自己把整个流程wrap起来.
fib := new(Fib) cacheFib := new(CacheFib) counterFib := new(CounterFib) counterCacheFib := cacheFib.Wrap(counterFib.Wrap(fib.Wrap(cacheFib))) // 通过pipe line把多个装饰器组合在了一起
The text was updated successfully, but these errors were encountered:
Petelin
No branches or pull requests
设计模式 - 装饰器 - 用go来实现
抄一下书
liu-jianhao/Cpp-Design-Patternsgithub.com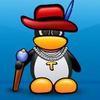
动机(Motivation)
模式定义
动态(组合)地给一个对象增加一些额外的职责。就增加功能而言,Decorator模式比生成子类(继承)更为灵活(消除重复代码 & 减少子类个数)。 ——《设计模式》GoF
要点总结
Show Code
https://gist.github.com/Petelin/a7bf8ccfd657536d46aaa355c6dc421agist.github.com
function 版本
我们原始需求是写一个fib函数.
现在为了统计调用了多少次需要加一个 counter
为了快一点我们需要加一个cache
...
实现这个有这么几种方式
装饰器模式就是这里的最后一种.
go语言和别的不一样的地方在于, 他没有提供面向对象编程, 在这个例子里就是缺少super , this 机制. 在Java里很容易实现的原因是
functionWrapper1 -> 做自己的事情, 然后通过super 向上传递 -> functionWrapper2 -> 最后类通过this call自己
this这里会变成functionWrape会动态的指向当前对象, 也就是functionWraper1. 而golang没有这个机制, 所以就需要我们自己把整个流程wrap起来.
The text was updated successfully, but these errors were encountered: