diff --git a/.github/pull_request_template.md b/.github/pull_request_template.md
index 989195a06..e870fb2d2 100644
--- a/.github/pull_request_template.md
+++ b/.github/pull_request_template.md
@@ -26,3 +26,4 @@ See the framework lifecycle in `packages/contracts/docs/framework-lifecycle` to
- [ ] I have updated the `DEPLOYMENT_CHECKLIST` file in the root folder.
- [ ] I have updated the `UPDATE_CHECKLIST` file in the root folder.
- [ ] I have updated the Subgraph and added a QA URL to the description of this PR.
+- [ ] I have created a follow-up task to update the Developer Portal with the changes made in this PR.
diff --git a/.github/workflows/documentation-update.yml b/.github/workflows/documentation-update.yml
index f91b8a341..5d4fa86af 100644
--- a/.github/workflows/documentation-update.yml
+++ b/.github/workflows/documentation-update.yml
@@ -43,10 +43,13 @@ jobs:
ref: staging
path: developer-portal
token: ${{ secrets.ARABOT_PAT }}
- - name: Remove the docs/osx folder in the developer-portal
- run: rm -rf $GITHUB_WORKSPACE/developer-portal/docs/osx
- - name: Copy the docs/developer-portal folder to the developer-portal
- run: cp -R packages/contracts/docs/developer-portal $GITHUB_WORKSPACE/developer-portal/docs/osx
+ - name: Remove the docs/osx/03-reference-guide folder in the developer-portal, excluding index.md
+ run: |
+ cd $GITHUB_WORKSPACE/developer-portal/docs/osx/03-reference-guide
+ shopt -s extglob
+ rm -rf !(index.md)
+ - name: Copy the docs/developer-portal/03-reference-guide folder to the developer-portal
+ run: cp -R packages/contracts/docs/developer-portal/03-reference-guide $GITHUB_WORKSPACE/developer-portal/docs/osx/03-reference-guide
- name: Get short commit hash
id: hash
run: echo "sha_short=$(git rev-parse --short HEAD)" >> $GITHUB_OUTPUT
diff --git a/.github/workflows/subgraph-documentation-update.yml b/.github/workflows/subgraph-documentation-update.yml
index 7c9153f09..21e8efd58 100644
--- a/.github/workflows/subgraph-documentation-update.yml
+++ b/.github/workflows/subgraph-documentation-update.yml
@@ -42,10 +42,10 @@ jobs:
path: developer-portal
token: ${{ secrets.ARABOT_PAT }}
- name: Remove the docs/subgraph folder in the developer-portal
- run: rm -rf $GITHUB_WORKSPACE/developer-portal/docs/subgraph
+ run: rm -rf $GITHUB_WORKSPACE/developer-portal/versioned_docs/version-1.3.0/osx/subgraph
- name: Copy the docs/developer-portal folder to the developer-portal
- run: cp -R packages/subgraph/docs/developer-portal $GITHUB_WORKSPACE/developer-portal/docs/subgraph
- - name: Copy the generated schema-introspection.json to the developer-portal
+ run: cp -R packages/subgraph/docs/developer-portal $GITHUB_WORKSPACE/developer-portal/versioned_docs/version-1.3.0/osx/subgraph
+ - name: Copy the generated schema-introspection-partial.json to the developer-portal
run: cp -R packages/subgraph/docs/schema-introspection-partial.json $GITHUB_WORKSPACE/developer-portal/static/subgraph
- name: Get short commit hash
id: hash
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/01-dao/01-actions.md b/packages/contracts/docs/developer-portal/01-how-it-works/01-core/01-dao/01-actions.md
deleted file mode 100644
index b9d83de3f..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/01-dao/01-actions.md
+++ /dev/null
@@ -1,138 +0,0 @@
----
-title: Advanced DAO Actions
----
-
-## A Deep Dive into Actions and Execution
-
-The DAO's `execute` function is part of the `DAO.sol` contract and has the following function header:
-
-```solidity title="@aragon/osx/core/dao/DAO.sol"
-function execute(
- bytes32 _callId,
- Action[] calldata _actions,
- uint256 _allowFailureMap
- )
- external
- override
- auth(address(this), EXECUTE_PERMISSION_ID)
- returns (bytes[] memory execResults, uint256 failureMap)
-```
-
-It offers two features that we will dive into in this article:
-
-1. Execution of an array of arbitrary `Action` items.
-2. Allowing failure of individual actions without reverting the entire transaction.
-
-### Actions
-
-In our framework, actions are represented by a solidity struct:
-
-```solidity title="@aragon/osx/core/dao/IDAO.sol"
-/// @notice The action struct to be consumed by the DAO's `execute` function resulting in an external call.
-/// @param to The address to call.
-/// @param value The native token value to be sent with the call.
-/// @param data The bytes-encoded function selector and calldata for the call.
-struct Action {
- address to;
- uint256 value;
- bytes data;
-}
-```
-
-Actions can be
-
-- function calls to the DAO itself (e.g., to upgrade the DAO contract to a newer version of Aragon OSx)
-- function calls to other contracts, such as
-
- - external services (e.g. Uniswap, Compound, etc.)
- - Aragon OSx plugins (e.g., the DAO can be a member of a multisig installed in another DAO),
- - Aragon OSx protocol infrastructure (e.g., to [setup a plugin](../../02-framework/02-plugin-management/02-plugin-setup/index.md))
-
-- transfers of native tokens
-
-#### Example: Calling the wETH Contract
-
-We have an Aragon DAO deployed on the Goerli testnet. Now, we want to wrap `0.1 ETH` from the DAO treasury into `wETH` by depositing it into the [Goerli WETH contract](https://goerli.etherscan.io/token/0xb4fbf271143f4fbf7b91a5ded31805e42b2208d6#writeContract) deployed on the address `0xb4fbf271143f4fbf7b91a5ded31805e42b2208d6`. The corresponding `Action` and `execute` function call look as follows:
-
-```solidity
-
-IDAO.Action[] memory actions = new IDAO.Action[](1);
-
-actions[0] = IDAO.Action({
- to: address(0xB4FBF271143F4FBf7B91A5ded31805e42b2208d6), // The address of the WETH contract on Goerli
- value: 0.1 ether, // The Goerli ETH value to be sent with the function call
- data: abi.encodeCall(IDAO.deposit, ()) // The calldata
-});
-
-dao().execute({_callId: '', _actions: actions, _allowFailureMap: 0});
-
-```
-
-For the `execute` call to work, the caller must have the required [`EXECUTE_PERMISSION_ID` permission](../02-permissions/index.md) on the DAO contract.
-
-### The Action Array
-
-The `Action[] calldata _actions` input argument allows you to execute an array of up to 256 `Action` items in a single transaction within the gas limitations of the Ethereum virtual machine (EVM).
-This is important so that several calls can be executed in a strict order within a single transaction.
-
-Imagine a DAO is currently governed by a multisig (it has the `Multisig` plugin installed) and wants to transition the DAO governance to token voting.
-To achieve this, one of the Multisig members creates a proposal in the plugin proposing to
-
-1. install the `TokenVoting` plugin
-2. uninstall the `Multisig` plugin
-
-If enough multisig signers approve and the proposals passes, the action array can be executed and the transition happens.
-
-Here, it is important that the two actions happen in the specified order and both are required to succeed.
-Otherwise, if the first action would fail or the second one would happen beforehand, the DAO could end up in a state without having a governance plugin enabled.
-
-Accordingly and by default, none of the atomic actions in the action array is allowed to revert. Otherwise, the entire action array is reverted.
-
-### Allowing For Failure
-
-In some scenarios, it makes sense to relax this requirement and allow specific actions in the array to revert.
-Imagine a DAO wants to buy two NFTs A and B with DAI tokens. A proposal is scheduled in a governance plugin to
-
-1. set the DAI allowance to `125`
-2. try to buy NFT A for `100` DAI
-3. try to buy NFT B for `125` DAI
-4. set the DAI allowance to `0`
-
-Once the proposal has passed, it might be that the NFT A or B was already bought by someone else so that action 2 or 3 would revert.
-However, if the other NFT is still available, the DAO might still want to make at least the second trade.
-Either way, the DAO needs to first approve `125` DAO tokens before the trade and wants to set it back to `0` after the potential trades have happened for security reasons.
-
-#### The `allowFailureMap` Input Argument
-
-This optionality can be achieved with the allow-failure feature available in the DAO's `execute` function.
-To specify that failure is allowed for the actions 2 and 3, we provide the following `_allowFailureMap`:
-
-```solidity
-uint256 _allowFailureMap = 6;
-```
-
-and explain why in the following:
-
-In binary representation a `uint256` value is a number with 256 digits that can be 0 or 1. In this representation, the value of `uint256(6)` translates into
-
-```solidity
-... 0 0 0 1 1 0 // the value 6 in binary representation
-... 5 4 3 2 1 0 // the indices of the action array items that are allowed to fail.
-```
-
-where we omitted 250 more leading zeros. This binary value encodes a map to be read from right to left encoding the indices of the action array that are allowed to revert.
-Accordingly, the second and third array item (with the indices 1 and 2) are allowed to fail.
-If we want that every atomic succeeds, we specify a `allowFailureMap` value of `0`.
-
-#### The `failureMap` Output Argument
-
-In analogy and after an action array with a provided allow-failure map was executed successfully in the DAO, the `execute` function returns a corresponding `uint256 failureMap` containing the actual failures that have happened.
-If all actions succeeded, the value `uint256(0)` will be returned.
-If the third action failed, for example, because the NFT was already sold or the DAO had not enough DAI, a failure map value `uint256(4)` is returned
-
-```solidity
-... 0 0 0 1 0 0 // the value 4 in binary representation
-... 5 4 3 2 1 0 // the indices of the action array items that are allowed to fail.
-```
-
-On the frontend, these conversions will be handled automatically.
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/01-dao/index.md b/packages/contracts/docs/developer-portal/01-how-it-works/01-core/01-dao/index.md
deleted file mode 100644
index 2938a7b14..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/01-dao/index.md
+++ /dev/null
@@ -1,62 +0,0 @@
----
-title: DAO
----
-
-## The DAO Contract: The Identity and Basis of Your Organization
-
-In this section, you will learn about the core functionality of every Aragon OSx DAO.
-
-The `DAO` contract is the identity and basis of your organization. It is the address carrying the DAO’s ENS name, metadata, and holding the funds. Furthermore, it has **six base functionalities** being commonly found in other DAO frameworks in the ecosystem.
-
-### 1. Execution of Arbitrary Actions
-
-The most important and basic functionality of your DAO is the **execution of arbitrary actions**, which allows you to execute the DAO's own functions as well as interacting with the rest of the world, i.e., calling methods in other contracts and sending assets to other addresses.
-
-:::note
-Typically, actions are scheduled in a proposal in a governance [plugin installed to your DAO](../03-plugins/index.md).
-:::
-
-Multiple `Action` structs can be put into one `Action[]` array and executed in a single transaction via the `execute` function. To learn more about actions and advanced features of the DAO executor, visit the [A Deep Dive Into Actions](./01-actions.md) section.
-
-### 2. Asset Management
-
-The DAO provides basic **asset management** functionality to deposit, withdraw, and keep track of
-
-- native
-- [ERC-20 (Token Standard)](https://eips.ethereum.org/EIPS/eip-20),
-- [ERC-721 (NFT Standard)](https://eips.ethereum.org/EIPS/eip-721), and
-- [ERC-1155 (Multi Token Standard)](https://eips.ethereum.org/EIPS/eip-1155)
-
-tokens in the treasury.
-In the future, more advanced asset management and finance functionality can be added to your DAO in the form of [plugins](../03-plugins/index.md).
-
-### 3. Upgradeability
-
-Your DAO contract has the ability to be upgraded to a newer version (see [Upgrade your DAO](../../../02-how-to-guides/01-dao/03-protocol-upgrades.md)) if a new version of Aragon OSx is released in the future. These upgrades allow your DAO to smoothly transition to a new protocol version unlocking new features.
-
-
-
-### 4. Callback Handling
-
-To interact with the DAO, external contracts might require certain callback functions to be present.
-Examples are the `onERC721Received` and `onERC1155Received` / `onERC1155BatchReceived` functions required by the [ERC-721 (NFT Standard)](https://eips.ethereum.org/EIPS/eip-721) and [ERC-1155 (Multi Token Standard)](https://eips.ethereum.org/EIPS/eip-1155) tokens.
-Our `CallbackHandler` allows to register the required callback responses dynamically so that the DAO contract does not need to be upgraded.
-
-
-
-### 5. Signature Validation
-
-Currently, externally owned accounts (EOAs) can sign messages with their associated private keys, but contracts cannot.
-An exemplary use case is a decentralized exchange with an off-chain order book, where buy/sell orders are signed messages.
-To accept such a request, both, the external service provider and caller need to follow a standard with which the signed message of the caller can be validated.
-
-By supporting the [ERC-1271](https://eips.ethereum.org/EIPS/eip-1271) standard, your DAO can validate signatures via its `isValidSignature` function that forwards the call to a signature validator contract.
-
-
-
-### 6. Permission Management
-
-Lastly, it is essential that only the right entities (e.g., the DAO itself or trusted addresses) have permission to use the above-mentioned functionalities. This is why Aragon OSx DAOs contain a flexible and battle-tested **permission manager** being able to assign permissions for the above functionalities to specific addresses.
-Although possible, the permissions to execute arbitrary actions or upgrade the DAO should not be given to EOAs as this poses a security risk to the organization if the account is compromised or acts adversarial. Instead, the permissions for the above-mentioned functionalities are better restricted to the `DAO` contract itself and triggered through governance [plugins](../03-plugins/index.md) that you can install on your DAO.
-
-To learn more, visit the [permission manager](../02-permissions/index.md) section.
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/02-permissions/01-conditions.md b/packages/contracts/docs/developer-portal/01-how-it-works/01-core/02-permissions/01-conditions.md
deleted file mode 100644
index 8c38623d3..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/02-permissions/01-conditions.md
+++ /dev/null
@@ -1,210 +0,0 @@
----
-title: Permission Conditions
----
-
-## Permission Conditions
-
-Permission conditions relay the decision if an authorized call is permitted to another contract.
-This contract must inherit from `PermissionCondition` and implement the `IPermissionCondition` interface.
-
-```solidity title="@aragon/osx/core/permission/IPermissionCondition.sol"
-interface IPermissionCondition {
- /// @notice This method is used to check if a call is permitted.
- /// @param _where The address of the target contract.
- /// @param _who The address (EOA or contract) for which the permissions are checked.
- /// @param _permissionId The permission identifier.
- /// @param _data Optional data passed to the `PermissionCondition` implementation.
- /// @return allowed Returns true if the call is permitted.
- function isGranted(
- address _where,
- address _who,
- bytes32 _permissionId,
- bytes calldata _data
- ) external view returns (bool allowed);
-}
-```
-
-By implementing the `isGranted` function, any number of custom conditions can be added to the permission.
-These conditions can be based on
-
-- The specific properties of
-
- - The caller `who`
- - The target `where`
-
-- The calldata `_data` of the function such as
-
- - Function signature
- - Parameter values
-
-- General on-chain data such as
-
- - Timestamps
- - Token ownership
- - Entries in curated registries
-
-- off-chain data being made available through oracle services (e.g., [chain.link](https://chain.link/), [witnet.io](https://witnet.io/)) such as
-
- - Market data
- - Weather data
- - Scientific data
- - Sports data
-
-The following examples illustrate
-
-## Examples
-
-:::caution
-The following code examples serve educational purposes and are not intended to be used in production.
-:::
-
-Let’s assume we have an `Example` contract managed by a DAO `_dao` containing a `sendCoins` function allowing you to send an `_amount` to an address `_to` and being permissioned through the `auth` modifier:
-
-```solidity title="Example.sol"
-contract Example is Plugin {
- constructor(IDAO _dao) Plugin(_dao) {}
-
- function sendCoins(address _to, uint256 _amount) external auth(SEND_COINS_PERMISSION_ID) {
- // logic to send `_amount` coins to the address `_to`...
- }
-}
-```
-
-Let's assume you own the private key to address `0x123456...` and the `Example` contract was deployed to address `0xabcdef...`.
-Now, to be able to call the `sendCoins` function, you need to `grant` the `SEND_COINS_PERMISSION_ID` permission to your wallet address (`_who=0x123456...`) for the `Example` contract (`_where=0xabcdef...`).
-If this is the case, the function call will succeed, otherwise it will revert.
-
-We can now add additional constraints to it by using the `grantWithCondition` function.
-Below, we show four exemplary conditions for different 4 different use cases that we could attach to the permission.
-
-### Condition 1: Adding Parameter Constraints
-
-Let’s imagine that we want to make sure that `_amount` is not more than `1 ETH` without changing the code of `Example` contract.
-
-We can realize this requirement by deploying a `ParameterConstraintCondition` condition.
-
-```solidity title="ParameterConstraintCondition.sol"
-contract ParameterConstraintCondition is PermissionCondition {
- uint256 internal maxValue;
-
- constructor(uint256 _maxValue) {
- maxValue = _maxValue;
- }
-
- function isGranted(
- address _where,
- address _who,
- bytes32 _permissionId,
- bytes calldata _data
- ) external view returns (bool) {
- (_where, _who, _permissionId); // Prevent compiler warnings resulting from unused arguments.
-
- (address _to, uint256 _amount) = abi.decode(_data, (address, uint256));
-
- return _amount <= _maxValue;
-}
-```
-
-Now, after granting the `SEND_COINS_PERMISSION_ID` permission to `_where` and `_who` via the `grantWithCondition` function and pointing to the `ParameterConstraintCondition` condition contract, the `_who` address can only call the `sendCoins` of the `Example` contract deployed at address `_where` successfully if `_amount` is not larger than `_maxValue` stored in the condition contract.
-
-### Condition 2: Delaying a Call With a Timestamp
-
-In another use-case, we might want to make sure that the `sendCoins` can only be called after a certain date. This would look as following:
-
-```solidity title="TimeCondition.sol"
-contract TimeCondition is PermissionCondition {
- uint256 internal date;
-
- constructor(uint256 _date) {
- date = _date;
- }
-
- function isGranted(
- address _where,
- address _who,
- bytes32 _permissionId,
- bytes calldata _data
- ) external view returns (bool) {
- (_where, _who, _permissionId, _data); // Prevent compiler warnings resulting from unused arguments
-
- return block.timestamp > date;
- }
-}
-```
-
-Here, the permission condition will only allow the call the `_date` specified in the constructor has passed.
-
-### Condition 3: Using Curated Registries
-
-In another use-case, we might want to make sure that the `sendCoins` function can only be called by real humans to prevent sybil attacks. For this, let's say we use the [Proof of Humanity (PoH)](https://www.proofofhumanity.id/) registry providing a curated list of humans:
-
-```solidity title="IProofOfHumanity.sol"
-interface IProofOfHumanity {
- function isRegistered(address _submissionID) external view returns (bool);
-}
-
-contract ProofOfHumanityCondition is PermissionCondition {
- IProofOfHumanity internal registry;
-
- constructor(IProofOfHumanity _registry) {
- registry = _registry;
- }
-
- function isGranted(
- address _where,
- address _who,
- bytes32 _permissionId,
- bytes calldata _data
- ) external view returns (bool) {
- (_where, _permissionId, _data); // Prevent compiler warnings resulting from unused arguments
-
- return registry.isRegistered(_who);
- }
-}
-```
-
-Here, the permission condition will only allow the call if the PoH registry confirms that the `_who` address is registered and belongs to a real human.
-
-#### Condition 4: Using a Price Oracle
-
-In another use-case, we might want to make sure that the `sendCoins` function can only be called if the ETH price in USD is above a certain threshold:
-
-
-```solidity title="PriceOracleCondition.sol"
-import '@chainlink/contracts/src/v0.8/interfaces/AggregatorV3Interface.sol';
-
-contract PriceOracleCondition is PermissionCondition {
- AggregatorV3Interface internal priceFeed;
-
- // Network: Goerli
- // Aggregator: ETH/USD
- // Address: 0xD4a33860578De61DBAbDc8BFdb98FD742fA7028e
- constructor() {
- priceFeed = AggregatorV3Interface(
- 0xD4a33860578De61DBAbDc8BFdb98FD742fA7028e
- );
- }
-
- function isGranted(
- address _where,
- address _who,
- bytes32 _permissionId,
- bytes calldata _data
- ) external view returns (bool) {
- (_where, _who, _permissionId, _data); // Prevent compiler warnings resulting from unused arguments
-
- (
- /*uint80 roundID*/,
- int256 price,
- /*uint startedAt*/,
- /*uint timeStamp*/,
- /*uint80 answeredInRound*/
- ) = priceFeed.latestRoundData();
-
- return price > 9000 * 10**18; // It's over 9000!
- }
-}
-
-Here, we use [a data feed from a Chainlink oracle](https://docs.chain.link/docs/data-feeds/) providing us with the latest ETH/USD price on the Goerli testnet and require that the call is only allowed if the ETH price is over $9000.
-
-````
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/02-permissions/index.md b/packages/contracts/docs/developer-portal/01-how-it-works/01-core/02-permissions/index.md
deleted file mode 100644
index 96ca1d1ae..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/02-permissions/index.md
+++ /dev/null
@@ -1,157 +0,0 @@
----
-title: Permissions
----
-
-## Managing Your DAO
-
-At Aragon, we believe that **DAOs are permission management systems**.
-Permissions between contracts and wallets allow a DAO to manage and govern its actions.
-
-Here, you will learn how the permissions in Aragon OSx work, how they can be granted and revoked from wallets and contracts, and how they are managed through the DAO.
-
-As we mentioned earlier, it is essential that only the right person or contract can execute a certain action. As a developer, you might have seen or used [modifiers such as `onlyOwner`](https://docs.openzeppelin.com/contracts/2.x/api/ownership#Ownable) in contracts. This `onlyOwner` modifier provides basic access control to your DAO: only the `owner` address is permitted to execute the function to which the modifier is attached.
-
-In Aragon OSx, we follow the same approach but provide more advanced functionality:
-Each `DAO` contracts includes a `PermissionManager` contract allowing to flexibly, securely, and collectively manage permissions through the DAO and, thus, govern its actions.
-This `PermissionManager`, called `ACL` in previous Aragon OS versions, was one big reason why our protocol never got hacked.
-The code and configuration of a DAO specifies which wallets or contracts (`who`) are allowed to call which authorized functions on a target contract (`where`).
-Identifiers, permissions, and modifiers link everything together.
-
-### Permission Identifiers
-
-To differentiate between different permissions, permission **identifiers** are used that you will frequently find at the top of Aragon OSx contracts. They look something like this:
-
-```solidity title="@aragon/osx/core/dao/DAO.sol"
-bytes32 public constant EXECUTE_PERMISSION_ID = keccak256("EXECUTE_PERMISSION");
-```
-
-### Permissions
-
-A permission specifies an address `who` being allowed to call certain functions on a contract address `where`. In the `PermissionManager` contract, permissions are defined as the concatenation of the word `"PERMISSION"` with the `who` and `where` address, as well as the `bytes32` permission identifier `permissionId`.
-
-```solidity title="@aragon/osx/core/permission/PermissionManager.sol"
-function permissionHash(
- address _where,
- address _who,
- bytes32 _permissionId
-) internal pure returns (bytes32) {
- return keccak256(abi.encodePacked('PERMISSION', _who, _where, _permissionId));
-}
-```
-
-This concatenated information is then stored as `keccak256` hashes inside a mapping like this one:
-
-```solidity title="@aragon/osx/core/permission/PermissionManager.sol"
-mapping(bytes32 => address) internal permissionsHashed;
-```
-
-Here, the `bytes32` keys are the permission hashes and the `address` values are either zero-address flags, such as `ALLOW_FLAG = address(2)` and `UNSET_FLAG = address(0)` indicating if the permission is set, or an actual address pointing to a `PermissionCondition` contract, which is discussed in the next section of this guide.
-
-### Authorization Modifiers
-
-Using **authorization modifiers** is how we make functions permissioned. Permissions are associated with functions by adding the `auth` modifier, which includes the permission identifier in the function’s definition header.
-
-For example, one can call the `execute` function in the DAO when the address making the call has been granted the `EXECUTE_PERMISSION_ID` permission.
-
-```solidity title="@aragon/osx/core/dao/DAO.sol"
-function execute(
- bytes32 callId,
- Action[] calldata _actions,
- uint256 allowFailureMap
-)
- external
- override
- auth(address(this), EXECUTE_PERMISSION_ID)
- returns (bytes[] memory execResults, uint256 failureMap);
-```
-
-### Managing Permissions
-
-To manage permissions, the DAO contract has the `grant`, `revoke` and `grantWithCondition` functions in its public interface.
-
-#### Granting and Revoking Permissions
-
-The `grant` and `revoke` functions are the main functions we use to manage permissions.
-Both receive the `_permissionId` identifier of the permission and the `_where` and `_who` addresses as arguments.
-
-```solidity title="@aragon/osx/core/permission/PermissionManager.sol"
-function grant(
- address _where,
- address _who,
- bytes32 _permissionId
-) external auth(_where, ROOT_PERMISSION_ID);
-```
-
-To prevent these functions from being called by any address, they are themselves permissioned via the `auth` modifier and require the caller to have the `ROOT_PERMISSION_ID` permission in order to call them.
-
-:::note
-Typically, the `ROOT_PERMISSION_ID` permission is granted only to the `DAO` contract itself. Contracts related to the Aragon infrastructure temporarily require it during the [DAO creation](../../02-framework/01-dao-creation/index.md) and [plugin setup ](../../02-framework/02-plugin-management/02-plugin-setup/index.md) processes.
-:::note
-
-This means, that these functions can only be called through the DAO’s `execute` function that, in turn, requires the calling address to have the `EXECUTE_PERMISSION_ID` permission.
-
-:::note
-Typically, the `EXECUTE_PERMISSION_ID` permission is granted to governance contracts (such as a majority voting plugin owned by the DAO or a multi-sig). Accordingly, a proposal is often required to change permissions.
-Exceptions are, again, the [DAO creation](../../02-framework/01-dao-creation/index.md) and [plugin setup ](../../02-framework/02-plugin-management/02-plugin-setup/index.md) processes.
-:::
-
-#### Granting Permission with Conditions
-
-Aragon OSx supports relaying the authorization of a function call to another contract inheriting from the `IPermissionCondition` interface. This works by granting the permission with the `grantWithCondition` function
-
-```solidity title="@aragon/osx/core/permission/PermissionManager.sol"
-function grantWithCondition(
- address _where,
- address _who,
- bytes32 _permissionId,
- PermissionCondition _condition
-) external auth(_where, ROOT_PERMISSION_ID) {}
-```
-
-and specifying the `_condition` contract address. This provides full flexibility to customize the conditions under which the function call is allowed.
-
-Typically, conditions are written specifically for and installed together with [plugins](../../01-core/03-plugins/index.md).
-
-To learn more about this advanced topic and possible applications, visit the [permission conditions](./01-conditions.md) section.
-
-#### Granting Permission to `ANY_ADDR`
-
-In combination with conditions, the arguments `_where` and `_who` can be set to `ANY_ADDR = address(type(uint160).max)`.
-Granting a permission with `_who: ANY_ADDR` has the effect that any address can now call the function so that it behaves as if the `auth` modifier is not present.
-Imagine, for example, you wrote a decentralized service
-
-```solidity
-contract Service {
- function use() external auth(USE_PERMISSION_ID);
-}
-```
-
-Calling the `use()` function inside requires the caller to have the `USE_PERMISSION_ID` permission. Now, you want to make this service available to every user without uploading a new contract or requiring every user to ask for the permission.
-By granting the `USE_PERMISSION_ID` to `_who: ANY_ADDR` on the contract `_where: serviceAddr` you allow everyone to call the `use()` function and you can add more conditions to it. If you later on decide that you want to be more selective about who is allowed to call it, you can revoke the permission to `ANY_ADDR`.
-
-Granting a permission with `_where: ANY_ADDR` to a condition has the effect that is granted on every contract. This is useful if you want to give an address `_who` permission over a large set of contracts that would be too costly or too much work to be granted on a per-contract basis.
-Imagine, for example, that many instances of the `Service` contract exist, and a user should have the permission to use all of them. By granting the `USE_PERMISSION_ID` with `_where: ANY_ADDR`, to some user `_who: userAddr`, the user has access to all of them. If this should not be possible anymore, you can later revoke the permission.
-
-However, some restrictions apply. For security reasons, Aragon OSx does not allow you to use both, `_where: ANY_ADDR` and `_who: ANY_ADDR` in the same permission. Furthermore, the permission IDs of [permissions native to the `DAO` Contract](#permissions-native-to-the-dao-contract) cannot be used.
-Moreover, if a condition is set, we return its `isGranted` result and do not fall back to a more generic one. The condition checks occur in the following order
-
-1. Condition with specific `_who` and specific `where`.
-2. Condition with generic `_who: ANY_ADDR` and specific `_where`.
-3. Condition with specific `_where` and generic `_who: ANY_ADDR`.
-
-### Permissions Native to the `DAO` Contract
-
-The following functions in the DAO are permissioned:
-
-| Functions | Permission Identifier | Description |
-| --------------------------------------- | ------------------------------------------ | --------------------------------------------------------------------------------------------------------------- |
-| `grant`, `grantWithCondition`, `revoke` | `ROOT_PERMISSION_ID` | Required to manage permissions of the DAO and associated plugins. |
-| `execute` | `EXECUTE_PERMISSION_ID` | Required to execute arbitrary actions. |
-| `_authorizeUpgrade` | `UPGRADE_DAO_PERMISSION_ID` | Required to upgrade the DAO (via the [UUPS](https://eips.ethereum.org/EIPS/eip-1822)). |
-| `setMetadata` | `SET_METADATA_PERMISSION_ID` | Required to set the DAO’s metadata and [DAOstar.one DAO URI](https://eips.ethereum.org/EIPS/eip-4824). |
-| `setTrustedForwarder` | `SET_TRUSTED_FORWARDER_PERMISSION_ID` | Required to set the DAO’s trusted forwarder for meta transactions. |
-| `registerStandardCallback` | `REGISTER_STANDARD_CALLBACK_PERMISSION_ID` | Required to register a standard callback for an [ERC-165](https://eips.ethereum.org/EIPS/eip-165) interface ID. |
-
-Plugins installed on the DAO might introduce other permissions and associated permission identifiers.
-
-In the next section, you will learn how to customize your DAO by installing plugins.
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/03-plugins/index.md b/packages/contracts/docs/developer-portal/01-how-it-works/01-core/03-plugins/index.md
deleted file mode 100644
index cfeadcd12..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/03-plugins/index.md
+++ /dev/null
@@ -1,51 +0,0 @@
----
-title: Plugins
----
-
-## Customizing your DAO
-
-To add features beyond the base functionality available, you can customize your Aragon OSx DAO by installing a wide variety of plugins.
-
-Plugins can be related to:
-
-- **Governance:** provides the DAO with different **decision-making** mechanisms such as token or address-based majority voting, conviction voting, optimistic governance, or direct execution from an admin address. They are characterized by requiring the `EXECUTE_PERMISSION_ID` permission on the DAO.
- Advanced governance architectures are possible by having multiple governance plugins simultaneously.
-
-- **Asset Management:** allows the DAO to manage its **treasury** or use it to invest (e.g., in lending, staking, or NFT mints).
-
-- **Membership:** determines **who** will be a part of the DAO and what role they have. This can mean minting governance tokens like [ERC-20](https://eips.ethereum.org/EIPS/eip-20), NFTs, or any other token standard. Typically, membership-related plugins grant permissions based on token ownership or maintenance of a curated list of addresses.
-
-- And **anything** else that comes to mind!
-
-## Understanding Plugins
-
-Whenever a DAO installs a plugin, an instance of that plugin's base template is deployed using the configuration parameters defined by the DAO. For example, you may want to use a specific token for your DAO's voting process, which means you have to determine this within your plugin's configuration parameters.
-
-Each instance of a plugin is installed to a DAO through the granting of permissions.
-
-:::info
-Lern more about the different [plugin types](../../../02-how-to-guides/02-plugin-development/02-plugin-types.md) in our How-to guide.
-:::
-
-This raises questions on how the DAO manages plugins and who actually owns plugins.
-
-### How Does the DAO Manage a Plugin?
-
-A DAO manages plugins and interactions between them. In more detail, its permission manager:
-
-- enables the plugin installation process through the granting and revoking of permissions for the DAO
-- authorizes calls to plugin functions carrying the `auth` modifier
-- authorizes calls to DAO functions, for example the `execute` function, allowing to act as the DAO
-
-
-
-
-
-
- An examplary DAO setup showing interactions between the three core contract pieces triggered by different user groups: The DAO contract in blue containing the PermissionManager in red, respectively, as well as two Plugin contracts in green.
- Function calls are visualized as black arrows and require permission checks (red, dashed arrow). In this example, the permission manager determines whether the token voting plugin can execute actions on the DAO, a member can change its settings, or if a DeFi-related plugin is allowed to invest in a certain, external contract.
-
-
-
-
-Whereas deployed plugin instances belong to the DAO, the developer of the original plugin implementation owns the implementation and setup contract of the plugin. The plugin developer is the maintainer of an Aragon OSx [plugin repo](../../02-framework/02-plugin-management/01-plugin-repo/index.md). Finally, the Aragon OSx protocol manages the registry in which the plugin repositories are listed, which is required to install a plugin using the Aragon OSx framework infrastructure to your DAO.
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/dao-plugin.drawio.svg b/packages/contracts/docs/developer-portal/01-how-it-works/01-core/dao-plugin.drawio.svg
deleted file mode 100644
index 2ffa18af2..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/dao-plugin.drawio.svg
+++ /dev/null
@@ -1,312 +0,0 @@
-
\ No newline at end of file
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/index.md b/packages/contracts/docs/developer-portal/01-how-it-works/01-core/index.md
deleted file mode 100644
index e5d42bef8..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/01-core/index.md
+++ /dev/null
@@ -1,44 +0,0 @@
----
-title: The Smart Contracts behind DAOs
----
-
-## The Contracts Constituting Your DAO
-
-In a nutshell, your Aragon OSx DAO consists of three pieces:
-
-1. **The DAO contract:** The DAO contract is where the **core functionality** of the protocol lies. It is in charge of:
-
- - Representing the identity of the DAO (ENS name, logo, description, other metadata)
- - Keeping the treasury
- - Executing arbitrary actions to
- - Transfer assets
- - Call its own functions
- - Call functions in external contracts
- - Providing general technical utilities (signature validation, callback handling)
-
-2. **The Permission Manager:** The permission manager is part of the DAO contract and the center of our protocol architecture. It **manages permissions for your DAO** by specifying which addresses have permission to call distinct functions on contracts associated with your DAO.
-
-3. **Plugins:** Any custom functionality can be added or removed through plugins, allowing you to **fully customize your DAO**. These plugins can be related to
-
- - Governance (e.g., token voting, one-person one-vote)
- - Asset management (e.g., ERC-20 or NFT minting, token streaming, DeFi)
- - Membership (governing budget allowances, gating access, curating a member list)
-
-The underlying smart contracts constitute **the core contracts** of the Aragon OSx DAO framework.
-
-
-
-
-
-
- An examplary DAO setup showing interactions between the three core contract pieces triggered by different user groups: The DAO contract in blue containing the PermissionManager in red, respectively, as well as two Plugin contracts in green.
- Function calls are visualized as black arrows and require permission checks (red, dashed arrow). In this example, the permission manager determines whether the token voting plugin can execute actions on the DAO, a member can change its settings, or if a DeFi-related plugin is allowed to invest in a certain, external contract.
-
-
-
-
-In the upcoming sections, you will learn about each of them in more depth.
-
-- [The DAO Contract: The Identity and Basis of Your Organization](./01-dao/index.md)
-- [Permissions: Managing Your DAO](./02-permissions/index.md)
-- [Plugins: Customizing your DAO](./03-plugins/index.md)
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/01-dao-creation/index.md b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/01-dao-creation/index.md
deleted file mode 100644
index 8fcbb41d6..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/01-dao-creation/index.md
+++ /dev/null
@@ -1,55 +0,0 @@
----
-title: Creating a DAO
----
-
-## The DAO Creation Process
-
-Two framework contracts manage the `DAO` contract creation process:
-
-- The [`DAOFactory`](../../../03-reference-guide/framework/dao/DAOFactory.md)
-- The [`DAORegistry`](../../../03-reference-guide/framework/dao/DAORegistry.md).
-
-
-
-### `DAOFactory`
-
-The `DAOFactory` creates and sets up a `DAO` for you in four steps with the `createDao` function. The function requires the `DAOSettings` including
-
-- The trusted forwarder address for future [ERC-2771 (Meta Transaction)](https://eips.ethereum.org/EIPS/eip-2771) compatibility that is set to `address(0)` for now
-- The ENS name (to be registered under the `dao.eth` domain)
-- The [ERC-4824 (Common Interfaces for DAOs)](https://eips.ethereum.org/EIPS/eip-4824) `daoURI`
-- Optional metadata
-
-as well as an array of `PluginSettings` containing `PluginSetup` contract references and respective setup data for the initial set of plugins to be installed on the DAO.
-
-The `DAOFactory` create the `DAO` in four steps and interacts with the `DAORegistry` and being also part of the Aragon OSx framework:
-
-1. Creates a new DAO by deploying an [ERC-1967](https://eips.ethereum.org/EIPS/eip-1967) proxy pointing to the latest Aragon OSx `DAO` impelementation and becomes the initial owner.
-
-2. Registers the new contract in the [`DAORegistry`](#daoregistry).
-
-3. Installs the plugins using the `PluginSetupProcessor` (see also the section about [the plugin setup process](../02-plugin-management/02-plugin-setup/index.md)).
-
-4. Sets the [native permissions](../../01-core/02-permissions/index.md#permissions-native-to-the-dao-contract) of the `DAO` and revokes its own ownership.
-
-For more details visit the [`DAOFactory` reference guide entry](../../../03-reference-guide/framework/dao/DAOFactory.md).
-
-### `DAORegistry`
-
-The `DAORegistry` is used by the `DAOFactory` and contains the `register` function
-
-```solidity title="@aragon/framework/dao/DAORegistry.sol"
-function register(
- IDAO dao,
- address creator,
- string calldata subdomain
-) external auth(REGISTER_DAO_PERMISSION_ID);
-```
-
-requiring the `REGISTER_DAO_PERMISSION_ID` permission currently held only by the `DAOFactory`.
-
-If the requested ENS `subdomain` name [is valid](../03-ens-names.md) and not taken, the `DAORegistry` registers the subdomain and adds the `DAO` contract address to the `DAORegistry`.
-If the `subdomain` parameter is non-empty (not `""`) and still available, the ENS name will be registered.
-If the registration was successful, the DAO name, contract and creator addresses are emitted in an event.
-
-For more details visit the [`DAORegistry` reference guide entry](../../../03-reference-guide/framework/dao/DAORegistry.md).
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/01-plugin-repo/01-plugin-repo-creation.md b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/01-plugin-repo/01-plugin-repo-creation.md
deleted file mode 100644
index dcb875a88..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/01-plugin-repo/01-plugin-repo-creation.md
+++ /dev/null
@@ -1,65 +0,0 @@
----
-title: Publishing a Plugin
----
-
-## Start publishing your Plugin by creating a PluginRepo
-
-To be available for installation in the Aragon OSx framework, a `PluginRepo` must be created for each plugin. The `PluginRepo` creation process is handled by:
-
-- The [`PluginRepoFactory`](../../../../03-reference-guide/framework/plugin/repo/PluginRepoFactory.md): who creates the `PluginRepo` instance for each plugin to hold all plugin versions
-- The [`PluginRepoRegistry`](../../../../03-reference-guide/framework/plugin/repo/PluginRepoRegistry.md): who registers the Plugin into the Protocol for DAOs to install.
-
-
-
-### The `PluginRepoFactory` Contract
-
-The `PluginRepoFactory` is the contract in charge of creating the first version of a plugin. It does this through the `createPluginRepoWithFirstVersion` function which creates a `PluginRepo` instance for the plugin with the first release and first build (`v1.1`).
-
-```solidity title="@aragon/framework/repo/PluginRepoFactory.sol"
-/// @notice Creates and registers a `PluginRepo` with an ENS subdomain and publishes an initial version `1.1`.
-/// @param _subdomain The plugin repository subdomain.
-/// @param _pluginSetup The plugin factory contract associated with the plugin version.
-/// @param _maintainer The plugin maintainer address.
-/// @param _releaseMetadata The release metadata URI.
-/// @param _buildMetadata The build metadata URI.
-/// @dev After the creation of the `PluginRepo` and release of the first version by the factory, ownership is transferred to the `_maintainer` address.
-function createPluginRepoWithFirstVersion(
- string calldata _subdomain,
- address _pluginSetup,
- address _maintainer,
- bytes memory _releaseMetadata,
- bytes memory _buildMetadata
-) external returns (PluginRepo pluginRepo);
-```
-
-It also registers the plugin in the Aragon OSx `PluginRepoRegistry`contract with an [ENS subdomain](../../03-ens-names.md) under the `plugin.dao.eth` domain managed by Aragon.
-
-Additional to the information required by the [`createVersion` function discussed earlier](./index.md#the-pluginrepo-contract), it receives:
-
-- A valid ENS `_subdomain` unique name composed of letters from a-z, all in lower caps, separated by a `-`. For ex: `token-voting-plugin`.
-- The address of the plugin repo maintainer who ends up having the `ROOT_PERMISSION_ID`, `MAINTAINER_PERMISSION_ID`, and `UPGRADE_REPO_PERMISSION_ID` permissions. These permissions enable the maintainer to call the internal `PermissionManager`, the `createVersion` and `updateReleaseMetadata` functions as well as upgrading the plugin contract.
-
-For more details visit the [`PluginRepoFactory` Reference Guide entry](../../../../03-reference-guide/framework/plugin/repo/PluginRepoFactory.md).
-
-### The `PluginRepoRegistry` Contract
-
-The `PluginRepoRegistry` contract is the central contract listing all the plugins managed through the Aragon OSx protocol. The `PluginRepoFactory` calls on the `PluginRepoRegistry` to register the plugin in the Aragon OSx protocol.
-
-```solidity title="@aragon/framework/PluginRepoRegistry.sol"
-/// @notice Registers a plugin repository with a subdomain and address.
-/// @param subdomain The subdomain of the PluginRepo.
-/// @param pluginRepo The address of the PluginRepo contract.
-function registerPluginRepo(
-string calldata subdomain,
-address pluginRepo
-) external auth(REGISTER_PLUGIN_REPO_PERMISSION_ID) {
- ...
-}
-```
-
-For more details visit the [`PluginRepoRegistry` reference guide entry](../../../../03-reference-guide/framework/plugin/repo/PluginRepoRegistry.md).
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/01-plugin-repo/index.md b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/01-plugin-repo/index.md
deleted file mode 100644
index fd58973eb..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/01-plugin-repo/index.md
+++ /dev/null
@@ -1,57 +0,0 @@
----
-title: Plugin Repositories
----
-
-## What are Plugin Repositories?
-
-Each plugin has its own Plugin Repository, unique ENS name, and on-chain repository contract, the `PluginRepo`, in which different versions of the plugin are stored for reference using verstion tags constituted by a **release** and **build** number.
-
-Different versions might contain:
-
-- bug fixes
-- new features
-- breaking changes
-
-`PluginRepo` contracts themselves, each associated with a different plugin, are registered in the Aragon OSx [`PluginRepoRegistry`](./01-plugin-repo-creation.md#the-pluginreporegistry-contract) and carry their own [ENS name](../../03-ens-names.md) that the creator chooses. The [`PluginRepoRegistry` contract](./01-plugin-repo-creation.md#the-pluginreporegistry-contract) is described in the upcoming subsection.
-
-
-
-
-
-
- Overview of the plugin versioning and registry in the Aragon OSx protocol. The `PluginRepoRegistry` contract, which is a curated list of ENS named `PluginRepo` contracts, is shown on the left. Each `PluginRepo` contract maintains a list of versions of the `PluginSetup` contract (internally referencing the `Plugin` implementation contract) and the associated UI building blocks as a URI, examplarily shown on the right.
-
-
-
-
-### The `PluginRepo` Contract
-
-The `PluginRepo` contract versions the releases of a `Plugin`. The first version of a plugin is always published as release 1 and build 1 (version tag `1.1`).
-When you publish the first plugin version, a new plugin repository is automatically created for you by the Aragon OSx protocol in which you are the maintainer. The creation process is described in the [plugin repo creation process](./01-plugin-repo-creation.md) section.
-
-The `PluginRepo` contract is [UUPS upgradeable](https://eips.ethereum.org/EIPS/eip-1822), inherits from the [`PermissionManager`](../../../01-core/02-permissions/index.md) and allows the maintainer of the repository to create new versions with the `createVersion` function:
-
-```solidity title="@aragon/framework/repo/PluginRepo.sol"
-/// @notice Creates a new plugin version as the latest build for an existing release number or the first build for a new release number for the provided `PluginSetup` contract address and metadata.
-/// @param _release The release number.
-/// @param _pluginSetupAddress The address of the plugin setup contract.
-/// @param _buildMetadata The build metadata URI.
-/// @param _releaseMetadata The release metadata URI.
-function createVersion(
- uint8 _release,
- address _pluginSetup,
- bytes calldata _buildMetadata,
- bytes calldata _releaseMetadata
-) external auth(address(this), MAINTAINER_PERMISSION_ID);
-```
-
-The function receives four input arguments:
-
-1. The `_release` number to create the build for. If the release number exists already (e.g., release `1`), it is registered as the latest build (e.g., `1.3` if the previous build was `1.2`). If it is a new release number, the build number is `1` (e.g., `2.1`).
-2. The address of `PluginSetup` contract internally referencing the implementation contract (to copy, proxy, or clone from it) and taking care of [installing, updating to, and uninstalling](../02-plugin-setup/index.md) this specific version.
-3. The `_buildMetadata` URI pointing to a JSON file containing the UI data, setup data, and change description for this specific version.
-4. The `_releaseMetadata` URI pointing to a JSON file containing the plugin name, description, as well as optional data such as images to be shown in the aragonApp frontend.
-
-Other functions present in the contract allow you to query previous versions and to update the release metadata. For more details visit the [`PluginRepo` reference guide entry](../../../../03-reference-guide/framework/plugin/repo/PluginRepo.md).
-
-The `PluginRepo` is created for you when you publish the `PluginSetup` contract of your first version to the Aragon OSx protocol, which is explained in the next section: [The Plugin Repo Creation Process](01-plugin-repo-creation.md).
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/01-plugin-repo/plugin-repo-overview.drawio.svg b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/01-plugin-repo/plugin-repo-overview.drawio.svg
deleted file mode 100644
index 62793afc9..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/01-plugin-repo/plugin-repo-overview.drawio.svg
+++ /dev/null
@@ -1,351 +0,0 @@
-
\ No newline at end of file
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/01-security-risk-mitigation.md b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/01-security-risk-mitigation.md
deleted file mode 100644
index 33015ebe9..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/01-security-risk-mitigation.md
+++ /dev/null
@@ -1,63 +0,0 @@
----
-title: Plugin Security and Risks
----
-
-## Risks and Their Mitigation
-
-Extending the functionality of your DAO in the form of plugins can introduce risks, particularly, if this code comes from unaudited and untrusted sources.
-
-### Risks
-
-If a plugin has a bug or vulnerability that can be exploited, this can result in loss of funds or compromise the DAO.
-
-Besides, standard vulnerabilities such as
-
-- Re-entrancy
-- Default function visibility
-- Leaving contracts uninitialized
-- Time or oracle manipulation attacks
-- Integer overflow & underflow
-
-that might be carelessly or intentionally caused, a malicious plugin can hide **backdoors** in its code or request **elevated permissions** in the installation, upgrade, or uninstallation process to the attacker.
-
-#### Backdoors
-
-- [metamorpic contracts](https://a16zcrypto.com/metamorphic-smart-contract-detector-tool/) (contracts, that can be redeployed with new code to the same address)
-- malicious repurposing of storage in an update of an upgradeable plugin contract
-
-
-
-#### Permissions
-
-Examples for elevated permissions, are the [permissions native to the DAO contract](../../../01-core/02-permissions/index.md#permissions-native-to-the-dao-contract) such as
-
-- `ROOT_PERMISSION_ID`
-- `EXECUTE_PERMISSION_ID`
-- `UPGRADE_DAO_PERMISSION_ID`
-
-That should never be granted to untrusted addresses as they can be used to take control over your DAO.
-
-Likewise, one must be careful to not lock your DAO accidentally by
-
-- uninstalling the last governance plugin with `EXECUTE_PERMISSION_ID` permission
-- revoking the `ROOT_PERMISSION_ID` permission from itself or
-- choosing governance settings and execution criteria that most likely can never be met (e.g., requiring 100% participation for a token vote to pass)
-
-### Mitigation
-
-To mitigate the risks mentioned above, proposals requesting the setup of one or multiple plugins must be carefully examined and reviewed by inspecting
-
-- The implementation contract
-- The setup contract, i.e.,
- - The installation and deployment logic
- - The requested permission
- - The helper contracts accompanying the plugin
-- The UI components, i.e.,
- - Misleading (re-)naming of input fields, buttons, or other elements
-
-Generally, we recommend only installing plugins from trusted, verified sources such as those verified by Aragon.
-
-More information can be found in the How-to guides
-
-- [Operating your DAO](../../../../02-how-to-guides/01-dao/index.md)
-- [Developing a Plugin](../../../../02-how-to-guides/02-plugin-development/index.md)
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/index.md b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/index.md
deleted file mode 100644
index 330b81fa3..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/index.md
+++ /dev/null
@@ -1,98 +0,0 @@
----
-title: Installing Plugins
----
-
-## The Smart Contracts Behind Plugins
-
-A DAO can be set up and customized by the **installation, update, and uninstallation** of plugins. Plugins are composed of two key contracts:
-
-- **Plugin contract:** contains the plugin's implementation logic; everything needed to extend the functionality for DAOs.
-- **Plugin Setup contract:** contains the instructions needed to install, update, and uninstall the plugin into the DAO. This is done through granting or revoking permissions, enabling plugins to perform actions within the scope of the DAO.
-
-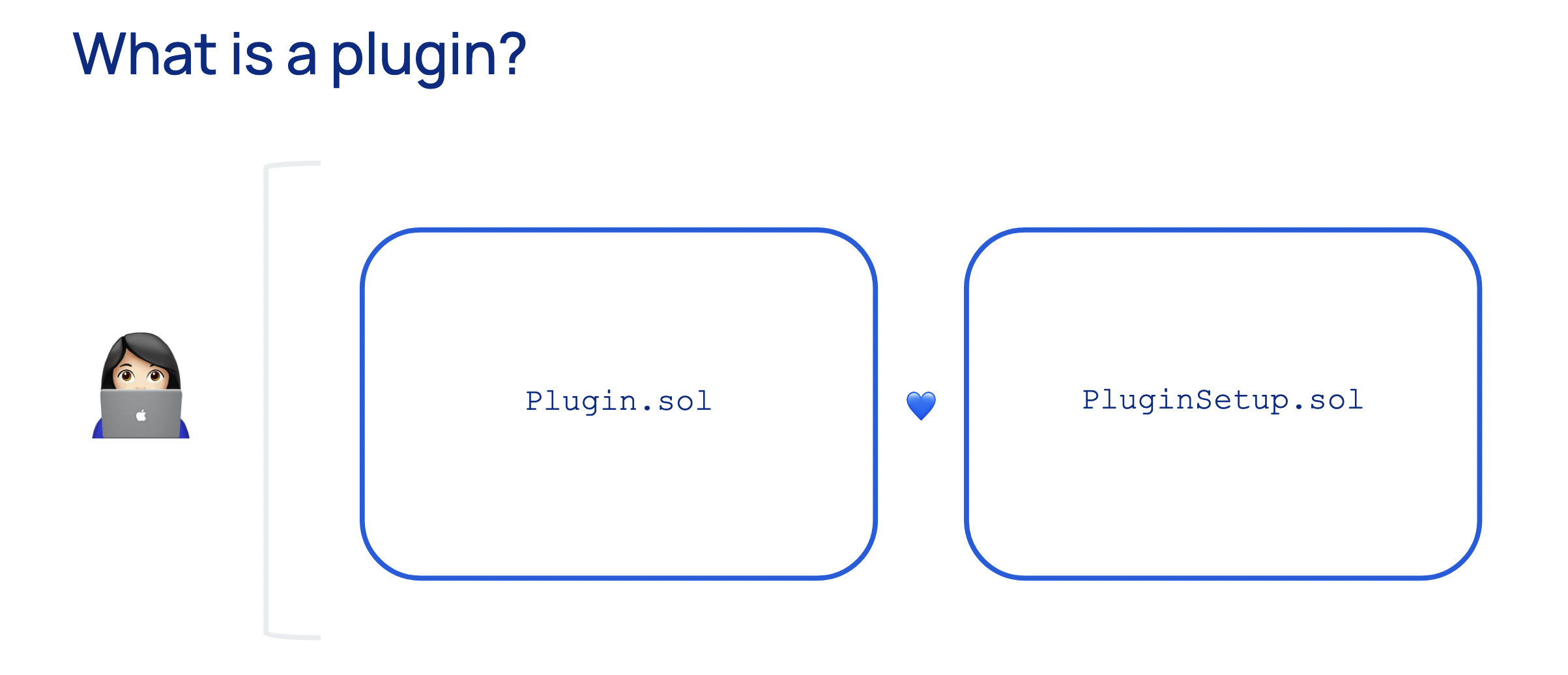
-
-How this works:
-
-- Although a Plugin is composed by the `Plugin` and `PluginSetup` contracts, the Aragon OSx protocol only knows of the `PluginSetup` contract.
-- Since the `PluginSetup` contract is the one containing the plugin installation instructions, it is the one in charge of deploying the Plugin instance. Each plugin instance is specific to that DAO, deployed with its own unique parameters. You can review how to build a `PluginSetup` contract [here](../../../../02-how-to-guides/02-plugin-development/index.md).
-- The `PluginSetup` contract then interacts with the Aragon OSx framework's `PluginSetupProcessor` contract, which is in charge of applying the installion, update, or uninstallation of a plugin into a DAO.
-- Publishing a Plugin into the Aragon OSx protocol is done through creating the first version of the plugin's `PluginRepo`. The plugin's `PluginRepo` instance stores all plugin versions. You can read more about that [here](../../../../02-how-to-guides/02-plugin-development/07-publication/index.md).
-- Except for the gas costs required, plugins are completely free to install, unless decided otherwise by the developer.
-
-### How are Plugins installed in DAOs?
-
-The `PluginSetup` processing is **security critical** because the permissions it handles are granted to third-party contracts.
-
-**Safety is our top priority in the design of the whole protocol.** We want to make sure that the DAO members know exactly what permissions are granted to whom before any processing takes place.
-
-This is why we see the installation process in two phases:
-
-1. **Preparation:** Defining the parameters to be set on the new plugin instance and helpers, as well as requesting the permissions needed for it to work properly. The `PluginSetup` contains the setup script where developers can perform any unprivileged operations. These will need a privileged confirmation in the next step.
-2. **Application:** The granting or revoking of the plugin's requested permissions (based on the preparation step above). This is a privileged action performed by Aragon's `PluginSetupProcessor` (you can understand it as the "installer"), so that the plugin becomes effectively installed or uninstalled. It gets executed whenever someone with `ROOT` privileges on the DAO applies it (most likely through a proposal).
-
-The `PluginSetupProcessor` is the Aragon contract in charge of invoking the `prepareInstallation()` function from your plugin's `PluginSetup` contract and use it to prepare the installation and (eventually) apply it once it has been approved by the DAO.
-
-#### What happens during the Plugin Preparation?
-
-The preparation of a `PluginSetup` contract proceeds as follows:
-
-1. A DAO builder selects a plugin to install, uninstall, or update.
-
-2. The DAO builder defines the parameters and settings that he/she wants for their DAO. Depending on the case, the `prepareInstallation`, `prepareUpdate`, or `prepareUninstallation` method in the `PluginSetup` contract is called through the `PluginSetupProcessor` (and creates a unique setup ID).
-
-3. The [`PluginSetup`](https://github.com/aragon/osx/blob/develop/packages/contracts/src/framework/plugin/setup/PluginSetupProcessor.sol) contract deploys all the contracts and gathers addresses and other input arguments required for the installation/uninstallation/upgrade instructions. This can include:
-
- - deployment of new contracts
- - initialization of new storage variables
- - deprecating/decomissioning outdated (helper) contracts
- - governance settings or other attributes
- - ...
-
- Because the addresses of all associated contracts are now known, a static permission list can be emitted, hashed, and stored on-chain.
-
-4. Once the Plugin installation has been prepared, we use it as the parameter of the `applyInstallation()` action. Once encoded, this action is what must be added to the `Action[]` array of the installation proposal. That way, when the proposal passes, the action becomes executable and the plugin can be installed in the DAO using the parameters defined in the prepare installation process. For a plugin to be installed, it needs to be approved by the governance mechanism (plugin) of the organization, passed as the encoded action of a proposal, and executed by a signer.
-
-:::info
-The governance plugin can be a simple majority vote, an optimistic process or an admin governance plugin that does not involve a waiting period. It can be any governance mechanism existing within the DAO which has access to the DAO's `execute` permission.
-:::
-
-This gives the DAO members the opportunity to check which permissions the `PluginSetup` contract request before granting/revoking them.
-
-Plugin setup proposals must be carefully examined as they can be a potential security risk if the `PluginSetup` contract comes from an untrusted source. To learn more visit the [Security](./01-security-risk-mitigation.md) section.
-
-
-
-#### What happens during the Preparation Application?
-
-After this initial preparation transaction, the addresses and permissions related to the plugin become apparent. The members of a governance plugin with permissions can decide if the installation proposal should be accepted or denied.
-
-Once the proposal has passed, the actions specified in the `Action[]` array can get executed and the `applyInstallation()` action is used to complete the installation of the plugin into the DAO.
-
-This is processed as follows:
-
-1. The DAO temporarily grants the `ROOT_PERMISSION_ID` permission to the `PluginSetupProcessor`. This is needed so that the processor can modify the DAO's permissions settings to set up the plugin.
-2. This `Action` calls the `applyInstallation`, `applyUpdate`, or `applyUninstallation` method in the `PluginSetupProcessor`, containing the list of requested permissions as argument. The permissions hash is compared with the stored hash to make sure that no permission was changed.
- In addition to the above, the update process also upgrades the logic contract to which the proxy points too.
-3. If the hash is valid, the list is processed and `PluginSetupProcessor` conducts the requested sequence of `grant`, `grantWithCondition` and `revoke` calls on the owning DAO.
- Finally, the `PluginSetupProcessor` asks the DAO to revoke the `ROOT_PERMISSION_ID` permission from itself.
-
-:::info
-The two-step setup procedure in Aragon OSx is not limited to the setup of only one plugin — you can **setup multiple plugins at once** by first preparing them in a single proposal and then processing the entire setup sequence in one transaction. This is powerful and allows you to **transform your entire DAO in one proposal**, for example, to install a new governance plugin (e.g., a gasless ZK-vote) and finance plugin (e.g., to stream loans to your members), while uninstalling your old ERC20 token vote in one go.
-:::
-
-In the next sections, you will learn about how plugins are curated on Aragon's repository.
-
-
-
-**a.** 
-**b.** 
-**c.** 
-
-
- Simplified overview of the two-transaction plugin a. installation, b. update, and c. uninstallation process with the involved contracts as rounded rectangles, interactions between them as arrows, and relations as dashed lines. The first and second transaction are distinguished by numbering as well as solid and dotted lines, respectively.
-
-
-
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/plugin-installation.drawio.svg b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/plugin-installation.drawio.svg
deleted file mode 100644
index d25b34465..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/plugin-installation.drawio.svg
+++ /dev/null
@@ -1,447 +0,0 @@
-
\ No newline at end of file
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/plugin-uninstallation.drawio.svg b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/plugin-uninstallation.drawio.svg
deleted file mode 100644
index dfb2ef572..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/plugin-uninstallation.drawio.svg
+++ /dev/null
@@ -1,468 +0,0 @@
-
\ No newline at end of file
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/plugin-update.drawio.svg b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/plugin-update.drawio.svg
deleted file mode 100644
index e92a3b128..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/plugin-update.drawio.svg
+++ /dev/null
@@ -1,523 +0,0 @@
-
\ No newline at end of file
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/index.md b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/index.md
deleted file mode 100644
index e26b10cc2..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/index.md
+++ /dev/null
@@ -1,53 +0,0 @@
----
-title: Plugins
----
-
-## Plugins
-
-As mentioned earlier, plugins built by Aragon and third-party developers can be added and removed from your DAO to adapt it to your needs.
-
-The management of these plugins is handled for you by the Aragon OSx protocol so that the process of
-
-- Releasing new plugins as well as
-- Installing, updating, and uninstalling them to your DAO
-
-becomes as streamlined as possible.
-
-In the following, we learn what a plugin consists of.
-
-
-
-### What Does a Plugin Consist Of?
-
-An Aragon OSx Plugin consists of:
-
-- The `PluginSetup` contract
-
- - referencing the `Plugin` implementation internally
- - containing the setup instruction to install, update, and uninstall it to an existing DAO
-
-- A metadata URI pointing to a `JSON` file containing the
-
- - AragonApp frontend information
- - Information needed for the setup ABI
-
-- A version tag consisting of a
-
- - Release number
- - Build number
-
-A detailed explanation of the [build and release versioning](../../../02-how-to-guides/02-plugin-development/07-publication/01-versioning.md) is found in the How-to sections in our developer portal.
-
-
-
-
-
-
- A schematic depiction of a plugin bundle consisting of a version tag, the plugin setup contract pointing to the plugin implementation contract, and a metadata URI.
-
-
-
-
-The `PluginSetup` is written by you, the plugin developer. The processing of the setup is managed by the `PluginSetupProcessor`, the central component of the setup process in the Aragon OSx framework, which is explained in the section [The Plugin Setup Process](./02-plugin-setup/index.md).
-
-Each plugin with its different builds and releases is versioned inside its own plugin repositories in a `PluginRepo` contract, which is explained in the next section.
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/plugin-version.drawio.svg b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/plugin-version.drawio.svg
deleted file mode 100644
index 05d6c84ce..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/02-plugin-management/plugin-version.drawio.svg
+++ /dev/null
@@ -1,155 +0,0 @@
-
\ No newline at end of file
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/03-ens-names.md b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/03-ens-names.md
deleted file mode 100644
index 36446e457..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/03-ens-names.md
+++ /dev/null
@@ -1,21 +0,0 @@
----
-title: ENS Names
----
-
-## Unique DAO and Plugin Repo Names
-
-To make DAOs and plugin repositories easily identifiable in the Aragon OSx ecosystem, we assign unique ENS names to them upon registration during the [DAO creation](./01-dao-creation/index.md) and [plugin publishing](./02-plugin-management/01-plugin-repo/01-plugin-repo-creation.md) processes.
-
-:::info
-You can skip registering an ENS name for your DAO under the `dao.eth` by leaving the [`DAOSettings.subdomain` field](../../03-reference-guide/framework/dao/DAOFactory.md#public-struct-daosettings) empty when calling the [`createDao`](../../03-reference-guide/framework/dao/DAOFactory.md#external-function-createdao) function.
-:::
-
-### Allowed Character Set
-
-We allow the following characters for the subdomain names:
-
-- Lowercase letters `a-z`
-- Digits `0-9`
-- The hyphen `-`
-
-This way, you can name and share the DAO or plugin repo you have created as `my-cool.dao.eth` or `my-handy.plugin.dao.eth` to make their addresses easily shareable and discoverable on ENS-supporting chains.
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/aragon-os-infrastructure-core-overview.drawio.svg b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/aragon-os-infrastructure-core-overview.drawio.svg
deleted file mode 100644
index 23595b18d..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/aragon-os-infrastructure-core-overview.drawio.svg
+++ /dev/null
@@ -1,822 +0,0 @@
-
\ No newline at end of file
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/index.md b/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/index.md
deleted file mode 100644
index adc424a25..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/02-framework/index.md
+++ /dev/null
@@ -1,29 +0,0 @@
----
-title: Framework - How Everything Connects
----
-
-## The Infrastructure Running the Aragon OSx Protocol
-
-The Aragon OSx protocol is composed of **framework-related contracts** creating and managing the **core contracts**. This includes the
-
-- [Creation of DAOs](01-dao-creation/index.md) and initial plugin configuration
-- [Management of plugins](02-plugin-management/index.md), which includes the
-
- - The setup in existing DAOs
- - The versioning of different implementations and respective setup contracts, UI, and related metadata
-
-- [Assignment of ENS Names](./03-ens-names.md) to `Plugin` and `DAO` contracts created through the framework
-
-An overview of the involved contracts and their interactions is shown below:
-
-
- Overview of the framework and core contracts of the Aragon OSx protocol.
-
-
-
-
-In the following sections, you will learn more about the framework-related contracts of the Aragon OSx protocol.
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/03-framwork-dao.md b/packages/contracts/docs/developer-portal/01-how-it-works/03-framwork-dao.md
deleted file mode 100644
index bf7ee42f9..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/03-framwork-dao.md
+++ /dev/null
@@ -1,11 +0,0 @@
----
-title: Protocol Governance
----
-
-## Governing the Aragon OSx Framework
-
-To govern the framework infrastructure, a **Framework DAO** was deployed.
-
-The Framework DAO controls the permissions of and between the framework-related contracts required to configure and maintain them as well as to replace or upgrade them.
-
-This Framework DAO constitutes the **governance layer** of the Aragon OSx DAO Framework.
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/aragon-os-framework-overview.drawio.svg b/packages/contracts/docs/developer-portal/01-how-it-works/aragon-os-framework-overview.drawio.svg
deleted file mode 100644
index e16654ece..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/aragon-os-framework-overview.drawio.svg
+++ /dev/null
@@ -1,515 +0,0 @@
-
\ No newline at end of file
diff --git a/packages/contracts/docs/developer-portal/01-how-it-works/index.md b/packages/contracts/docs/developer-portal/01-how-it-works/index.md
deleted file mode 100644
index edd79c1c9..000000000
--- a/packages/contracts/docs/developer-portal/01-how-it-works/index.md
+++ /dev/null
@@ -1,29 +0,0 @@
----
-title: How It Works
----
-
-## The Aragon OSx DAO Framework
-
-The Aragon OSx protocol is a DAO framework structured as follows:
-
-
-
-
- Overview of the Aragon OSx protocol with its structural components and their responsibilities: the governance layer constituted by the framework DAO, the code layer including the framework and core contracts, which depends on external libraries and services
-
-
-
-### Code Layer
-
-The foundation of the Aragon OSx protocol is the **code layer** constituted by the core and framework related contracts.
-The [core contracts](./01-core/index.md) provide the core primitives intended to be used by users and implemented by developers of the DAO framework.
-The [framework contracts](./02-framework/index.md) provide the infrastructure to easily create and manage your DAOs and plugins easy.
-Both are built on top of external dependencies, most notably the [OpenZeppelin](https://www.openzeppelin.com/contracts) and the [Ethereum Name Service (ENS)](https://docs.ens.domains/) contracts.
-
-The core and framework contracts are free to use, and no additional fees are charged.
-
-### Governance Layer
-
-To govern the framework infrastructure, an Aragon OSx [Framework DAO](./03-framwork-dao.md) is deployed constituting the **governance layer** of the Aragon OSx protocol.
-
-In the next sections, you will learn more about the individual components of the framework.
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/01-best-practices.md b/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/01-best-practices.md
deleted file mode 100644
index 987d064b9..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/01-best-practices.md
+++ /dev/null
@@ -1,22 +0,0 @@
----
-title: Best Practices
----
-
-## Some Advice When Operating your DAO
-
-### DOs 👌
-
-- Make sure that at least one address (typically a governance plugin) has `EXECUTE_PERMISSION_ID` permission so that something can be executed on behalf of the DAO.
-- Check every proposal asking to install, update, or uninstall a plugin with utmost care and review. Installation means granting an external contract permissions to do things on behalf of your DAO, so you want to be extra careful about:
- - the implementation contract
- - the setup contract
- - the helper contracts
- - the permissions being granted/revoked
-
-### DON'Ts ✋
-
-- Incapacitate your DAO by revoking all `EXECUTE_PERMISSION`. This means your DAO will be blocked and any assets you hold may be locked in forever. This can happen through:
- - uninstalling your last governance plugin.
- - applying an update to your last governance plugin.
-- Don't give permissions to directly call functions from the DAO. Better and safer to use a plugin instead.
-- If you're using the Token Voting plugin in your DAO, make sure you don't mint additional tokens without careful consideration. If you mint too many at once, this may lock your DAO, since you will not be able to reach the minimum participation threshold. This happens if there are not enough tokens already on the market to meet the minimum participation percentage and the DAO owns most of the governance tokens.
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/02-action-execution.md b/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/02-action-execution.md
deleted file mode 100644
index 4344ae907..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/02-action-execution.md
+++ /dev/null
@@ -1,150 +0,0 @@
----
-title: Executing actions on behalf of the DAO
----
-
-## Using the DAO Executor
-
-Executing actions on behalf of the DAO is done through the `execute` function from the `DAO.sol` contract. This function allows us to [pass an array of actions)](https://github.com/aragon/osx/blob/develop/packages/contracts/src/core/dao/DAO.sol) to be executed by the DAO contract itself.
-
-However, for the `execute` call to work, the address calling the function (the `msg.sender`) needs to have the [`EXECUTE_PERMISSION`](../../01-how-it-works/01-core/02-permissions/index.md#permissions-native-to-the-dao-contract). This is to prevent anyone from being able to execute actions on behalf of the DAO and keep your assets safe from malicious actors.
-
-## How to grant the Execute Permission
-
-Usually, the `EXECUTE_PERMISSION` is granted to a governance plugin of the DAO so that only the approved proposals can be executed. For example, we'd grant the `EXECUTE_PERMISSION` to the address of the Multisig Plugin. That way, when a proposal is approved by the required members of the multisig, the plugin is able to call the `execute` function on the DAO in order to get the actions executed.
-
-To grant the `EXECUTE_PERMISSION` to an address, you'll want to call on the `PermissionManager`'s [`grant` function](https://github.com/aragon/osx/blob/develop/packages/contracts/src/core/permission/PermissionManager.sol#L105) and pass it 4 parameters:
-
-- `where`: the address of the contract containing the function `who` wants access to
-- `who`: the address (EOA or contract) receiving the permission
-- `permissionId`: the permission identifier the caller needs to have in order to be able to execute the action
-- `condition`: the address of the condition contract that will be asked (if any) before authorizing the call to happen
-
-:::caution
-You probably don't want to grant `EXECUTE_PERMISSION` to any random address, since this gives the address access to execute any action on behalf of the DAO. We recommend you only grant `EXECUTE_PERMISSION` to governance plugins to ensure the safety of your assets. Granting `EXECUTE_PERMISSION` to an externally owned account is considered an anti-pattern.
-:::
-
-## Examples
-
-### Calling a DAO Function
-
-Imagine you want to call an internal function inside the `DAO` contract, for example, to manually [grant or revoke a permission](../../01-how-it-works/01-core/02-permissions/index.md). The corresponding `Action` and `execute` function call look as follows:
-
-```solidity
-function exampleGrantCall(
- DAO dao,
- bytes32 _callId,
- address _where,
- address _who,
- bytes32 _permissionId
-) {
- // Create the action array
- IDAO.Action[] memory actions = new IDAO.Action[](1);
- actions[0] = IDAO.Action({
- to: address(dao),
- value: 0,
- data: abi.encodeWithSelector(PermissionManager.grant.selector, _where, _who, _permissionId)
- });
-
- // Execute the action array
- (bytes[] memory execResults, ) = dao.execute({
- _callId: _callId,
- _actions: actions,
- _allowFailureMap: 0
- });
-}
-```
-
-Here we use the selector of the [`grant` function](../../03-reference-guide/core/permission/PermissionManager.md#external-function-grant). To revoke the permission, the selector of the [`revoke` function](../../03-reference-guide/core/permission/PermissionManager.md#external-function-revoke) must be used.
-
-If the caller possesses the [`ROOT_PERMISSION_ID` permission](../../01-how-it-works/01-core/02-permissions/index.md#permissions-native-to-the-dao-contract) on the DAO contract, the call becomes simpler and cheaper:
-
-:::caution
-Granting the `ROOT_PERMISSION_ID` permission to other contracts other than the `DAO` contract is dangerous and considered as an anti-pattern.
-:::
-
-```solidity
-function exampleGrantFunction(DAO dao, address _where, address _who, bytes32 _permissionId) {
- dao.grant(_where, _who, _permissionId); // For this to work, the `msg.sender` needs the `ROOT_PERMISSION_ID`
-}
-```
-
-### Sending Native Tokens
-
-Send `0.1 ETH` from the DAO treasury to Alice.
-The corresponding `Action` and `execute` function call would look as follows:
-
-```solidity
-function exampleNativeTokenTransfer(IDAO dao, bytes32 _callId, address _receiver) {
- // Create the action array
- IDAO.Action[] memory actions = new IDAO.Action[](1);
-
- actions[0] = IDAO.Action({to: _receiver, value: 0.1 ether, data: ''});
-
- // Execute the action array
- dao.execute({_callId: _callId, _actions: actions, _allowFailureMap: 0});
-}
-```
-
-### Calling a Function from an External Contract
-
-Imagine that you want to call an external function, let's say in a `Calculator` contract that adds two numbers for you. The corresponding `Action` and `execute` function call look as follows:
-
-```solidity
-contract ICalculator {
- function add(uint256 _a, uint256 _b) external pure returns (uint256 sum);
-}
-
-function exampleFunctionCall(
- IDAO dao,
- bytes32 _callId,
- ICalculator _calculator,
- uint256 _a,
- uint256 _b
-) {
- // Create the action array
- IDAO.Action[] memory actions = new IDAO.Action[](1);
- actions[0] = IDAO.Action({
- to: address(_calculator),
- value: 0, // 0 native tokens must be sent with this call
- data: abi.encodeCall(_calculator.add, (_a, _b))
- });
-
- // Execute the action array
- (bytes[] memory execResults, ) = dao.execute({
- _callId: _callId,
- _actions: actions,
- _allowFailureMap: 0
- });
-
- // Decode the action results
- uint256 sum = abi.decode(execResults[0], (uint256)); // the result of `add(_a,_b)`
-}
-```
-
-### Calling a Payable Function
-
-Wrap `0.1 ETH` from the DAO treasury into `wETH` by depositing it into the [Goerli WETH9 contract](https://goerli.etherscan.io/token/0xb4fbf271143f4fbf7b91a5ded31805e42b2208d6#writeContract).
-The corresponding `Action` and `execute` function call look as follows:
-
-```solidity
-interface IWETH9 {
- function deposit() external payable;
-
- function withdraw(uint256 _amount) external;
-}
-
-function examplePayableFunctionCall(IDAO dao, bytes32 _callId, IWETH9 _wethToken) {
- // Create the action array
-
- IDAO.Action[] memory actions = new IDAO.Action[](1);
-
- actions[0] = IDAO.Action({
- to: address(_wethToken),
- value: 0.1 ether,
- data: abi.encodeCall(IWETH9.deposit, ())
- });
-
- // Execute the action array
- dao.execute({_callId: _callId, _actions: actions, _allowFailureMap: 0});
-}
-```
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/03-protocol-upgrades.md b/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/03-protocol-upgrades.md
deleted file mode 100644
index ad874d1b0..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/03-protocol-upgrades.md
+++ /dev/null
@@ -1,13 +0,0 @@
----
-title: Upgrade your DAO to future Aragon OSx Versions
----
-
-## Upgrading to Future Aragon OSx Protocol Versions
-
-At Aragon, we are constantly working on improving the Aragon OSx framework.
-
-To make it easy for your DAO to switch to future Aragon OSx protocol versions, we added the possibility to upgrade our protocol in the future.
-
-Whenever we provide a new Aragon OSx protocol version, it will be possible to upgrade your DAO and associated plugins through your DAO dashboard.
-
-[Add your email here](https://aragondevelopers.substack.com/) if you'd like to receive updates when this happens!
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/04-managing-plugins/index.md b/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/04-managing-plugins/index.md
deleted file mode 100644
index 57f317043..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/04-managing-plugins/index.md
+++ /dev/null
@@ -1,50 +0,0 @@
----
-title: Manage your DAO's Plugins
----
-
-## How to manage the Plugins within your DAO
-
-
-
-You can install, uninstall or update any plugin into your DAO. If you want to dive deeper into plugins, check out [how plugins work here](../../../01-how-it-works/01-core/03-plugins/index.md).
-
-Before diving deeper into this guide, make sure that you understand [permissions](../../../01-how-it-works/01-core/02-permissions/index.md) and know about the [DAO executor](../../../01-how-it-works/01-core/01-dao/index.md).
-
-#### How to create a DAO with Plugins
-
-When you create your DAO, you must **install at least one functioning governance plugin** (meaning one plugin having the `EXECUTION_PERMISSION`) so your have a mechanism of executing actions on behalf of your DAO.
-This is crucial because otherwise nobody can operate the DAO and it would become incapacitated right after it was created. You would have spent gas for nothing.
-
-:::info
-If you create your DAO through the [Aragon App](https://app.aragon.org) or the [Aragon SDK](https://devs.aragon.org/docs/sdk), this will be checked and you will be warned in case you have not selected a suitable Aragon plugin.
-:::
-
-Although the easiest (and recommended) way to create your DAO is through the [Aragon App](https://app.aragon.org) or the [Aragon SDK](https://devs.aragon.org/docs/sdk), you can also do it directly from the protocol through calling on the [`createDAO` function](https://github.com/aragon/osx/blob/develop/packages/contracts/src/framework/dao/DAOFactory.sol#L63) from the `DAOFactory` contract and passing it the calldata `DAOSettings` for your DAO as well as the `PluginSettings` array referencing the plugins and the settings to be installed upon DAO creation.
-
-
-
-#### How to change a DAO's Governance Setup after a DAO has been created
-
-After a DAO is created with at least one plugin installed with `EXECUTE_PERMISSION` on the DAO, it's likely you may want to change change your governance setup later on by [installing, updating, or uninstalling plugins](../../../01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/index.md).
-
-Here, it is very important that you **maintain at least one functioning governance plugin** (a contract with `EXECUTE_PERMISSION` on the DAO) so that your assets are not locked in the future. In that regard, you want to be careful to not accidentally:
-
-- uninstall every plugin within your DAO, or
-- update or upgrade the plugin or otherwise change the internal plugin settings.
-
-If you do that, nobody would be able to create proposals and execute actions on the DAO anymore. Accordingly, DAOs must review proposals requesting to change the governance setup with utmost care before voting for them. In the next section, we explain how to review a proposal properly and what to pay attention too.
-
-
-
-### How to maintain Execution Permission on the DAO
-
-A very important thing to consider when operating your DAO is to make sure that you do not lock it - meaning, you allow it into a state where the DAO cannot execute actions anymore.
-
-The accidental loss of the permission to execute actions on your DAO ([the `EXECUTION_PERMISSION_ID` permission](../../../01-how-it-works/01-core/02-permissions/index.md#permissions-native-to-the-dao-contract)) incapacitates your DAO. If this happens, you are not able to withdraw funds or act through the DAO, unless you have the `ROOT_PERMISSION_ID` on the DAO.
-
-:::danger
-Do not interact directly with the smart contracts unless you know exactly what you are doing, **especially if this involves granting or revoking permissions**. Instead, use the Aragon App or Aragon SDK for creating and managing your DAO and interacting with the smart contracts.
-:::
-
-If you interact with the Aragon OSx protocol through the Aragon App frontend or the Aragon SDK and use only audited and verified plugins, this will not happen.
-However, diligence and care is required in some situations.
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/index.md b/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/index.md
deleted file mode 100644
index e50ec1097..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/01-dao/index.md
+++ /dev/null
@@ -1,27 +0,0 @@
----
-title: How to Operate a DAO
----
-
-DAOs are decentralized autonomous organizations. They are a group of people managing on-chain assets through a set of smart contracts.
-
-## What do you need to know in order to operate your DAO?
-
-DAOs manage assets through collective decision-making mechanisms. Although a lot can be said of the social, behavioral aspects of operating a DAO, in this guide we will focus on the technical aspects.
-
-In Aragon OSx, DAOs are a treasury and a permission management system - all other functionality is enabled through "capsules of opt-in functionality allowing the DAO to work in custom ways". These are called Plugins.
-
-:::note
-Plugins are smart contracts which extend the functionality of what the DAO can do. They are able to execute actions on behalf of the DAO through permissions the DAO grants or revokes them.
-:::
-
-Decision-making mechanisms are one example of Plugins. Treasury management, action bundles, or connections to other smart contracts are others.
-
-
-
-
-In this section, we'll go through how to operate and maintain your DAO:
-
-- [A Summary of Best Practices](./01-best-practices.md)
-- [How to execute actions on behalf of the DAO](./02-action-execution.md)
-- [How to manage a DAO's plugins](./04-managing-plugins/index.md)
-- [How to upgrade your DAO to a future Aragon OSx Releases](./03-protocol-upgrades.md)
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/01-best-practices.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/01-best-practices.md
deleted file mode 100644
index 7230dc4e1..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/01-best-practices.md
+++ /dev/null
@@ -1,30 +0,0 @@
----
-title: Before Starting
----
-
-## Advice for Developing a Plugin
-
-### DOs 👌
-
-- Document your contracts using [NatSpec](https://docs.soliditylang.org/en/v0.8.17/natspec-format.html).
-- Test your contracts, e.g., using toolkits such as [hardhat (JS)](https://hardhat.org/hardhat-runner/docs/guides/test-contracts) or [Foundry (Rust)](https://book.getfoundry.sh/forge/tests).
-- Use the `auth` modifier to control the access to functions in your plugin instead of `onlyOwner` or similar.
-- Write plugins implementations that need minimal permissions on the DAO.
-- Write `PluginSetup` contracts that remove all permissions on uninstallation that they requested during installation or updates.
-- Plan the lifecycle of your plugin (need for upgrades).
-- Follow our [versioning guidelines](../02-plugin-development/07-publication/01-versioning.md).
-
-### DON'Ts ✋
-
-- Leave any contract uninitialized.
-- Grant the `ROOT_PERMISSION_ID` permission to anything or anyone.
-- Grant with `who: ANY_ADDR` unless you know what you are doing.
-- Expect people to grant or revoke any permissions manually during the lifecycle of a plugin. The `PluginSetup` should take this complexity away from the user and after uninstallation, all permissions should be removed.
-- Write upgradeable contracts that:
- - Repurpose existing storage (in upgradeable plugins).
- - Inherit from previous versions as this can mess up the inheritance chain. Instead, write self-contained contracts.
-
-
-
-
-In the following sections, you will learn about the details about plugin development.
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/02-plugin-types.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/02-plugin-types.md
deleted file mode 100644
index eb2d31915..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/02-plugin-types.md
+++ /dev/null
@@ -1,73 +0,0 @@
----
-title: Choosing the Plugin Type
----
-
-## How to Choose the Base Contract for Your Plugin
-
-Although it is not mandatory to choose one of our interfaces as the base contracts for your plugins, we do offer some options for you to inherit from and speed up development.
-
-The needs of your plugin determine the type of plugin you may want to choose. This is based on:
-
-- the need for a plugin's upgradeability
-- whether you need it deployed by a specific deployment method
-- whether you need it to be compatible with meta transactions
-
-In this regard, we provide 3 options for base contracts you can choose from:
-
-- `Plugin` for instantiation via `new`
-- `PluginClones` for [minimal proxy pattern (ERC-1167)](https://eips.ethereum.org/EIPS/eip-1167) deployment
-- `PluginUUPSUpgradeable` for [UUPS pattern (ERC-1822)](https://eips.ethereum.org/EIPS/eip-1822) deployment
-
-Let's take a look at what this means for you.
-
-### Upgradeability & Deployment
-
-Upgradeability and the deployment method of a plugin contract go hand in hand. The motivation behind upgrading smart contracts is nicely summarized by OpenZeppelin:
-
-> Smart contracts in Ethereum are immutable by default. Once you create them there is no way to alter them, effectively acting as an unbreakable contract among participants.
->
-> However, for some scenarios, it is desirable to be able to modify them [...]
->
-> - to fix a bug [...],
-> - to add additional features, or simply to
-> - change the rules enforced by it.
->
-> Here’s what you’d need to do to fix a bug in a contract you cannot upgrade:
->
-> 1. Deploy a new version of the contract
-> 2. Manually migrate all state from the old one contract to the new one (which can be very expensive in terms of gas fees!)
-> 3. Update all contracts that interacted with the old contract to use the address of the new one
-> 4. Reach out to all your users and convince them to start using the new deployment (and handle both contracts being used simultaneously, as users are slow to migrate
->
-> _source: [OpenZeppelin: What's in an upgrade](https://docs.openzeppelin.com/learn/upgrading-smart-contracts#whats-in-an-upgrade)_
-
-Some key things to keep in mind:
-
-- With upgradeable smart contracts, you can modify their code while keep using or even extending the storage (see the guide [Writing Upgradeable Contracts](https://docs.openzeppelin.com/upgrades-plugins/1.x/writing-upgradeable) by OpenZeppelin).
-- To enable upgradeable smart contracts (as well as cheap contract clones), the proxy pattern is used.
-- Depending on your upgradeability requirements and the deployment method you choose, you can also greatly reduce the gas costs to distribute your plugin. However, the upgradeability and deployment method can introduce caveats during [the plugin setup](../../01-how-it-works/02-framework/02-plugin-management/02-plugin-setup/index.md), especially when updating from an older version to a new one.
-
-The following table compares the different deployment methods with their benefits and drawbacks:
-
-| | `new` Instantiation | Minimal Proxy (Clones) | Transparent Proxy | UUPS Proxy |
-| ------------- | --------------------------------------------- | ------------------------------------------------- | ------------------------------------------------ | --------------------------------------------- |
-| upgradability | no | no | yes | yes |
-| gas costs | high | very low | moderate | low |
-| difficulty | low | low | high | high |
-
-Accordingly, we recommend to use [minimal proxies (ERC-1167)](https://eips.ethereum.org/EIPS/eip-1167) for non-upgradeable and [UUPS proxies (1822)](https://eips.ethereum.org/EIPS/eip-1822) for upgradeable plugin.
-To help you with developing and deploying your plugin within the Aragon infrastructure, we provide the following implementation that you can inherit from:
-
-- `Plugin` for instantiation via `new`
-- `PluginClones` for [minimal proxy pattern (ERC-1167)](https://eips.ethereum.org/EIPS/eip-1167) deployment
-- `PluginUUPSUpgradeable` for [UUPS pattern (ERC-1822)](https://eips.ethereum.org/EIPS/eip-1822) deployment
-
-#### Caveats of Non-upgradeable Plugins
-
-Aragon plugins using the non-upgradeable smart contracts bases (`Plugin`, `PluginCloneable`) can be cheap to deploy (i.e., using clones) but **cannot be updated**.
-
-Updating, in distinction from upgrading, will call Aragon OSx' internal process for switching from an older plugin version to a newer one.
-
-:::caution
-To switch from an older version of a non-upgradeable contract to a newer one, the underlying contract has to be replaced. In consequence, the state of the older version is not available in the new version anymore, unless it is migrated or has been made publicly accessible in the old version through getter functions.
-:::
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/03-non-upgradeable-plugin/01-initialization.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/03-non-upgradeable-plugin/01-initialization.md
deleted file mode 100644
index ea62070a3..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/03-non-upgradeable-plugin/01-initialization.md
+++ /dev/null
@@ -1,81 +0,0 @@
----
-title: Initialization
----
-
-## How to Initialize Non-Upgradeable Plugins
-
-Every plugin should receive and store the address of the DAO it is associated with upon initialization. This is how the plugin will be able to interact with the DAO that has installed it.
-
-In addition, your plugin implementation might want to introduce other storage variables that should be initialized immediately after the contract was created. For example, in the `SimpleAdmin` plugin example (which sets one address as the full admin of the DAO), we'd want to store the `admin` address.
-
-```solidity
-contract SimpleAdmin is Plugin {
- address public admin;
-}
-```
-
-The way we set up the plugin's `initialize()` function depends on the plugin type selected. To review plugin types in depth, check out our [guide here](../02-plugin-types.md).
-
-Additionally, the way we deploy our contracts is directly correlated with how they're initialized. For Non-Upgradeable Plugins, there's two ways in which we can deploy our plugin:
-
-- Deployment via Solidity's `new` keyword, OR
-- Deployment via the Minimal Proxy Pattern
-
-### Option A: Deployment via Solidity's `new` Keyword
-
-To instantiate the contract via Solidity's `new` keyword, you should inherit from the `Plugin` Base Template Aragon created. You can find it [here](https://github.com/aragon/osx-commons/blob/develop/contracts/src/plugin/Plugin.sol).
-
-In this case, the compiler will force you to write a `constructor` function calling the `Plugin` parent `constructor` and provide it with a contract of type `IDAO`. Inside the constructor, you might want to initialize the storage variables that you have added yourself, such as the `admin` address in the example below.
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {Plugin, IDAO} from '@aragon/osx/core/plugin/Plugin.sol';
-
-contract SimpleAdmin is Plugin {
- address public immutable admin;
-
- /// @notice Initializes the contract.
- /// @param _dao The associated DAO.
- /// @param _admin The address of the admin.
- constructor(IDAO _dao, address _admin) Plugin(_dao) {
- admin = _admin;
- }
-}
-```
-
-:::note
-The `admin` variable is set as `immutable` so that it can never be changed. Immutable variables can only be initialized in the constructor.
-:::
-
-This type of constructor implementation stores the `IDAO _dao` reference in the right place. If your plugin is deployed often, which we could expect, we can [save significant amounts of gas by deployment through using the minimal proxy pattern](https://blog.openzeppelin.com/workshop-recap-cheap-contract-deployment-through-clones/).
-
-### Option B: Deployment via the Minimal Proxy Pattern
-
-To deploy our plugin via the [minimal clones pattern (ERC-1167)](https://eips.ethereum.org/EIPS/eip-1167), you inherit from the `PluginCloneable` contract introducing the same features as `Plugin`. The only difference is that you now have to remember to write an `initialize` function.
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {PluginCloneable, IDAO} from '@aragon/osx/core/plugin/PluginCloneable.sol';
-
-contract SimpleAdmin is PluginCloneable {
- address public admin;
-
- /// @notice Initializes the contract.
- /// @param _dao The associated DAO.
- /// @param _admin The address of the admin.
- function initialize(IDAO _dao, address _admin) external initializer {
- __PluginCloneable_init(_dao);
- admin = _admin;
- }
-}
-```
-
-We must protect it from being called multiple times by using [OpenZeppelin's `initializer` modifier made available through `Initalizable`](https://docs.openzeppelin.com/contracts/4.x/api/proxy#Initializable) and call the internal function `__PluginCloneable_init(IDAO _dao)` available through the `PluginCloneable` base contract to store the `IDAO _dao` reference in the right place.
-
-:::caution
-If you forget calling `__PluginCloneable_init(_dao)` inside your `initialize` function, your plugin won't be associated with a DAO and cannot use the DAO's `PermissionManager`.
-:::
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/03-non-upgradeable-plugin/02-implementation.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/03-non-upgradeable-plugin/02-implementation.md
deleted file mode 100644
index d0b8af771..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/03-non-upgradeable-plugin/02-implementation.md
+++ /dev/null
@@ -1,81 +0,0 @@
----
-title: Plugin Implementation Contract
----
-
-## How to Build a Non-Upgradeable Plugin
-
-Once we've initialized our plugin (take a look at our guide on [how to initialize Non-Upgradeable Plugins here](./01-initialization.md)), we can start using the Non-Upgradeable Base Template to perform actions on the DAO.
-
-### 1. Set the Permission Identifier
-
-Firstly, we want to define a [permission identifier](../../../01-how-it-works/01-core/02-permissions/index.md#permission-identifiers) `bytes32` constant at the top of the contract and establish a `keccak256` hash of the permission name we want to choose. In this example, we're calling it the `ADMIN_EXECUTE_PERMISSION`.
-
-```solidity
-contract SimpleAdmin is PluginCloneable {
- /// @notice The ID of the permission required to call the `execute` function.
- bytes32 public constant ADMIN_EXECUTE_PERMISSION_ID = keccak256('ADMIN_EXECUTE_PERMISSION');
-
- address public admin;
-
- /// @notice Initializes the contract.
- /// @param _dao The associated DAO.
- /// @param _admin The address of the admin.
- function initialize(IDAO _dao, address _admin) external initializer {
- __PluginCloneable_init(_dao);
- admin = _admin;
- }
-
- /// @notice Executes actions in the associated DAO.
- function execute(IDAO.Action[] calldata _actions) external auth(ADMIN_EXECUTE_PERMISSION_ID) {
- revert('Not implemented.');
- }
-}
-```
-
-:::note
-You are free to choose the permission name however you like. For example, you could also have used `keccak256('SIMPLE_ADMIN_PLUGIN:PERMISSION_1')`. However, it is important that the permission names are descriptive and cannot be confused with each other.
-:::
-
-Setting this permission is key because it ensures only signers who have been granted that permission are able to execute functions.
-
-### 2. Add the logic implementation
-
-Now that we have created the permission, we will use it to protect the implementation. We want to make sure only the authorized callers holding the `ADMIN_EXECUTE_PERMISSION`, can use the function.
-
-Because we have initialized the [`PluginClonable` base contract](https://github.com/aragon/osx-commons/blob/develop/contracts/src/plugin/PluginCloneable.sol), we can now use its features, i.e., the [`auth` modifier](https://github.com/aragon/osx-commons/blob/1cf46ff15dbda8481f9ee37558e7ea8b257d51f2/contracts/src/permission/auth/DaoAuthorizable.sol#L30-L35) provided through the `DaoAuthorizable` base class. The `auth('ADMIN_EXECUTE_PERMISSION')` returns an error if the address calling on the function has not been granted that permission, effectively protecting from malicious use cases.
-
-Later, we will also use the [`dao()` getter function from the base contract](https://github.com/aragon/osx-commons/blob/1cf46ff15dbda8481f9ee37558e7ea8b257d51f2/contracts/src/permission/auth/DaoAuthorizable.sol#L24-L28), which returns the associated DAO for that plugin.
-
-```solidity
-contract SimpleAdmin is PluginCloneable {
- /// @notice The ID of the permission required to call the `execute` function.
- bytes32 public constant ADMIN_EXECUTE_PERMISSION_ID = keccak256('ADMIN_EXECUTE_PERMISSION');
-
- address public admin;
-
- /// @notice Initializes the contract.
- /// @param _dao The associated DAO.
- /// @param _admin The address of the admin.
- function initialize(IDAO _dao, address _admin) external initializer {
- __PluginCloneable_init(_dao);
- admin = _admin;
- }
-
- /// @notice Executes actions in the associated DAO.
- /// @param _actions The actions to be executed by the DAO.
- function execute(IDAO.Action[] calldata _actions) external auth(ADMIN_EXECUTE_PERMISSION_ID) {
- dao().execute({callId: 0x0, actions: _actions, allowFailureMap: 0});
- }
-}
-```
-
-:::note
-In this example, we are building a governance plugin. To increase its capabilities and provide some standardization into the protocol, we recommend completing the governance plugin by [implementing the `IProposal` and `IMembership` interfaces](../05-governance-plugins/index.md).
-Optionally, you can also allow certain actions to fail by using [the failure map feature of the DAO executor](../../../01-how-it-works/01-core/01-dao/01-actions.md#allowing-for-failure).
-:::
-
-For now, we used default values for the `callId` and `allowFailureMap` parameters required by the DAO's `execute` function. With this, the plugin implementation could be used and deployed already. Feel free to add any additional logic to your plugin's capabilities here.
-
-### 3. Plugin done, Setup contract next!
-
-Now that we have the logic for the plugin implemented, we'll need to define how this plugin should be installed/uninstalled from a DAO. In the next step, we'll write the `PluginSetup` contract - the one containing the installation, uninstallation, and upgrading instructions for the plugin.
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/03-non-upgradeable-plugin/03-setup.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/03-non-upgradeable-plugin/03-setup.md
deleted file mode 100644
index 12e45a026..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/03-non-upgradeable-plugin/03-setup.md
+++ /dev/null
@@ -1,338 +0,0 @@
----
-title: Plugin Setup Contract
----
-
-## What is the Plugin Setup contract?
-
-The Plugin Setup contract is the contract defining the instructions for installing, uninstalling, or upgrading plugins into DAOs. This contract prepares the permission granting or revoking that needs to happen in order for plugins to be able to perform actions on behalf of the DAO.
-
-You need it for the plugin to be installed intto the DAO.
-
-### 1. Finish the Plugin contract
-
-Before building your Plugin Setup contract, make sure you have the logic for your plugin implemented. In this case, we're building a simple admin plugin which grants one address permission to execute actions on behalf of the DAO.
-
-```solidity
-contract SimpleAdmin is PluginCloneable {
- /// @notice The ID of the permission required to call the `execute` function.
- bytes32 public constant ADMIN_EXECUTE_PERMISSION_ID = keccak256('ADMIN_EXECUTE_PERMISSION');
-
- address public admin;
-
- /// @notice Initializes the contract.
- /// @param _dao The associated DAO.
- /// @param _admin The address of the admin.
- function initialize(IDAO _dao, address _admin) external initializer {
- __PluginCloneable_init(_dao);
- admin = _admin;
- }
-
- /// @notice Executes actions in the associated DAO.
- /// @param _actions The actions to be executed by the DAO.
- function execute(IDAO.Action[] calldata _actions) external auth(ADMIN_EXECUTE_PERMISSION_ID) {
- dao().execute({_callId: 0x0, _actions: _actions, _allowFailureMap: 0});
- }
-}
-```
-
-### 2. How to initialize the Plugin Setup contract
-
-Each `PluginSetup` contract is deployed only once and we will publish a separate `PluginSetup` instance for each version. Accordingly, we instantiate the `implementation` contract via Solidity's `new` keyword as deployment with the minimal proxy pattern would be more expensive in this case.
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {PluginSetup, IPluginSetup} from '@aragon/osx/framework/plugin/setup/PluginSetup.sol';
-import {SimpleAdmin} from './SimpleAdmin.sol';
-
-contract SimpleAdminSetup is PluginSetup {
- /// @notice The address of `SimpleAdmin` plugin contract to be cloned.
- address private immutable simpleAdminImplementation;
-
- /// @notice The constructor setting the `SimpleAdmin` implementation contract to clone from.
- constructor() {
- simpleAdminImplementation = address(new SimpleAdmin());
- }
-
- /// @inheritdoc IPluginSetup
- function implementation() external view returns (address) {
- return simpleAdminImplementation;
- }
-}
-```
-
-### 3. Build the Skeleton
-
-In order for the Plugin to be easily installed into the DAO, we need to define the permissions the plugin will need.
-
-We will create a `prepareInstallation()` function, as well as a `prepareUninstallation()` function. These are the functions the `PluginSetupProcessor.sol` (the contract in charge of installing plugins into the DAO) will use to prepare the installation/uninstallation of the plugin into the DAO.
-
-For example, a skeleton for our `SimpleAdminSetup` contract inheriting from `PluginSetup` looks as follows:
-
-```solidity
-import {PermissionLib} from '@aragon/osx/core/permission/PermissionLib.sol';
-
-contract SimpleAdminSetup is PluginSetup {
- /// @notice The address of `SimpleAdmin` plugin logic contract to be cloned.
- address private immutable simpleAdminImplementation;
-
- /// @notice The constructor setting the `SimpleAdmin` implementation contract to clone from.
- constructor() {
- simpleAdminImplementation = address(new SimpleAdmin());
- }
-
- /// @inheritdoc IPluginSetup
- function prepareInstallation(
- address _dao,
- bytes calldata _data
- ) external returns (address plugin, PreparedSetupData memory preparedSetupData) {
- revert('Not implemented yet.');
- }
-
- /// @inheritdoc IPluginSetup
- function prepareUninstallation(
- address _dao,
- SetupPayload calldata _payload
- ) external view returns (PermissionLib.MultiTargetPermission[] memory permissions) {
- revert('Not implemented yet.');
- }
-
- /// @inheritdoc IPluginSetup
- function implementation() external view returns (address) {
- return simpleAdminImplementation;
- }
-}
-```
-
-As you can see, we have a constructor storing the implementation contract instantiated via the `new` method in the private immutable variable `implementation` to save gas and a `implementation` function to retrieve it.
-
-Next, we will add the implementation for the `prepareInstallation` and `prepareUninstallation` functions.
-
-### 4. Implementing the `prepareInstallation()` function
-
-The `prepareInstallation()` function should take in two parameters:
-
-1. the `DAO` it prepares the installation for, and
-2. the `_data` parameter containing all the information needed for this function to work properly, in this case, the address we want to set as admin of our DAO.
-
-Hence, the first thing we should do when working on the `prepareInsallation()` function is decode the information from the `_data` parameter. We also want to check that the address is not accidentally set to `address(0)`, which would freeze the DAO forever.
-
-```solidity
-import {Clones} from '@openzeppelin/contracts/proxy/Clones.sol';
-
-contract SimpleAdminSetup is PluginSetup {
- using Clones for address;
-
- /// @notice Thrown if the admin address is zero.
- /// @param admin The admin address.
- error AdminAddressInvalid(address admin);
-
- // ...
-}
-```
-
-Then, we will use [OpenZeppelin's `Clones` library](https://docs.openzeppelin.com/contracts/4.x/api/proxy#Clones) to clone our Plugin contract and initialize it with the `admin` address. The first line, `using Clones for address;`, allows us to call OpenZeppelin `Clones` library to clone contracts deployed at an address.
-
-The second line introduces a custom error being thrown if the admin address specified is the zero address.
-
-```solidity
-function prepareInstallation(
- address _dao,
- bytes calldata _data
-) external returns (address plugin, PreparedSetupData memory preparedSetupData) {
- // Decode `_data` to extract the params needed for cloning and initializing the `Admin` plugin.
- address admin = abi.decode(_data, (address));
-
- if (admin == address(0)) {
- revert AdminAddressInvalid({admin: admin});
- }
-
- // Clone plugin contract.
- plugin = implementation.clone();
-
- // Initialize cloned plugin contract.
- SimpleAdmin(plugin).initialize(IDAO(_dao), admin);
-
- // Prepare permissions
- PermissionLib.MultiTargetPermission[]
- memory permissions = new PermissionLib.MultiTargetPermission[](2);
-
- // Grant the `ADMIN_EXECUTE_PERMISSION` of the plugin to the admin.
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Grant,
- where: plugin,
- who: admin,
- condition: PermissionLib.NO_CONDITION,
- permissionId: SimpleAdmin(plugin).ADMIN_EXECUTE_PERMISSION_ID()
- });
-
- // Grant the `EXECUTE_PERMISSION` on the DAO to the plugin.
- permissions[1] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Grant,
- where: _dao,
- who: plugin,
- condition: PermissionLib.NO_CONDITION,
- permissionId: DAO(payable(_dao)).EXECUTE_PERMISSION_ID()
- });
-
- preparedSetupData.permissions = permissions;
-}
-```
-
-Finally, we construct and return an array with the permissions that we need for our plugin to work properly.
-
-- First, we request granting the `ADMIN_EXECUTE_PERMISSION_ID` to the `admin` address received. This is what gives the address access to use `plugin`'s functionality - in this case, call on the plugin's `execute` function so it can execute actions on behalf of the DAO.
-- Second, we request that our newly deployed plugin can use the `EXECUTE_PERMISSION_ID` permission on the `_dao`. We don't add conditions to the permissions in this case, so we use the `NO_CONDITION` constant provided by `PermissionLib`.
-
-### 5. Implementing the `prepareUninstallation()` function
-
-For the uninstallation, we have to make sure to revoke the two permissions that have been granted during the installation process.
-First, we revoke the `ADMIN_EXECUTE_PERMISSION_ID` from the `admin` address that we have stored in the implementation contract.
-Second, we revoke the `EXECUTE_PERMISSION_ID` from the `plugin` address that we obtain from the `_payload` calldata.
-
-```solidity
-function prepareUninstallation(
- address _dao,
- SetupPayload calldata _payload
-) external view returns (PermissionLib.MultiTargetPermission[] memory permissions) {
- // Collect addresses
- address plugin = _payload.plugin;
- address admin = SimpleAdmin(plugin).admin();
-
- // Prepare permissions
- permissions = new PermissionLib.MultiTargetPermission[](2);
-
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Revoke,
- where: plugin,
- who: admin,
- condition: PermissionLib.NO_CONDITION,
- permissionId: SimpleAdmin(plugin).ADMIN_EXECUTE_PERMISSION_ID()
- });
-
- permissions[1] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Revoke,
- where: _dao,
- who: plugin,
- condition: PermissionLib.NO_CONDITION,
- permissionId: DAO(payable(_dao)).EXECUTE_PERMISSION_ID()
- });
-}
-```
-
-#### 6. Putting Everything Together
-
-Now, it's time to wrap up everything together. You should have a contract that looks like this:
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {Clones} from '@openzeppelin/contracts/proxy/Clones.sol';
-
-import {PermissionLib} from '@aragon/osx/core/permission/PermissionLib.sol';
-import {PluginSetup, IPluginSetup} from '@aragon/osx/framework/plugin/setup/PluginSetup.sol';
-import {SimpleAdmin} from './SimpleAdmin.sol';
-
-contract SimpleAdminSetup is PluginSetup {
- using Clones for address;
-
- /// @notice The address of `SimpleAdmin` plugin logic contract to be cloned.
- address private immutable simpleAdminImplementation;
-
- /// @notice Thrown if the admin address is zero.
- /// @param admin The admin address.
- error AdminAddressInvalid(address admin);
-
- /// @notice The constructor setting the `Admin` implementation contract to clone from.
- constructor() {
- simpleAdminImplementation = address(new SimpleAdmin());
- }
-
- /// @inheritdoc IPluginSetup
- function prepareInstallation(
- address _dao,
- bytes calldata _data
- ) external returns (address plugin, PreparedSetupData memory preparedSetupData) {
- // Decode `_data` to extract the params needed for cloning and initializing the `Admin` plugin.
- address admin = abi.decode(_data, (address));
-
- if (admin == address(0)) {
- revert AdminAddressInvalid({admin: admin});
- }
-
- // Clone plugin contract.
- plugin = implementation.clone();
-
- // Initialize cloned plugin contract.
- SimpleAdmin(plugin).initialize(IDAO(_dao), admin);
-
- // Prepare permissions
- PermissionLib.MultiTargetPermission[]
- memory permissions = new PermissionLib.MultiTargetPermission[](2);
-
- // Grant the `ADMIN_EXECUTE_PERMISSION` of the plugin to the admin.
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Grant,
- where: plugin,
- who: admin,
- condition: PermissionLib.NO_CONDITION,
- permissionId: SimpleAdmin(plugin).ADMIN_EXECUTE_PERMISSION_ID()
- });
-
- // Grant the `EXECUTE_PERMISSION` on the DAO to the plugin.
- permissions[1] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Grant,
- where: _dao,
- who: plugin,
- condition: PermissionLib.NO_CONDITION,
- permissionId: DAO(payable(_dao)).EXECUTE_PERMISSION_ID()
- });
-
- preparedSetupData.permissions = permissions;
- }
-
- /// @inheritdoc IPluginSetup
- function prepareUninstallation(
- address _dao,
- SetupPayload calldata _payload
- ) external view returns (PermissionLib.MultiTargetPermission[] memory permissions) {
- // Collect addresses
- address plugin = _payload.plugin;
- address admin = SimpleAdmin(plugin).admin();
-
- // Prepare permissions
- permissions = new PermissionLib.MultiTargetPermission[](2);
-
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Revoke,
- where: plugin,
- who: admin,
- condition: PermissionLib.NO_CONDITION,
- permissionId: SimpleAdmin(plugin).ADMIN_EXECUTE_PERMISSION_ID()
- });
-
- permissions[1] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Revoke,
- where: _dao,
- who: plugin,
- condition: PermissionLib.NO_CONDITION,
- permissionId: DAO(payable(_dao)).EXECUTE_PERMISSION_ID()
- });
- }
-
- /// @inheritdoc IPluginSetup
- function implementation() external view returns (address) {
- return simpleAdminImplementation;
- }
-}
-```
-
-Once done, our plugin is ready to be published on the Aragon plugin registry. With the address of the `SimpleAdminSetup` contract, we are ready for creating our `PluginRepo`, the plugin's repository where all plugin versions will live. Check out our how to guides on [publishing your plugin here](../07-publication/index.md).
-
-### In the future: Subsequent Builds
-
-For subsequent builds or releases of your plugin, you'll simply write a new implementation and associated Plugin Setup contract providing a new `prepareInstallation` and `prepareUninstallation` function.
-
-If a DAO wants to install the new build or release, it must uninstall its current plugin and freshly install the new plugin version, which can happen in the same action array in a governance proposal. However, the plugin storage and event history will be lost since this is a non-upgradeable plugin. If you want to prevent the latter, you can learn [how to write an upgradeable plugin here](../03-non-upgradeable-plugin/index.md).
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/03-non-upgradeable-plugin/index.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/03-non-upgradeable-plugin/index.md
deleted file mode 100644
index bf7da1984..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/03-non-upgradeable-plugin/index.md
+++ /dev/null
@@ -1,351 +0,0 @@
----
-title: Non-Upgradeable Plugins
----
-
-## Get Started with Non-Upgradeable Plugins
-
-A Non-Upgradeable Plugin is a Plugin built on smart contracts that cannot be upgraded. This may or may not be what you want.
-
-Some observations:
-
-- Non-Upgradeable contracts are simpler to create, deploy, and manage.
-- Instantiation is done via the `new` keyword or deployed via the [minimal proxy pattern (ERC-1167)](https://eips.ethereum.org/EIPS/eip-1167)
-- The storage is contained within each version. So if your plugin is dependent on state information from previous versions, you won't have access to it directly in upcoming versions, since every version is a blank new state. If this is a requirement for your project, we recommend you deploy an [Upgradeable Plugin](../04-upgradeable-plugin/index.md).
-
-Before moving on with the Guide, make sure you've read our documentation on [Choosing the Best Type for Your Plugin](../02-plugin-types.md) to make sure you're selecting the right type of contract for your Plugin.
-
-## Building a Non-Upgradeble Plugin
-
-We will build a plugin which returns "Hello world!" and the amount of times the function has been called.
-
-### 1. Setup
-
-1. Make sure you have Node.js in your computer.
-
-For Mac:
-
-```bash
-curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.1/install.sh | bash
-nvm install 18
-nvm use 18
-nvm alias default 18
-npm install npm --global
-```
-
-Or for Linux:
-
-```bash
-sudo apt update
-sudo apt install curl git
-curl -fsSL https://deb.nodesource.com/setup_18.x | sudo -E bash -
-sudo apt-get install -y nodejs
-```
-
-[Here's a tutorial](https://hardhat.org/tutorial/setting-up-the-environment) on installing this if you haven't done so already.
-
-2. Next up, we want to create a Hardhat project in our terminal. This is the Solidity framework we'll use to get our project up and runing.
-
-```bash
-npm init
-npm install --save-dev hardhat
-npx hardhat
-```
-
-[Here's a tutorial](https://hardhat.org/tutorial/creating-a-new-hardhat-project) on how to answer the prompts if you need. For reference, I used the Typescript option for my Hardhat project.
-
-3. Install `@aragon/osx` package
-
-We want to install the Aragon OSx contract package within our project so we can access and import them throughout our project. This should speed up development significantly, although not it's not mandatory in order to build Aragon plugins.
-
-```bash
-npm i @aragon/osx
-```
-
-### 2. Building the plugin
-
-1. Create `GreeterPlugin` contract
-
-Plugins are composed of two key contracts:
-
-- The `Plugin` contract, containing the implementation logic for the Plugin,
-- The `PluginSetup` contract, containing the instructions needed to install or uninstall a Plugin into a DAO.
-
-In this case, we will create the `GreeterPlugin.sol` contract containing the main logic for our plugin - aka returning "Hello world!" when calling on the `greet()` function. Keep in mind, that because we're importing from the `Plugin` base template in this case, we are able to tap into:
-
-- the `auth(PERMISSION_ID)` modifier, which checks whether the account calling on that function has the permission specified in the `auth` parameters.
-- the `dao()` getter function, which returns the DAO instance to which the plugin permissions are bound.
-
-First, in your terminal, create the `GreeterPlugin.sol` contract:
-
-```bash
-touch contracts/GreeterPlugin.sol
-```
-
-Then, inside of the file, add the functionality:
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {Plugin, IDAO} from '@aragon/osx/core/plugin/Plugin.sol';
-
-contract GreeterPlugin is Plugin {
- // Permissions are what connects everything together. Addresses who have been granted the GREET_PERMISSION will be able to call on functions with the modifier `auth(GREET_PERMISSION_ID)`. These will be granted in the PluginSetup.sol contract up next.
- bytes32 public constant GREET_PERMISSION_ID = keccak256('GREET_PERMISSION');
-
- uint256 public amountOfTimes = 0;
-
- constructor(IDAO _dao) Plugin(_dao) {}
-
- function greet() external auth(GREET_PERMISSION_ID) returns (string memory greeter) {
- greeter = string.concat(
- 'Hello world! This function has been called ',
- Strings.toString(amountOfTimes),
- ' times.'
- );
- amountOfTimes += 1;
- }
-
- function _amountOftimes() external view returns (uint256) {
- return amountOfTimes;
- }
-}
-```
-
-2. Create `GreeterPluginSetup` contract
-
-Now that we're done with our `GreeterPlugin` implementation, we can get started with the installation instructions.
-
-When we speak of installation, we're essentially referring to the the granting of the permissions needed so that the transactions can happen. In our `GreeterPlugin` contract, we defined a `GREET_PERMISSION`. Then, we used the `auth(GREET_PERMISSION_ID)` modifier on the `greet()`, defining that only those addresses with the `GREET_PERMISSION` will be able to call on the `greet()` function.
-
-In the `prepareInstallation()` function here then, we will grant the `GREET_PERMISSION` to the DAO so it can call the function. In the `prepareUninstallation()` function, we do the opposite and revoke the `GREET_PERMISSION`.
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {PluginSetup} from '@aragon/osx/framework/plugin/setup/PluginSetup.sol';
-import {PermissionLib} from '@aragon/osx/core/permission/PermissionLib.sol';
-import './GreeterPlugin.sol';
-
-contract GreeterPluginSetup is PluginSetup {
- function prepareInstallation(
- address _dao,
- bytes memory
- ) external returns (address plugin, PreparedSetupData memory preparedSetupData) {
- plugin = address(new GreeterPlugin(IDAO(_dao)));
-
- PermissionLib.MultiTargetPermission[]
- memory permissions = new PermissionLib.MultiTargetPermission[](1);
-
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Grant,
- where: plugin,
- who: _dao,
- condition: PermissionLib.NO_CONDITION,
- permissionId: keccak256('GREET_PERMISSION')
- });
-
- preparedSetupData.permissions = permissions;
- }
-
- function prepareUninstallation(
- address _dao,
- SetupPayload calldata _payload
- ) external pure returns (PermissionLib.MultiTargetPermission[] memory permissions) {
- permissions = new PermissionLib.MultiTargetPermission[](1);
-
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Revoke,
- where: _payload.plugin,
- who: _dao,
- condition: PermissionLib.NO_CONDITION,
- permissionId: keccak256('GREET_PERMISSION')
- });
- }
-
- function implementation() external view returns (address) {}
-}
-```
-
-### 3. Deploy your Plugin
-
-#### a) Hardhat's local environment
-
-1. In the Terminal, we first want to create a deployment script file:
-
-```bash
-touch scripts/deploy.ts
-```
-
-2. Now, let's add the deployment script in to the `deploy.ts` file
-
-```solidity
-import { ethers } from "hardhat";
-
-async function main() {
- const [deployer] = await ethers.getSigners();
-
- console.log("Deploying contracts with the account:", deployer.address);
- console.log("Account balance:", (await deployer.getBalance()).toString());
-
- const getGreeterSetup = await ethers.getContractFactory("GreeterPluginSetup");
- const GreeterSetup = await getGreeterSetup.deploy();
-
- await GreeterSetup.deployed();
-
- console.log("GreeterSetup address:", GreeterSetup.address);
-}
-
-// We recommend this pattern to be able to use async/await everywhere
-// and properly handle errors.
-main().catch((error) => {
- console.error(error);
- process.exitCode = 1;
-});
-```
-
-3. Let's deploy!
-
-In your terminal, run:
-
-```bash
-npx hardhat run scripts/deploy.ts
-```
-
-#### b) Goerli testnet network or others
-
-Now that we know the local deployment works, we will want to deploy our plugin to Goerli testnet so we can publish it in the Aragon OSx protocol to be accessed by DAOs.
-
-1. Firstly, let's set up the `hardhat.config.js` with Goerli environment attributes (or whichever network you'd like to deploy your plugin to).
-
-```tsx
-import '@nomicfoundation/hardhat-toolbox';
-
-// To find your Alchemy key, go to https://dashboard.alchemy.com/. Infura or any other provider would work here as well.
-const goerliAlchemyKey = 'add-your-own-alchemy-key';
-// To find a private key, go to your wallet of choice and export a private key. Remember this must be kept secret at all times.
-const privateKeyGoerli = 'add-your-account-private-key';
-
-module.exports = {
- defaultNetwork: 'hardhat',
- networks: {
- hardhat: {},
- goerli: {
- url: `https://eth-goerli.g.alchemy.com/v2/${goerliAlchemyKey}`,
- accounts: [privateKeyGoerli],
- },
- },
- solidity: {
- version: '0.8.17',
- settings: {
- optimizer: {
- enabled: true,
- runs: 200,
- },
- },
- },
- paths: {
- sources: './contracts',
- tests: './test',
- cache: './cache',
- artifacts: './artifacts',
- },
- mocha: {
- timeout: 40000,
- },
-};
-```
-
-2. Once we have the Goerli environment set up, run this command in your terminal to deploy the plugin:
-
-```bash
-npx hardhat run --network goerli scripts/deploy.ts
-```
-
-### 4. Publish the Plugin in Aragon OSx
-
-Now that the plugin is deployed on Goerli, we can publish it into the Aragon OSx Protocol so any DAO can install it!
-
-Publishing a plugin into Aragon OSx means creating a `PluginRepo` instance containing the plugin's first version. As developers can deploy more versions of the plugin moving forward, publishing a new version means adding a new `PluginSetup` contract into their plugin's `PluginRepo` contract. This is where all plugin versions will be stored and what Aragon's plugin installer will use to fetch the `latestVersion` and install it into DAO's.
-
-You can publish the plugin into Aragon's protocol through a few different ways:
-
-#### a) Etherscan
-
-Go to the [`PluginFactory`](https://goerli.etherscan.io/address/0x301868712b77744A3C0E5511609238399f0A2d4d#writeContract) contract on Etherscan and deploy the first version of your plugin.
-
-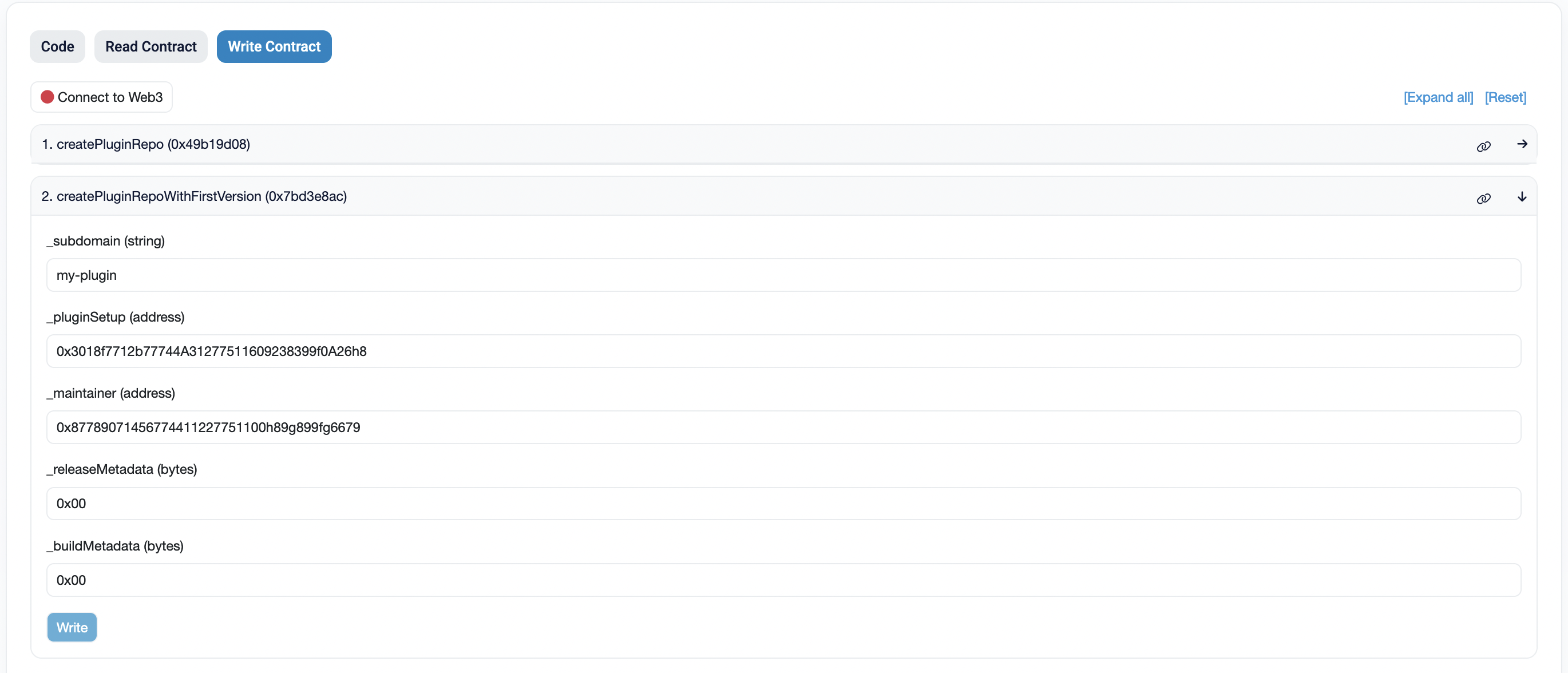
-
-#### b) Publishing script
-
-You can also publish your Plugin through using a `publish` script.
-
-1. Create the `publish.ts` file within your `scripts` folder.
-
-```bash
-touch scripts/publish.ts
-```
-
-2. Add this publishing script to the `publish.ts` file.
-
-This will get the `PluginRepoFactory` contract and call on its `createPluginRepoWithFirstVersion` to create the plugin's `PluginRepo` instance, where plugin versions will be stored.
-
-```ts
-import {
- PluginRepoFactory__factory,
- PluginRepoRegistry__factory,
- PluginRepo__factory,
-} from '@aragon/osx-ethers';
-import {DeployFunction} from 'hardhat-deploy/types';
-import {HardhatRuntimeEnvironment} from 'hardhat/types';
-
-const func: DeployFunction = async function (hre: HardhatRuntimeEnvironment) {
- const {deployments, network} = hre;
- const [deployer] = await hre.ethers.getSigners();
-
- const pluginRepoFactoryAddr = '0x301868712b77744A3C0E5511609238399f0A2d4d';
-
- const pluginRepoFactory = PluginRepoFactory__factory.connect(pluginRepoFactoryAddr, deployer);
-
- const pluginName = 'greeter-plugin';
- const pluginSetupContractName = 'GreeterPluginSetup';
-
- const pluginSetupContract = await deployments.get(pluginSetupContractName);
-
- const tx = await pluginRepoFactory.createPluginRepoWithFirstVersion(
- pluginName,
- pluginSetupContract.address,
- deployer.address,
- '0x00', // releaseMetadata: the hex representation of the CID containing your plugin's metadata - so the description, name, author, any UI, etc
- '0x00' // buildMetadata: same as above but for each build, rather than release
- );
-
- console.log(
- `You can find the transaction address which published the ${pluginName} Plugin here: ${tx}`
- );
-};
-
-export default func;
-```
-
-In order to run the script and finalize the publishing, run this in your terminal:
-
-```bash
-npx hardhat run scripts/publish.ts
-```
-
-To publish new versions in the future,
-
-## Conclusion
-
-Hope this tutorial is useful to get you started developing for Aragon! If you need any additional support or questions, feel free to hop into our [Discord](https://discord.com/channels/672466989217873929/742442842474938478) and ask away.
-
-Excited to see what you build! 🔥
-
-Up next, check out our guides on:
-
-1. [How to initialize Non-Upgradeable Plugins](./01-initialization.md)
-2. [How to build the implementation of a Non-Upgradeable Plugin](./02-implementation.md)
-3. [How to build and deploy a Plugin Setup contract for a Non-Upgradeable Plugin](./03-setup.md)
-4. [How to publish my plugin into the Aragon OSx protocol](../07-publication/index.md)
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/01-initialization.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/01-initialization.md
deleted file mode 100644
index c843ec27c..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/01-initialization.md
+++ /dev/null
@@ -1,76 +0,0 @@
----
-title: Intialization
----
-
-## How to Initialize Upgradeable Plugins
-
-To deploy your implementation contract via the [UUPS pattern (ERC-1822)](https://eips.ethereum.org/EIPS/eip-1822), you inherit from the `PluginUUPSUpgradeable` contract.
-
-We must protect it from being set up multiple times by using [OpenZeppelin's `initializer` modifier made available through `Initalizable`](https://docs.openzeppelin.com/contracts/4.x/api/proxy#Initializable). In order to do this, we will call the internal function `__PluginUUPSUpgradeable_init(IDAO _dao)` function available through the `PluginUUPSUpgradeable` base contract to store the `IDAO _dao` reference in the right place.
-
-:::note
-This has to be called - otherwise, anyone else could call the plugin's initialization with whatever params they wanted.
-:::
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {PluginUUPSUpgradeable, IDAO} '@aragon/osx/core/plugin/PluginUUPSUpgradeable.sol';
-
-/// @title SimpleStorage build 1
-contract SimpleStorageBuild1 is PluginUUPSUpgradeable {
- uint256 public number; // added in build 1
-
- /// @notice Initializes the plugin when build 1 is installed.
- function initializeBuild1(IDAO _dao, uint256 _number) external initializer {
- __PluginUUPSUpgradeable_init(_dao);
- number = _number;
- }
-}
-```
-
-:::note
-Keep in mind that in order to discriminate between the different initialize functions of your different builds, we name the initialize function `initializeBuild1`. This becomes more demanding for subsequent builds of your plugin.
-:::
-
-### Initializing Subsequent Builds
-
-Since you have chosen to build an upgradeable plugin, you can publish subsequent builds of plugin and **allow the users to update from an earlier build without losing the storage**.
-
-:::caution
-Do not inherit from previous versions as this can mess up the inheritance chain. Instead, write self-contained contracts by simply copying the code or modifying the file in your git repo.
-:::
-
-In this example, we wrote a `SimpleStorageBuild2` contract and added a new storage variable `address public account;`. Because users can freshly install the new version or update from build 1, we now have to write two initializer functions: `initializeBuild2` and `initializeFromBuild1` in our Plugin implementation contract.
-
-```solidity
-/// @title SimpleStorage build 2
-contract SimpleStorageBuild2 is PluginUUPSUpgradeable {
- uint256 public number; // added in build 1
- address public account; // added in build 2
-
- /// @notice Initializes the plugin when build 2 is installed.
- function initializeBuild2(
- IDAO _dao,
- uint256 _number,
- address _account
- ) external reinitializer(2) {
- __PluginUUPSUpgradeable_init(_dao);
- number = _number;
- account = _account;
- }
-
- /// @notice Initializes the plugin when the update from build 1 to build 2 is applied.
- /// @dev The initialization of `SimpleStorageBuild1` has already happened.
- function initializeFromBuild1(IDAO _dao, address _account) external reinitializer(2) {
- account = _account;
- }
-}
-```
-
-In general, for each version for which you want to support updates from, you have to provide a separate `initializeFromBuildX` function taking care of initializing the storage and transferring the `helpers` and `permissions` of the previous version into the same state as if it had been freshly installed.
-
-Each `initializeBuildX` must be protected with a modifier that allows it to be only called once.
-
-In contrast to build 1, we now must use [OpenZeppelin's `modifier reinitializer(uint8 build)`](https://docs.openzeppelin.com/contracts/4.x/api/proxy#Initializable-reinitializer-uint8-) for build 2 instead of `modifier initializer` because it allows us to execute 255 subsequent initializations. More specifically, we used `reinitializer(2)` here for our build 2. Note that we could also have used `function initializeBuild1(IDAO _dao, uint256 _number) external reinitializer(1)` for build 1 because `initializer` and `reinitializer(1)` are equivalent statements. For build 3, we must use `reinitializer(3)`, for build 4 `reinitializer(4)` and so on.
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/02-implementation.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/02-implementation.md
deleted file mode 100644
index fa53b80f4..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/02-implementation.md
+++ /dev/null
@@ -1,44 +0,0 @@
----
-title: Plugin Implementation Contract
----
-
-## How to build an Upgradeable Plugin implementation contract
-
-In this guide, we'll build a `SimpleStorage` Upgradeable plugin which all it does is storing a number.
-
-The Plugin contract is the one containing all the logic we'd like to implement on the DAO.
-
-### 1. Set up the initialize function
-
-Make sure you have the initializer of your plugin well set up. Please review [our guide on how to do that here](./01-initialization.md) if you haven't already.
-
-Once you this is done, let's dive into several implementations and builds, as can be expected for Upgradeable plugins.
-
-### 2. Adding your plugin implementation logic
-
-In our first build, we want to add an authorized `storeNumber` function to the contract - allowing a caller holding the `STORE_PERMISSION_ID` permission to change the stored value similar to what we did for [the non-upgradeable `SimpleAdmin` Plugin](../03-non-upgradeable-plugin/02-implementation.md):
-
-```solidity
-import {PluginUUPSUpgradeable, IDAO} '@aragon/osx/core/plugin/PluginUUPSUpgradeable.sol';
-
-/// @title SimpleStorage build 1
-contract SimpleStorageBuild1 is PluginUUPSUpgradeable {
- bytes32 public constant STORE_PERMISSION_ID = keccak256('STORE_PERMISSION');
-
- uint256 public number; // added in build 1
-
- /// @notice Initializes the plugin when build 1 is installed.
- function initializeBuild1(IDAO _dao, uint256 _number) external initializer {
- __PluginUUPSUpgradeable_init(_dao);
- number = _number;
- }
-
- function storeNumber(uint256 _number) external auth(STORE_PERMISSION_ID) {
- number = _number;
- }
-}
-```
-
-### 3. Plugin done, PluginSetup contract next!
-
-Now that we have the logic for the plugin implemented, we'll need to define how this plugin should be installed/uninstalled from a DAO. In the next step, we'll write the `PluginSetup` contract - the one containing the installation, uninstallation, and upgrading instructions for the plugin.
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/03-setup.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/03-setup.md
deleted file mode 100644
index 4f3fc186e..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/03-setup.md
+++ /dev/null
@@ -1,204 +0,0 @@
----
-title: Plugin Setup Contract
----
-
-## How to build the Plugin Setup Contract for Upgradeable Plugins
-
-The Plugin Setup contract is the contract defining the instructions for installing, uninstalling, or upgrading plugins into DAOs. This contract prepares the permission granting or revoking that needs to happen in order for plugins to be able to perform actions on behalf of the DAO.
-
-### 1. Finish the Plugin contract's first build
-
-Before building the Plugin Setup contract, make sure you have the logic for your plugin implemented. In this case, we're building a simple storage plugin which stores a number.
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {IDAO, PluginUUPSUpgradeable} from '@aragon/osx/core/plugin/PluginUUPSUpgradeable.sol';
-
-/// @title SimpleStorage build 1
-contract SimpleStorageBuild1 is PluginUUPSUpgradeable {
- bytes32 public constant STORE_PERMISSION_ID = keccak256('STORE_PERMISSION');
-
- uint256 public number; // added in build 1
-
- /// @notice Initializes the plugin when build 1 is installed.
- function initializeBuild1(IDAO _dao, uint256 _number) external initializer {
- __PluginUUPSUpgradeable_init(_dao);
- number = _number;
- }
-
- function storeNumber(uint256 _number) external auth(STORE_PERMISSION_ID) {
- number = _number;
- }
-}
-```
-
-### 2. Add the `prepareInstallation()` and `prepareUninstallation()` functions
-
-Each `PluginSetup` contract is deployed only once and each plugin version will have its own `PluginSetup` contract deployed. Accordingly, we instantiate the `implementation` contract via Solidity's `new` keyword as deployment with the minimal proxy pattern would be more expensive in this case.
-
-In order for the Plugin to be easily installed into the DAO, we need to define the instructions for the plugin to work effectively. We have to tell the DAO's Permission Manager which permissions it needs to grant or revoke.
-
-Hence, we will create a `prepareInstallation()` function, as well as a `prepareUninstallation()` function. These are the functions the `PluginSetupProcessor.sol` (the contract in charge of installing plugins into the DAO) will use.
-
-The `prepareInstallation()` function takes in two parameters:
-
-1. the `DAO` it should prepare the installation for, and
-2. the `_data` parameter containing all the information needed for this function to work properly, encoded as a `bytes memory`. In this case, we get the number we want to store.
-
-Hence, the first thing we should do when working on the `prepareInsallation()` function is decode the information from the `_data` parameter.
-Similarly, the `prepareUninstallation()` function takes in a `payload`.
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-
-pragma solidity 0.8.21;
-
-import {PermissionLib} from '@aragon/osx/core/permission/PermissionLib.sol';
-import {PluginSetup, IPluginSetup} from '@aragon/osx/framework/plugin/setup/PluginSetup.sol';
-import {SimpleStorageBuild1} from './SimpleStorageBuild1.sol';
-
-/// @title SimpleStorageSetup build 1
-contract SimpleStorageBuild1Setup is PluginSetup {
- address private immutable simpleStorageImplementation;
-
- constructor() {
- simpleStorageImplementation = address(new SimpleStorageBuild1());
- }
-
- /// @inheritdoc IPluginSetup
- function prepareInstallation(
- address _dao,
- bytes memory _data
- ) external returns (address plugin, PreparedSetupData memory preparedSetupData) {
- uint256 number = abi.decode(_data, (uint256));
-
- plugin = createERC1967Proxy(
- simpleStorageImplementation,
- abi.encodeCall(SimpleStorageBuild1.initializeBuild1, (IDAO(_dao), number))
- );
-
- PermissionLib.MultiTargetPermission[]
- memory permissions = new PermissionLib.MultiTargetPermission[](1);
-
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Grant,
- where: plugin,
- who: _dao,
- condition: PermissionLib.NO_CONDITION,
- permissionId: SimpleStorageBuild1(this.implementation()).STORE_PERMISSION_ID()
- });
-
- preparedSetupData.permissions = permissions;
- }
-
- /// @inheritdoc IPluginSetup
- function prepareUninstallation(
- address _dao,
- SetupPayload calldata _payload
- ) external view returns (PermissionLib.MultiTargetPermission[] memory permissions) {
- permissions = new PermissionLib.MultiTargetPermission[](1);
-
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Revoke,
- where: _payload.plugin,
- who: _dao,
- condition: PermissionLib.NO_CONDITION,
- permissionId: SimpleStorageBuild1(this.implementation()).STORE_PERMISSION_ID()
- });
- }
-
- /// @inheritdoc IPluginSetup
- function implementation() external view returns (address) {
- return simpleStorageImplementation;
- }
-}
-```
-
-As you can see, we have a constructor storing the implementation contract instantiated via the `new` method in the private immutable variable `implementation` to save gas and an `implementation` function to return it.
-
-:::note
-Specifically important for this type of plugin is the `prepareUpdate()` function. Since we don't know the parameters we will require when updating the plugin to the next version, we can't add the `prepareUpdate()` function just yet. However, keep in mind that we will need to deploy new Plugin Setup contracts in subsequent builds to add in the `prepareUpdate()` function with each build requirements. We see this in depth in the ["How to update an Upgradeable Plugin" section](./05-updating-versions.md).
-:::
-
-### 3. Deployment
-
-Once you're done with your Plugin Setup contract, we'll need to deploy it so we can publish it into the Aragon OSx protocol. You can deploy your contract with a basic deployment script.
-
-Firstly, we'll make sure our preferred network is well setup within our `hardhat.config.js` file, which should look something like:
-
-```js
-import '@nomicfoundation/hardhat-toolbox';
-
-// To find your Alchemy key, go to https://dashboard.alchemy.com/. Infure or any other provider would work here as well.
-const goerliAlchemyKey = 'add-your-own-alchemy-key';
-// To find a private key, go to your wallet of choice and export a private key. Remember this must be kept secret at all times.
-const privateKeyGoerli = 'add-your-account-private-key';
-
-module.exports = {
- defaultNetwork: 'hardhat',
- networks: {
- hardhat: {},
- goerli: {
- url: `https://eth-goerli.g.alchemy.com/v2/${goerliAlchemyKey}`,
- accounts: [privateKeyGoerli],
- },
- },
- solidity: {
- version: '0.8.17',
- settings: {
- optimizer: {
- enabled: true,
- runs: 200,
- },
- },
- },
- paths: {
- sources: './contracts',
- tests: './test',
- cache: './cache',
- artifacts: './artifacts',
- },
- mocha: {
- timeout: 40000,
- },
-};
-```
-
-Then, create a `scripts/deploy.js` file and add a simple deploy script. We'll only be deploying the PluginSetup contract, since this should deploy the Plugin contract within its constructor.
-
-```js
-import {ethers} from 'hardhat';
-
-async function main() {
- const [deployer] = await ethers.getSigners();
-
- console.log('Deploying contracts with the account:', deployer.address);
- console.log('Account balance:', (await deployer.getBalance()).toString());
-
- const getSimpleStorageSetup = await ethers.getContractFactory('SimpleStorageSetup');
- const SimpleStorageSetup = await SimpleStorageSetup.deploy();
-
- await SimpleStorageSetup.deployed();
-
- console.log('SimpleStorageSetup address:', SimpleStorageSetup.address);
-}
-
-// We recommend this pattern to be able to use async/await everywhere
-// and properly handle errors.
-main().catch(error => {
- console.error(error);
- process.exitCode = 1;
-});
-```
-
-Finally, run this in your terminal to execute the command:
-
-```bash
-npx hardhat run scripts/deploy.ts
-```
-
-### 4. Publishing the Plugin to the Aragon OSx Protocol
-
-Once done, our plugin is ready to be published on the Aragon plugin registry. With the address of the `SimpleAdminSetup` contract deployed, we're almost ready for creating our `PluginRepo`, the plugin's repository where all plugin versions will live. Check out our how to guides on [publishing your plugin here](../07-publication/index.md).
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/04-subsequent-builds.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/04-subsequent-builds.md
deleted file mode 100644
index bd2d9bcd8..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/04-subsequent-builds.md
+++ /dev/null
@@ -1,150 +0,0 @@
----
-title: Subsequent Builds
----
-
-## How to create a subsequent build to an Upgradeable Plugin
-
-A build is a new implementation of your Upgradeable Plugin. Upgradeable contracts offer advantages because you can cheaply change or fix the logic of your contract without losing the storage of your contract.
-
-The Aragon OSx protocol has an on-chain versioning system built-in, which distinguishes between releases and builds.
-
-- **Releases** contain breaking changes, which are incompatible with preexisting installations. Updates to a different release are not possible. Instead, you must install the new plugin release and uninstall the old one.
-- **Builds** are minor/patch versions within a release, and they are meant for compatible upgrades only (adding a feature or fixing a bug without changing anything else).
-
-In this how to guide, we'll go through how we can create these builds for our plugins. Specifically, we'll showcase two specific types of builds - one that modifies the storage of the plugins, another one which modifies its bytecode. Both are possible and can be implemented within the same build implementation as well.
-
-### 1. Make sure your previous build is deployed and published
-
-Make sure you have at least one build already deployed and published into the Aragon protocol. Make sure to check out our [publishing guide](../07-publication/index.md) to ensure this step is done.
-
-### 2. Create a new build implementation
-
-In this second build implementation we want to update the functionality of our plugin - in this case, we want to update the storage of our plugin with new values. Specifically, we will add a second storage variable `address public account;`. Additional to the `initializeFromBuild2` function, we also want to add a second setter function `storeAccount` that uses the same permission as `storeNumber`.
-
-As you can see, we're still inheritting from the `PluginUUPSUpgradeable` contract and simply overriding some implementation from the previous build. The idea is that when someone upgrades the plugin and calls on these functions, they'll use this new upgraded implementation, rather than the older one.
-
-```solidity
-import {PluginUUPSUpgradeable, IDAO} '@aragon/osx/core/plugin/PluginUUPSUpgradeable.sol';
-
-/// @title SimpleStorage build 2
-contract SimpleStorageBuild2 is PluginUUPSUpgradeable {
- bytes32 public constant STORE_PERMISSION_ID = keccak256('STORE_PERMISSION');
-
- uint256 public number; // added in build 1
- address public account; // added in build 2
-
- /// @notice Initializes the plugin when build 2 is installed.
- function initializeBuild2(
- IDAO _dao,
- uint256 _number,
- address _account
- ) external reinitializer(2) {
- __PluginUUPSUpgradeable_init(_dao);
- number = _number;
- account = _account;
- }
-
- /// @notice Initializes the plugin when the update from build 1 to build 2 is applied.
- /// @dev The initialization of `SimpleStorageBuild1` has already happened.
- function initializeFromBuild1(address _account) external reinitializer(2) {
- account = _account;
- }
-
- function storeNumber(uint256 _number) external auth(STORE_PERMISSION_ID) {
- number = _number;
- }
-
- function storeAccount(address _account) external auth(STORE_PERMISSION_ID) {
- account = _account;
- }
-}
-```
-
-Builds that you publish don't necessarily need to introduce new storage varaibles of your contracts and don't necessarily need to change the storage. To read more about Upgradeability, check out [OpenZeppelin's UUPSUpgradeability implementation here](https://docs.openzeppelin.com/contracts/4.x/api/proxy#UUPSUpgradeable).
-
-:::note
-Note that because these contracts are Upgradeable, keeping storage gaps `uint256 [50] __gap;` in dependencies is a must in order to avoid storage corruption. To learn more about storage gaps, review OpenZeppelin's documentation [here](https://docs.openzeppelin.com/upgrades-plugins/1.x/writing-upgradeable#storage-gaps).
-:::
-
-### 3. Alternatively, a build implementation modifying bytecode
-
-Updates for your contracts don't necessarily need to affect the storage, they can also modify the plugin's bytecode. Modifying the contract's bytecode, means making changes to:
-
-- functions
-- constants
-- immutables
-- events
-- errors
-
-For this third build, then, we want to change the bytecode of our implementation as an example, so we 've introduced two separate permissions for the `storeNumber` and `storeAccount` functions and named them `STORE_NUMBER_PERMISSION_ID` and `STORE_ACCOUNT_PERMISSION_ID` permission, respectively. Additionally, we decided to add the `NumberStored` and `AccountStored` events as well as an error preventing users from setting the same value twice. All these changes only affect the contract bytecode and not the storage.
-
-Here, it is important to remember how Solidity stores `constant`s (and `immutable`s). In contrast to normal variables, they are directly written into the bytecode on contract creation so that we don't need to worry that the second `bytes32` constant that we added shifts down the storage so that the value in `uint256 public number` gets lost.
-It is also important to note that, the `initializeFromBuild2` could be left empty. Here, we just emit the events with the currently stored values.
-
-```solidity
-import {PluginUUPSUpgradeable, IDAO} '@aragon/osx/core/plugin/PluginUUPSUpgradeable.sol';
-
-/// @title SimpleStorage build 3
-contract SimpleStorageBuild3 is PluginUUPSUpgradeable {
- bytes32 public constant STORE_NUMBER_PERMISSION_ID = keccak256('STORE_NUMBER_PERMISSION'); // changed in build 3
- bytes32 public constant STORE_ACCOUNT_PERMISSION_ID = keccak256('STORE_ACCOUNT_PERMISSION'); // added in build 3
-
- uint256 public number; // added in build 1
- address public account; // added in build 2
-
- // added in build 3
- event NumberStored(uint256 number);
- event AccountStored(address number);
- error AlreadyStored();
-
- /// @notice Initializes the plugin when build 3 is installed.
- function initializeBuild3(
- IDAO _dao,
- uint256 _number,
- address _account
- ) external reinitializer(3) {
- __PluginUUPSUpgradeable_init(_dao);
- number = _number;
- account = _account;
-
- emit NumberStored({number: _number});
- emit AccountStored({account: _account});
- }
-
- /// @notice Initializes the plugin when the update from build 2 to build 3 is applied.
- /// @dev The initialization of `SimpleStorageBuild2` has already happened.
- function initializeFromBuild2() external reinitializer(3) {
- emit NumberStored({number: number});
- emit AccountStored({account: account});
- }
-
- /// @notice Initializes the plugin when the update from build 1 to build 3 is applied.
- /// @dev The initialization of `SimpleStorageBuild1` has already happened.
- function initializeFromBuild1(address _account) external reinitializer(3) {
- account = _account;
-
- emit NumberStored({number: number});
- emit AccountStored({account: _account});
- }
-
- function storeNumber(uint256 _number) external auth(STORE_NUMBER_PERMISSION_ID) {
- if (_number == number) revert AlreadyStored();
-
- number = _number;
-
- emit NumberStored({number: _number});
- }
-
- function storeAccount(address _account) external auth(STORE_ACCOUNT_PERMISSION_ID) {
- if (_account == account) revert AlreadyStored();
-
- account = _account;
-
- emit AccountStored({account: _account});
- }
-}
-```
-
-:::note
-Despite no storage-related changes happening in build 3, we must apply the `reinitializer(3)` modifier to all `initialize` functions so that none of them can be called twice or in the wrong order.
-:::
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/05-updating-versions.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/05-updating-versions.md
deleted file mode 100644
index e10e3b44b..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/05-updating-versions.md
+++ /dev/null
@@ -1,360 +0,0 @@
----
-title: Upgrade a DAO Plugin
----
-
-## How to upgrade an Upgradeable Plugin
-
-Updating an Upgradeable plugin means we want to direct the implementation of our functionality to a new build, rather than the existing one.
-
-In this tutorial, we will go through how to update the version of an Upgradeable plugin and each component needed.
-
-### 1. Create the new build implementation contract
-
-Firstly, you want to create the new build implementation contract the plugin should use. You can read more about how to do this in the ["How to create a subsequent build implementation to an Upgradeable Plugin" guide](./04-subsequent-builds.md).
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {IDAO, PluginUUPSUpgradeable} from '@aragon/osx/core/plugin/PluginUUPSUpgradeable.sol';
-
-/// @title SimpleStorage build 2
-contract SimpleStorageBuild2 is PluginUUPSUpgradeable {
- bytes32 public constant STORE_PERMISSION_ID = keccak256('STORE_PERMISSION');
-
- uint256 public number; // added in build 1
- address public account; // added in build 2
-
- /// @notice Initializes the plugin when build 2 is installed.
- function initializeBuild2(
- IDAO _dao,
- uint256 _number,
- address _account
- ) external reinitializer(2) {
- __PluginUUPSUpgradeable_init(_dao);
- number = _number;
- account = _account;
- }
-
- /// @notice Initializes the plugin when the update from build 1 to build 2 is applied.
- /// @dev The initialization of `SimpleStorageBuild1` has already happened.
- function initializeFromBuild1(address _account) external reinitializer(2) {
- account = _account;
- }
-
- function storeNumber(uint256 _number) external auth(STORE_PERMISSION_ID) {
- number = _number;
- }
-
- function storeAccount(address _account) external auth(STORE_PERMISSION_ID) {
- account = _account;
- }
-}
-```
-
-### 2. Write a new Plugin Setup contract
-
-In order to do update a plugin, we need a `prepareUpdate()` function in our Plugin Setup contract which points the functionality to a new build, as we described in the ["How to create a subsequent build implementation to an Upgradeable Plugin" guide](./04-subsequent-builds.md). The `prepareUpdate()` function must transition the plugin from the old build state into the new one so that it ends up having the same permissions (and helpers) as if it had been freshly installed.
-
-In contrast to the original build 1, build 2 requires two input arguments: `uint256 _number` and `address _account` that we decode from the bytes-encoded input `_data`.
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-
-pragma solidity 0.8.21;
-
-import {PermissionLib} from '@aragon/osx/core/permission/PermissionLib.sol';
-import {PluginSetup, IPluginSetup} from '@aragon/osx/framework/plugin/setup/PluginSetup.sol';
-import {SimpleStorageBuild2} from './SimpleStorageBuild2.sol';
-
-/// @title SimpleStorageSetup build 2
-contract SimpleStorageBuild2Setup is PluginSetup {
- address private immutable simpleStorageImplementation;
-
- constructor() {
- simpleStorageImplementation = address(new SimpleStorageBuild2());
- }
-
- /// @inheritdoc IPluginSetup
- function prepareInstallation(
- address _dao,
- bytes memory _data
- ) external returns (address plugin, PreparedSetupData memory preparedSetupData) {
- (uint256 _number, address _account) = abi.decode(_data, (uint256, address));
-
- plugin = createERC1967Proxy(
- simpleStorageImplementation,
- abi.encodeWithSelector(SimpleStorageBuild2.initializeBuild2.selector, _dao, _number, _account)
- );
-
- PermissionLib.MultiTargetPermission[]
- memory permissions = new PermissionLib.MultiTargetPermission[](1);
-
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Grant,
- where: plugin,
- who: _dao,
- condition: PermissionLib.NO_CONDITION,
- permissionId: SimpleStorageBuild2(this.implementation()).STORE_PERMISSION_ID()
- });
-
- preparedSetupData.permissions = permissions;
- }
-
- /// @inheritdoc IPluginSetup
- function prepareUpdate(
- address _dao,
- uint16 _currentBuild,
- SetupPayload calldata _payload
- )
- external
- pure
- override
- returns (bytes memory initData, PreparedSetupData memory preparedSetupData)
- {
- (_dao, preparedSetupData);
-
- if (_currentBuild == 0) {
- address _account = abi.decode(_payload.data, (address));
- initData = abi.encodeWithSelector(
- SimpleStorageBuild2.initializeFromBuild1.selector,
- _account
- );
- }
- }
-
- /// @inheritdoc IPluginSetup
- function prepareUninstallation(
- address _dao,
- SetupPayload calldata _payload
- ) external view returns (PermissionLib.MultiTargetPermission[] memory permissions) {
- permissions = new PermissionLib.MultiTargetPermission[](1);
-
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Revoke,
- where: _payload.plugin,
- who: _dao,
- condition: PermissionLib.NO_CONDITION,
- permissionId: SimpleStorageBuild2(this.implementation()).STORE_PERMISSION_ID()
- });
- }
-
- /// @inheritdoc IPluginSetup
- function implementation() external view returns (address) {
- return simpleStorageImplementation;
- }
-}
-```
-
-The key thing to review in this new Plugin Setup contract is its `prepareUpdate()` function. The function only contains a condition checking from which build number the update is transitioning to build `2`. Here, it is the build number `1` as this is the only update path we support. Inside, we decode the `address _account` input argument provided with `bytes _date` and pass it to the `initializeFromBuild1` function taking care of intializing the storage that was added in this build.
-
-### 3. Future builds
-
-For each build we add, we will need to add a `prepareUpdate()` function with any parameters needed to update to that implementation.
-
-In this third build, for example, we are modifying the bytecode of the plugin.
-
-
-Third plugin build example, modifying the plugin's bytecode
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {IDAO, PluginUUPSUpgradeable} from '@aragon/osx/core/plugin/PluginUUPSUpgradeable.sol';
-
-/// @title SimpleStorage build 3
-contract SimpleStorageBuild3 is PluginUUPSUpgradeable {
- bytes32 public constant STORE_NUMBER_PERMISSION_ID = keccak256('STORE_NUMBER_PERMISSION'); // changed in build 3
- bytes32 public constant STORE_ACCOUNT_PERMISSION_ID = keccak256('STORE_ACCOUNT_PERMISSION'); // added in build 3
-
- uint256 public number; // added in build 1
- address public account; // added in build 2
-
- // added in build 3
- event NumberStored(uint256 number);
- event AccountStored(address account);
- error AlreadyStored();
-
- /// @notice Initializes the plugin when build 3 is installed.
- function initializeBuild3(
- IDAO _dao,
- uint256 _number,
- address _account
- ) external reinitializer(3) {
- __PluginUUPSUpgradeable_init(_dao);
- number = _number;
- account = _account;
-
- emit NumberStored({number: _number});
- emit AccountStored({account: _account});
- }
-
- /// @notice Initializes the plugin when the update from build 2 to build 3 is applied.
- /// @dev The initialization of `SimpleStorageBuild2` has already happened.
- function initializeFromBuild2() external reinitializer(3) {
- emit NumberStored({number: number});
- emit AccountStored({account: account});
- }
-
- /// @notice Initializes the plugin when the update from build 1 to build 3 is applied.
- /// @dev The initialization of `SimpleStorageBuild1` has already happened.
- function initializeFromBuild1(address _account) external reinitializer(3) {
- account = _account;
-
- emit NumberStored({number: number});
- emit AccountStored({account: _account});
- }
-
- function storeNumber(uint256 _number) external auth(STORE_NUMBER_PERMISSION_ID) {
- if (_number == number) revert AlreadyStored();
-
- number = _number;
-
- emit NumberStored({number: _number});
- }
-
- function storeAccount(address _account) external auth(STORE_ACCOUNT_PERMISSION_ID) {
- if (_account == account) revert AlreadyStored();
-
- account = _account;
-
- emit AccountStored({account: _account});
- }
-}
-```
-
-
-
-With each new build implementation, we will need to udate the Plugin Setup contract to be able to update to that new version. We do this through updating the `prepareUpdate()` function to support any new features that need to be set up.
-
-
-Third plugin setup example, modifying prepareUpdate function
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-
-pragma solidity 0.8.21;
-
-import {PermissionLib} from '@aragon/osx/core/permission/PermissionLib.sol';
-import {PluginSetup, IPluginSetup} from '@aragon/osx/framework/plugin/setup/PluginSetup.sol';
-import {SimpleStorageBuild2} from '../build2/SimpleStorageBuild2.sol';
-import {SimpleStorageBuild3} from './SimpleStorageBuild3.sol';
-
-/// @title SimpleStorageSetup build 3
-contract SimpleStorageBuild3Setup is PluginSetup {
- address private immutable simpleStorageImplementation;
-
- constructor() {
- simpleStorageImplementation = address(new SimpleStorageBuild3());
- }
-
- /// @inheritdoc IPluginSetup
- function prepareInstallation(
- address _dao,
- bytes memory _data
- ) external returns (address plugin, PreparedSetupData memory preparedSetupData) {
- (uint256 _number, address _account) = abi.decode(_data, (uint256, address));
-
- plugin = createERC1967Proxy(
- simpleStorageImplementation,
- abi.encodeWithSelector(SimpleStorageBuild3.initializeBuild3.selector, _dao, _number, _account)
- );
-
- PermissionLib.MultiTargetPermission[]
- memory permissions = new PermissionLib.MultiTargetPermission[](2);
-
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Grant,
- where: plugin,
- who: _dao,
- condition: PermissionLib.NO_CONDITION,
- permissionId: SimpleStorageBuild3(this.implementation()).STORE_NUMBER_PERMISSION_ID()
- });
-
- permissions[1] = permissions[0];
- permissions[1].permissionId = SimpleStorageBuild3(this.implementation())
- .STORE_ACCOUNT_PERMISSION_ID();
-
- preparedSetupData.permissions = permissions;
- }
-
- /// @inheritdoc IPluginSetup
- function prepareUpdate(
- address _dao,
- uint16 _currentBuild,
- SetupPayload calldata _payload
- )
- external
- view
- override
- returns (bytes memory initData, PreparedSetupData memory preparedSetupData)
- {
- if (_currentBuild == 0) {
- address _account = abi.decode(_payload.data, (address));
- initData = abi.encodeWithSelector(
- SimpleStorageBuild3.initializeFromBuild1.selector,
- _account
- );
- } else if (_currentBuild == 1) {
- initData = abi.encodeWithSelector(SimpleStorageBuild3.initializeFromBuild2.selector);
- }
-
- PermissionLib.MultiTargetPermission[]
- memory permissions = new PermissionLib.MultiTargetPermission[](3);
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Revoke,
- where: _dao,
- who: _payload.plugin,
- condition: PermissionLib.NO_CONDITION,
- permissionId: keccak256('STORE_PERMISSION')
- });
-
- permissions[1] = permissions[0];
- permissions[1].operation = PermissionLib.Operation.Grant;
- permissions[1].permissionId = SimpleStorageBuild3(this.implementation())
- .STORE_NUMBER_PERMISSION_ID();
-
- permissions[2] = permissions[1];
- permissions[2].permissionId = SimpleStorageBuild3(this.implementation())
- .STORE_ACCOUNT_PERMISSION_ID();
-
- preparedSetupData.permissions = permissions;
- }
-
- /// @inheritdoc IPluginSetup
- function prepareUninstallation(
- address _dao,
- SetupPayload calldata _payload
- ) external view returns (PermissionLib.MultiTargetPermission[] memory permissions) {
- permissions = new PermissionLib.MultiTargetPermission[](2);
-
- permissions[0] = PermissionLib.MultiTargetPermission({
- operation: PermissionLib.Operation.Revoke,
- where: _payload.plugin,
- who: _dao,
- condition: PermissionLib.NO_CONDITION,
- permissionId: SimpleStorageBuild3(this.implementation()).STORE_NUMBER_PERMISSION_ID()
- });
-
- permissions[1] = permissions[1];
- permissions[1].permissionId = SimpleStorageBuild3(this.implementation())
- .STORE_ACCOUNT_PERMISSION_ID();
- }
-
- /// @inheritdoc IPluginSetup
- function implementation() external view returns (address) {
- return simpleStorageImplementation;
- }
-}
-```
-
-
-
-In this case, the `prepareUpdate()` function only contains a condition checking from which build number the update is transitioning to build 2. Here, we can update from build 0 or build 1 and different operations must happen for each case to transition to `SimpleAdminBuild3`.
-
-In the first case, `initializeFromBuild1` is called taking care of intializing `address _account` that was added in build 1 and emitting the events added in build 2.
-
-In the second case, `initializeFromBuild2` is called taking care of intializing the build. Here, only the two events will be emitted.
-
-Lastly, the `prepareUpdate()` function takes care of modifying the permissions by revoking the `STORE_PERMISSION_ID` and granting the more specific `STORE_NUMBER_PERMISSION_ID` and `STORE_ACCOUNT)PERMISSION_ID` permissions, that are also granted if build 2 is freshly installed. This must happen for both update paths so this code is outside the `if` statements.
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/index.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/index.md
deleted file mode 100644
index 37ca2ad33..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/04-upgradeable-plugin/index.md
+++ /dev/null
@@ -1,33 +0,0 @@
----
-title: Upgradeable Plugins
----
-
-## How to develop an Upgradeable Plugin
-
-Upgradeable contracts offer advantages because you can cheaply change or fix the logic of your contract without losing the storage of your contract. If you want to review plugin types in depth, check out our [guide on plugin types here](../02-plugin-types.md).
-
-The drawbacks however, are that:
-
-- there are plenty of ways to make a mistake, and
-- the changeable logic poses a new attack surface.
-
-Although we've abstracted away most of the complications of the upgrade process through our `PluginUUPSUpgradeable` base class, please know that writing an upgradeable contract is an advanced topic.
-
-### Prerequisites
-
-- You have read about the different [plugin types](../02-plugin-types.md) and decided to develop an upgradeable plugin being deployed via the [UUPS pattern (ERC-1822)](https://eips.ethereum.org/EIPS/eip-1822).
-- You know how to write a [non-upgradeable plugin](../03-non-upgradeable-plugin/index.md).
-- You know about the difficulties and pitfalls of ["Writing Upgradeable Contracts"](https://docs.openzeppelin.com/upgrades-plugins/1.x/writing-upgradeable) that come with
- - modifiying the storage layout
- - initialization
- - inheritance
- - leaving storage gaps
-
-Up next, check out our guides on:
-
-1. [How to initialize an Upgradeable Plugins](./01-initialization.md)
-2. [How to build the implementation of an Upgradeable Plugin](./02-implementation.md)
-3. [How to build and deploy a Plugin Setup contract for an Upgradeable Plugin](./03-setup.md)
-4. [How to create a subsequent build implementation to an Upgradeable Plugin](./04-subsequent-builds.md)
-5. [How to upgrade an Upgradeable Plugin](./05-updating-versions.md)
-6. [How to publish my plugin into the Aragon OSx protocol](../07-publication/index.md)
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/05-governance-plugins/01-proposals.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/05-governance-plugins/01-proposals.md
deleted file mode 100644
index e515dc093..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/05-governance-plugins/01-proposals.md
+++ /dev/null
@@ -1,43 +0,0 @@
----
-title: Proposals
----
-
-## The `IProposal` Interface
-
-:::note
-This page is a stub and work in progress
-:::
-
-Create and execute proposals containing actions and a description.
-
-### Interface `IProposal`
-
-```solidity
-interface IProposal {
- /// @notice Emitted when a proposal is created.
- /// @param proposalId The ID of the proposal.
- /// @param creator The creator of the proposal.
- /// @param startDate The start date of the proposal in seconds.
- /// @param endDate The end date of the proposal in seconds.
- /// @param metadata The metadata of the proposal.
- /// @param actions The actions that will be executed if the proposal passes.
- /// @param allowFailureMap A bitmap allowing the proposal to succeed, even if individual actions might revert. If the bit at index `i` is 1, the proposal succeeds even if the `i`th action reverts. A failure map value of 0 requires every action to not revert.
- event ProposalCreated(
- uint256 indexed proposalId,
- address indexed creator,
- uint64 startDate,
- uint64 endDate,
- bytes metadata,
- IDAO.Action[] actions,
- uint256 allowFailureMap
- );
-
- /// @notice Emitted when a proposal is executed.
- /// @param proposalId The ID of the proposal.
- event ProposalExecuted(uint256 indexed proposalId);
-
- /// @notice Returns the proposal count determining the next proposal ID.
- /// @return The proposal count.
- function proposalCount() external view returns (uint256);
-}
-```
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/05-governance-plugins/02-membership.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/05-governance-plugins/02-membership.md
deleted file mode 100644
index 24d829e34..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/05-governance-plugins/02-membership.md
+++ /dev/null
@@ -1,44 +0,0 @@
----
-title: Membership
----
-
-## The `IMembership` Interface
-
-:::note
-This page is a stub and work in progress
-:::
-
-Introduce members to the DAO upon installation through [the `IMembership` interface](./02-membership.md).
-
-### Interface IMembership
-
-```solidity title=
-/// @notice An interface to be implemented by DAO plugins that define membership.
-interface IMembership {
- /// @notice Emitted when members are added to the DAO plugin.
- /// @param members The list of new members being added.
- event MembersAdded(address[] members);
-
- /// @notice Emitted when members are removed from the DAO plugin.
- /// @param members The list of existing members being removed.
- event MembersRemoved(address[] members);
-
- /// @notice Emitted to announce the membership being defined by a contract.
- /// @param definingContract The contract defining the membership.
- event MembershipContractAnnounced(address indexed definingContract);
-
- /// @notice Checks if an account is a member of the DAO.
- /// @param _account The address of the account to be checked.
- /// @return Whether the account is a member or not.
- /// @dev This function must be implemented in the plugin contract that introduces the members to the DAO.
- function isMember(address _account) external view returns (bool);
-}
-```
-
-### Introducing Members directly
-
-```solidity
-event MembersAdded(address[] members)
-
-event MembersRemoved(address[] members)
-```
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/05-governance-plugins/index.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/05-governance-plugins/index.md
deleted file mode 100644
index b43f7807d..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/05-governance-plugins/index.md
+++ /dev/null
@@ -1,41 +0,0 @@
----
-title: Governance Plugins
----
-
-## How to Build a Governance Plugin
-
-One of the most common use cases for plugins are governance plugins. Governance plugins are plugins DAOs install to help them make decisions.
-
-### What are Governance Plugins
-
-Governance plugins are characterized by the **ability to execute actions in the DAO** they have been installed to. Accordingly, the `EXECUTE_PERMISSION_ID` is granted on installation on the installing DAO to the governance plugin contract.
-
-```solidity
-grant({
- where: installingDao,
- who: governancePlugin,
- permissionId: EXECUTE_PERMISSION_ID
-});
-```
-
-Beyond this fundamental ability, governance plugins usually implement two interfaces:
-
-- [The `IProposal` interface](./01-proposals.md) introducing the **notion of proposals** and how they are created and executed.
-- [The `IMembership` interface](./02-membership.md) introducing the **notion of membership** to the DAO.
-
-### Examples of Governance Plugins
-
-Some examples of governance plugins are:
-
-- [A token-voting plugin](https://github.com/aragon/osx/tree/develop/packages/contracts/src/plugins/governance/majority-voting/token): Results are based on what the majority votes and the vote's weight is determined by how many tokens an account holds. Ex: Alice has 10 tokens, Bob 2, and Alice votes yes, the yes wins.
-- [Multisig plugin](https://github.com/aragon/osx/tree/develop/packages/contracts/src/plugins/governance/multisig): A determined set of addresses is able to approve. Once `x` amount of addresses approve (as determined by the plugin settings), then the proposal automatically succeeds.
-- [Admin plugin](https://github.com/aragon/osx/tree/develop/packages/contracts/src/plugins/governance/admin): One address can create and immediately execute proposals on the DAO (full control).
-- [Addresslist plugin](https://github.com/aragon/osx/tree/develop/packages/contracts/src/plugins/governance/majority-voting/addresslist): Majority-based voting, where list of addresses are able to vote in decision-making for the organization. Unlike a multisig, everybody here is expected to vote yes/no/abstain within a certain time frame.
-
-:::note
-More tutorials on how to build governance plugins coming soon.
-:::
-
-
-
-
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/06-meta-tx-plugins.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/06-meta-tx-plugins.md
deleted file mode 100644
index a195e393c..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/06-meta-tx-plugins.md
+++ /dev/null
@@ -1,46 +0,0 @@
----
-title: Meta Transactions
----
-
-## Support for Meta Transactions
-
-Our plugins are compatible with the [ERC-2771 (Meta Transaction)](https://eips.ethereum.org/EIPS/eip-2771) standard, which allows users to send gasless transactions, also known as meta transactions.
-This is possible because we use `_msgSender` and `_msgData` context from OpenZeppelin's `Context` and `ContextUpgradeable` in our `Plugin`, `PluginCloneable`, and `PluginUUPSUpgradeable` classes.
-
-To support meta transactions, your implementation contract must inherit and override the `Context` implementation with the `_msgSender` and `_msgData` functions provided in OpenGSN's `BaseRelayRecipient`, and use the DAO's trusted forwarder.
-
-Below we show for the example of the `TokenVoting` plugin how you can make an existing plugin contract meta-transaction compatible.
-
-```solidity
-import {ContextUpgradeable} from '@openzeppelin/contracts-upgradeable/utils/ContextUpgradeable.sol';
-import {BaseRelayRecipient} from '@opengsn/contracts/src/BaseRelayRecipient.sol';
-
-contract MyPlugin is PluginUUPSUpgradeable, BaseRelayRecipient {
- function initialize(IDAO _dao) external initializer {
- __PluginUUPSUpgradeable_init(_dao);
- _setTrustedForwarder(dao.getTrustedForwarder());
- }
-
- // ... the implementation
-
- function _msgSender()
- internal
- view
- virtual
- override(ContextUpgradeable, BaseRelayRecipient)
- returns (address)
- {
- return BaseRelayRecipient._msgSender();
- }
-
- function _msgData()
- internal
- view
- virtual
- override(ContextUpgradeable, BaseRelayRecipient)
- returns (bytes calldata)
- {
- return BaseRelayRecipient._msgData();
- }
-}
-```
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/07-publication/01-versioning.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/07-publication/01-versioning.md
deleted file mode 100644
index 89348f29c..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/07-publication/01-versioning.md
+++ /dev/null
@@ -1,59 +0,0 @@
----
-title: New Plugin Version
----
-
-## How to add a new version of your plugin
-
-The Aragon OSx protocol has an on-chain versioning system built-in, which distinguishes between releases and builds.
-
-- **Releases** contain breaking changes, which are incompatible with preexisting installations. Updates to a different release are not possible. Instead, you must install the new plugin release and uninstall the old one.
-- **Builds** are minor/patch versions within a release, and they are meant for compatible upgrades only (adding a feature or fixing a bug without changing anything else).
-
-Builds are particularly important for `UUPSUpgradeable` plugins, whereas a non-upgradeable plugin will work off of only releases.
-
-Given a version tag `RELEASE.BUILD`, we can infer that:
-
-1. We are doing a `RELEASE` version when we apply breaking changes affecting the interaction with other contracts on the blockchain to:
-
- - The `Plugin` implementation contract such as the
-
- - change or removal of storage variables
- - removal of external functions
- - change of external function headers
-
-2. We are doing a `BUILD` version when we apply backward compatible changes not affecting the interaction with other contracts on the blockchain to:
-
- - The `Plugin` implementation contract such as the
-
- - addition of
-
- - storage variables
- - external functions
-
- - change of
-
- - external function bodies
-
- - addition, change, or removal of
-
- - internal functions
- - constants
- - immutables
- - events
- - errors
-
- - The `PluginSetup` contract such as
-
- - addition, change, or removal of
-
- - input parameters
- - helper contracts
- - requested permissions
-
- - The release and build `metadata` URIs such as the
-
- - change of
-
- - the plugin setup ABI
- - the plugin UI components
- - the plugin description
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/07-publication/02-metadata.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/07-publication/02-metadata.md
deleted file mode 100644
index bfe6d57c1..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/07-publication/02-metadata.md
+++ /dev/null
@@ -1,122 +0,0 @@
----
-title: Plugin Metadata
----
-
-## Plugin Metadata Specification
-
-The plugin metadata is necessary to allow the App frontend to interact with any plugins:
-
-- Now: generic setup (installation, update, uninstallation)
- - Allows the frontend to render the necessary fields for the input being required to setup the plugin (e.g., the list of initial members of the Multisig plugin)
-- Future: render a UI in a generic way (buttons, text fields, flows) within the specs of the Open Design System (ODS) (e.g. manage the list of Multisig members or the approval settings)
-
-Currently, two kinds of metadata exist:
-
-1. Release metadata
-2. Build metadata
-
-### Release Metadata
-
-The release metadata is a `.json` file stored on IPFS with its IPFS CID published for each release in the [PluginRepo](../../../01-how-it-works/02-framework/02-plugin-management/01-plugin-repo/index.md) (see also the section about [versioning](../07-publication/01-versioning.md#)).
-
-The intention is to provide an appealing overview of each releases functionality.
-It can be updated with each call to [`createVersion()`](../../../03-reference-guide/framework/plugin/repo/IPluginRepo.md#external-function-createversion) in `IPluginRepo` by the repo maintainer.
-It can be replaced at any time with [`updateReleaseMetadata()`](../../../03-reference-guide/framework/plugin/repo/IPluginRepo.md#external-function-updatereleasemetadata) in `IPluginRepo` by the repo maintainer.
-
-The `release-metadata.json` file consists of the following entries:
-
-| Key | Type | Description |
-| ----------- | ----------- | ---------------------------------------------------------------------------- |
-| name | `string` | Name of the plugin (e.g. `"Multisig"`) |
-| description | `string` | Description of the plugin release and its functionality. |
-| images | UNSPECIFIED | Optional. Contains a series of images advertising the plugins functionality. |
-
-#### Example
-
-```json
-{
- "name": "Multisig",
- "description": "",
- "images": {}
-}
-```
-
-### Build Metadata
-
-The build metadata is a `.json` file stored on IPFS with its IPFS CID published for each build **only once** in the [PluginRepo](../../../01-how-it-works/02-framework/02-plugin-management/01-plugin-repo/index.md) (see also the section about [versioning](../07-publication/01-versioning.md#)).
-
-The intention is to inform about the changes that were introduced in this build compared to the previous one and give instructions to the App frontend and other users on how to interact with the plugin setup and implementation contract.
-It can be published **only once** with the call to [`createVersion()`](../../../03-reference-guide/framework/plugin/repo/IPluginRepo.md#external-function-createversion) in `IPluginRepo` by the repo maintainer.
-
-| Key | Type | Description |
-| ----------- | ----------- | --------------------------------------------------------------------------------------------------------- |
-| ui | UNSPECIFIED | A special formatted object containing instructions for the App frontend on how to render the plugin's UI. |
-| change | `string` | Description of the code and UI changes compared to the previous build of the same release. |
-| pluginSetup | `object` | Optional. Contains a series of images advertising the plugins functionality. |
-
-Each build metadata contains the following fields:
-
-- one `"prepareInstallation"` object
-- one `"prepareUninstallation"` object
-- 0 to N `"prepareUpdate"` objects enumerated from 1 to N+1
-
-Each `"prepare..."` object contains:
-
-| Key | Type | Description |
-| ----------- | ---------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| description | `string` | The description of what this particular setup step is doing and what it requires the input for. |
-| inputs | `object[]` | A description of the inputs required for this setup step following the [Solidity JSON ABI](https://docs.ethers.org/v5/api/utils/abi/formats/#abi-formats--solidity) format enriched with an additional `"description"` field for each element. |
-
-By following the Solidity JSON ABI format for the inputs, we followed an establishd standard, have support for complex types (tuples, arrays, nested versions of the prior) and allow for future extensibility (such as the human readable description texts that we have added).
-
-#### Example
-
-```json
-{
- "ui": {},
- "change": "- The ability to create a proposal now depends on the membership status of the current instead of the snapshot block.\n- Added a check ensuring that the initial member list cannot overflow.",
- "pluginSetup": {
- "prepareInstallation": {
- "description": "The information required for the installation.",
- "inputs": [
- {
- "internalType": "address[]",
- "name": "members",
- "type": "address[]",
- "description": "The addresses of the initial members to be added."
- },
- {
- "components": [
- {
- "internalType": "bool",
- "name": "onlyListed",
- "type": "bool",
- "description": "Whether only listed addresses can create a proposal or not."
- },
- {
- "internalType": "uint16",
- "name": "minApprovals",
- "type": "uint16",
- "description": "The minimal number of approvals required for a proposal to pass."
- }
- ],
- "internalType": "struct Multisig.MultisigSettings",
- "name": "multisigSettings",
- "type": "tuple",
- "description": "The inital multisig settings."
- }
- ],
- "prepareUpdate": {
- "1": {
- "description": "No input is required for the update.",
- "inputs": []
- }
- },
- "prepareUninstallation": {
- "description": "No input is required for the uninstallation.",
- "inputs": []
- }
- }
- }
-}
-```
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/07-publication/index.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/07-publication/index.md
deleted file mode 100644
index 6187a414a..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/07-publication/index.md
+++ /dev/null
@@ -1,23 +0,0 @@
----
-title: Publication of your Plugin into Aragon OSx
----
-
-## How to publish a plugin into Aragon's plugin registry
-
-Once you've deployed your Plugin Setup contract, you will be able to publish your plugin into Aragon's plugin registry so any Aragon DAO can install it.
-
-### 1. Make sure your plugin is deployed in the right network
-
-Make sure your Plugin Setup contract is deployed in your network of choice (you can find all of the networks we support [here](https://github.com/aragon/osx-commons/tree/develop/configs/src/deployments/json)). You will need the address of your Plugin Setup contract to be able to publish the plugin into the protocol.
-
-### 2. Publishing your plugin
-
-Every plugin in Aragon can have future versions, so when publishing a plugin to the Aragon protocol, we're really creating a [`PluginRepo`](https://github.com/aragon/osx/blob/develop/packages/contracts/src/framework/plugin/repo/PluginRepo.sol) instance for each plugin, which will contain all of the plugin's versions.
-
-To publish a plugin, we will use Aragon's `PluginRepoFactory` contract - in charge of creating `PluginRepo` instances containing your plugin's versions. To do this, we will call its `createPluginRepoWithFirstVersion` function, which will [create the first version of a plugin](https://github.com/aragon/core/blob/develop/packages/contracts/src/framework/plugin/repo/PluginRepoFactory.sol#L48) and add that new `PluginRepo` address into the `PluginRepoRegistry` containing all available plugins within the protocol.
-
-You can find all of the addresses of `PluginRepoFactory` contracts by network [here](https://github.com/aragon/osx-commons/tree/develop/configs/src/deployments/json).
-
-To create more versions of your plugin in the future, you'll call on the [`createVersion` function](https://github.com/aragon/osx/blob/develop/packages/contracts/src/framework/plugin/repo/PluginRepo.sol#L128) from the `PluginRepo` instance of your plugin. When you publish your plugin, you'll be able to find the address of your plugin's `PluginRepo` instance within the transaction data.
-
-To deploy your plugin, follow the steps in the [`osx-plugin-template-hardhat` README.md](https://github.com/aragon/osx-plugin-template-hardhat/blob/main/README.md#deployment).
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/index.md b/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/index.md
deleted file mode 100644
index 869b37a71..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/02-plugin-development/index.md
+++ /dev/null
@@ -1,211 +0,0 @@
----
-title: How to build a DAO Plugin
----
-
-## Plugin Development Quickstart Guide
-
-Plugins are how we extend the functionality for DAOs. In Aragon OSx, everything a DAO can do is based on Plugin functionality enabled through permissions.
-
-In this Quickstart guide, we will build a Greeter Plugin which returns "Hello World!".
-
-## Hello, World!
-
-### 1. Setup
-
-First, let's [create a Hardhat project](https://hardhat.org/tutorial/creating-a-new-hardhat-project).
-
-```bash
-mkdir aragon-plugin-tutorial
-cd aragon-plugin-tutorial
-yarn init
-yarn add --dev hardhat
-npx hardhat
-```
-
-You'll want to select an empty Hardhat project to get started.
-
-```
-$ npx hardhat
-888 888 888 888 888
-888 888 888 888 888
-888 888 888 888 888
-8888888888 8888b. 888d888 .d88888 88888b. 8888b. 888888
-888 888 "88b 888P" d88" 888 888 "88b "88b 888
-888 888 .d888888 888 888 888 888 888 .d888888 888
-888 888 888 888 888 Y88b 888 888 888 888 888 Y88b.
-888 888 "Y888888 888 "Y88888 888 888 "Y888888 "Y888
-
-👷 Welcome to Hardhat v2.9.9 👷
-
-? What do you want to do? …
- Create a JavaScript project
- Create a TypeScript project
-❯ Create an empty hardhat.config.js
- Quit
-```
-
-Then, you'll want to import the Aragon OSx contracts inside your Solidity project.
-
-```bash
-yarn add @aragon/osx
-```
-
-or
-
-```bash
-npm install @aragon/osx
-```
-
-Now that we have OSx within our project, we can start developing our plugin implementation.
-
-### 2. GreeterPlugin
-
-We'll create a Greeter Plugin which returns a "Hello World!" string when calling on `greet()`.
-
-In order to do this, we'll create a `GreeterPlugin.sol` file. This is where all of our plugin logic will live.
-
-```bash
-mkdir contracts && cd contracts
-touch GreeterPlugin.sol
-```
-
-Inside the `GreeterPlugin.sol`, we want to:
-
-- Pass the DAO the plugin will be using within the constructor. This will enable us to install a Plugin into a DAO.
-- Add the greeter function.
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {Plugin, IDAO} from '@aragon/osx/core/plugin/Plugin.sol';
-
-contract GreeterPlugin is Plugin {
- constructor(IDAO _dao) Plugin(_dao) {}
-
- function greet() external pure returns (string memory) {
- return 'Hello world!';
- }
-}
-```
-
-### 3. GreeterSetup
-
-Once we're done with the plugin logic, we want to write a Setup contract.
-
-The Setup contract contains the instructions to be called whenever this plugin is installed, uninstalled or upgraded for a DAO. It is the one in charge of setting the permissions that enable the plugin execute actions on the DAO.
-
-Let's create our GreeterSetup contract inside your `contracts` folder:
-
-```bash
-touch GreeterSetup.sol
-```
-
-Inside the file, we'll add the `prepareInstallation` and `prepareUninstallation` functions. These are the functions that will get called to install/uninstall the plugin into a DAO.
-
-```solidity
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {PermissionLib} from '@aragon/osx/core/permission/PermissionLib.sol';
-import {PluginSetup} from '@aragon/osx/framework/plugin/setup/PluginSetup.sol';
-import './GreeterPlugin.sol';
-
-contract GreeterSetup is PluginSetup {
- function prepareInstallation(
- address _dao,
- bytes memory
- ) external returns (address plugin, PreparedSetupData memory /*preparedSetupData*/) {
- plugin = address(new GreeterPlugin(IDAO(_dao)));
- }
-
- function prepareUninstallation(
- address _dao,
- SetupPayload calldata _payload
- ) external pure returns (PermissionLib.MultiTargetPermission[] memory /*permissions*/) {
- (_dao, _payload);
- }
-
- function implementation() external view returns (address) {}
-}
-```
-
-### 4. Deploy the Plugin
-
-To publish the plugin into the Aragon protocol, we first need to deploy the `PluginSetup.sol` contract to our network of choice. We can deploy it using [Hardhat's deploy script](https://hardhat.org/tutorial/deploying-to-a-live-network).
-
-In order to deploy directly from Hardhat, we'll use [Hardhat's Toolbox](https://hardhat.org/hardhat-runner/plugins/nomicfoundation-hardhat-toolbox).
-
-```bash
-yarn add @nomicfoundation/hardhat-toolbox
-```
-
-Then, we can create a folder called `scripts` and inside of it, we'll add our deploy script.
-
-```bash
-mkdir scripts && touch scripts/deploy.cjs
-```
-
-Inside that file, we will add our deploy script.
-
-```js
-const hre = require('hardhat');
-
-async function main() {
- const [deployer] = await hre.ethers.getSigners();
-
- console.log('Deploying contracts with the account:', deployer.address);
- console.log('Account balance:', (await deployer.getBalance()).toString());
-
- const getGreeterSetup = await hre.ethers.getContractFactory('GreeterSetup');
- const GreeterSetup = await getGreeterSetup.deploy();
-
- console.log('GreeterSetup address:', GreeterSetup.address);
-}
-
-main()
- .then(() => process.exit(0))
- .catch(error => {
- console.error(error);
- process.exit(1);
- });
-```
-
-On the terminal, we should then see something like this:
-
-```bash
-Deploying contracts with the account: 0xf39Fd6e51aad88F6F4ce6aB8827279cffFb92266
-Account balance: 10000000000000000000000
-GreeterSetup address: 0x5FbDB2315678afecb367f032d93F642f64180aa3
-```
-
-### 5. Publish the Plugin to the Aragon protocol
-
-Lastly, we can call the [`createPluginRepoWithFirstVersion` function from Aragon's `PluginRepoFactory`](../../03-reference-guide/framework/plugin/repo/PluginRepoFactory.md) passing it the address of your deployed `GreeterSetup` contract and the first version of your Plugin will be published into the protocol!
-
-We can do this directly by calling the function on Etherscan ([make sure to get the right scan and contract address based on your network](https://github.com/aragon/osx-commons/tree/develop/configs/src/deployments/json)) or through locally calling on the method from your project using Ethers.
-
-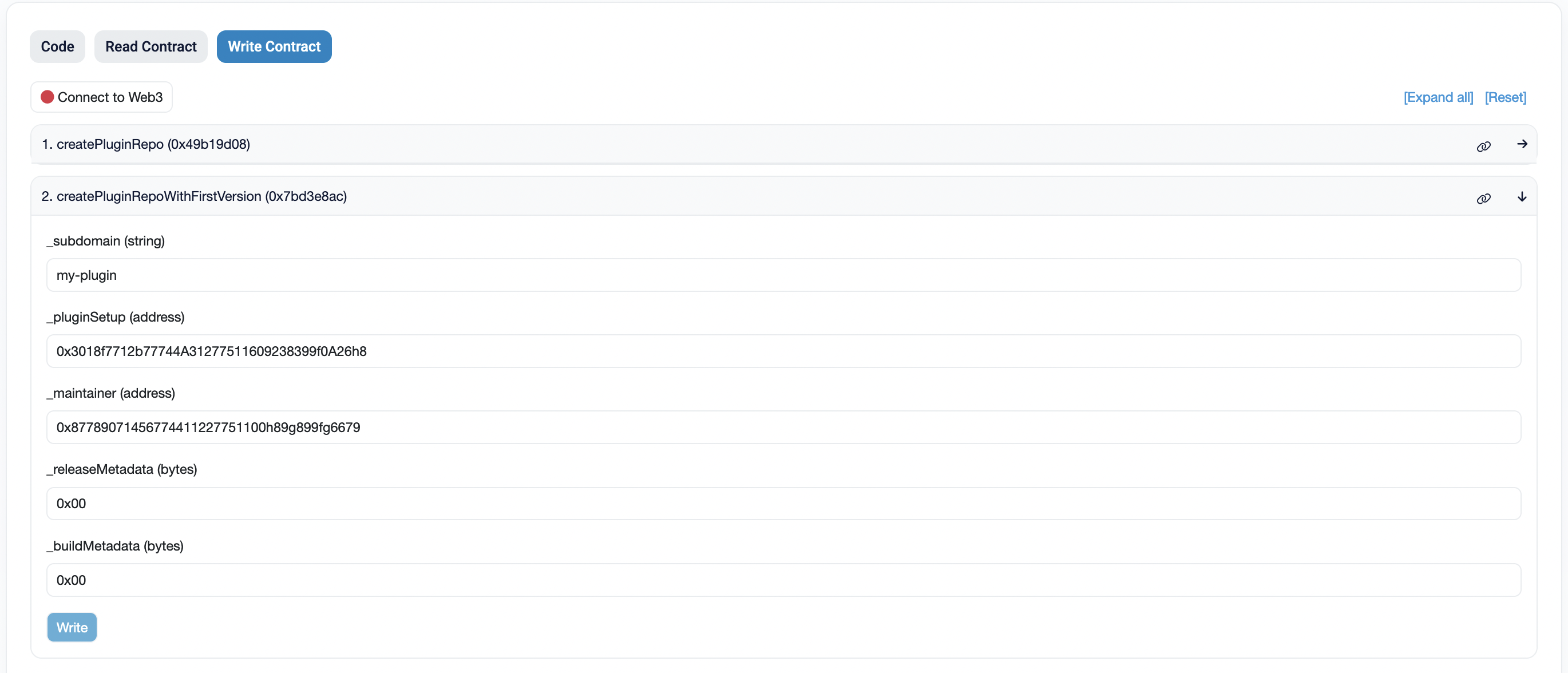
-
-If you want to review how to publish your plugin in more depth, review our [How to Publish a Plugin in Aragon OSx guide here](./07-publication//index.md)
-
-### Next Steps
-
-Congratulations 🎉! You have developed a plugin that every Aragon DAO will be able to use.
-
-Currently, it is not doing much. Let's change this by adding additional functionality. You check out our [existing plugins](https://github.com/aragon/osx/tree/develop/packages/contracts/src/plugins) as inspiration.
-
-You could also make it:
-
-- [a non-upgradeable governance plugin](./03-non-upgradeable-plugin/index.md)
-- [an upgradeable plugin (advanced)](./04-upgradeable-plugin/index.md)
-
-But first, let's have a look at:
-
-- [best practices and patterns](./01-best-practices.md)
-- [different plugin deployment types](./02-plugin-types.md)
-
-And if you want to add additional versions to it, check out our guides on:
-
-- [How to publish a plugin](./07-publication/index.md)
-- [How to manage plugin versioning](./07-publication/01-versioning.md)
diff --git a/packages/contracts/docs/developer-portal/02-how-to-guides/index.md b/packages/contracts/docs/developer-portal/02-how-to-guides/index.md
deleted file mode 100644
index 2bf688431..000000000
--- a/packages/contracts/docs/developer-portal/02-how-to-guides/index.md
+++ /dev/null
@@ -1,49 +0,0 @@
----
-title: How-to Guides
----
-
-## Welcome to our How To Guides on Using the Aragon OSx Protocol!
-
-With a few lines of code, the Aragon OSx protocol allows you create, manage, and change your on-chain organizations, through extending functionality for DAOs through the installation and uninstallation of plugins.
-
-The organizations that survive the longest are the ones that easily adapt to changing circumstances. DAOs too need a way to adapt and evolve, even if they’re governed on an immutable blockchain.
-
-This is where Plugins come in!
-
-### DAO Plugins
-
-DAO Plugins are smart contracts extending the functionality for DAOs
-
-Some examples of DAO Plugins are:
-
-- 💰 Treasury management tools (i.e. staking, yield distributions, etc),
-- 👩🏾⚖️ Governance mechanisms for collective decision-making (i.e. NFT voting, multi-sig voting, etc)
-- 🔌 Integrations with other ecosystem projects (i.e. Snapshot off-chain voting with Aragon on-chain execution, AI-enabled decision-makers, etc)
-- …. basically anything you’d like your DAO to do!
-
-In the Aragon OSx protocol, everything a DAO does is decided and implemented through plugins.
-
-Technically speaking, Aragon DAOs are:
-
-- 💳 A treasury: holding all of the DAO’s assets, and
-- 🤝 A permission management system: protecting the assets, through checking that only addresses with x permissions can execute actions on behalf of the DAO.
-
-All other functionality is enabled through plugins. This allows DAOs to be extremely flexible and modular as they mature, through installing and uninstalling these plugins as needs arise.
-
-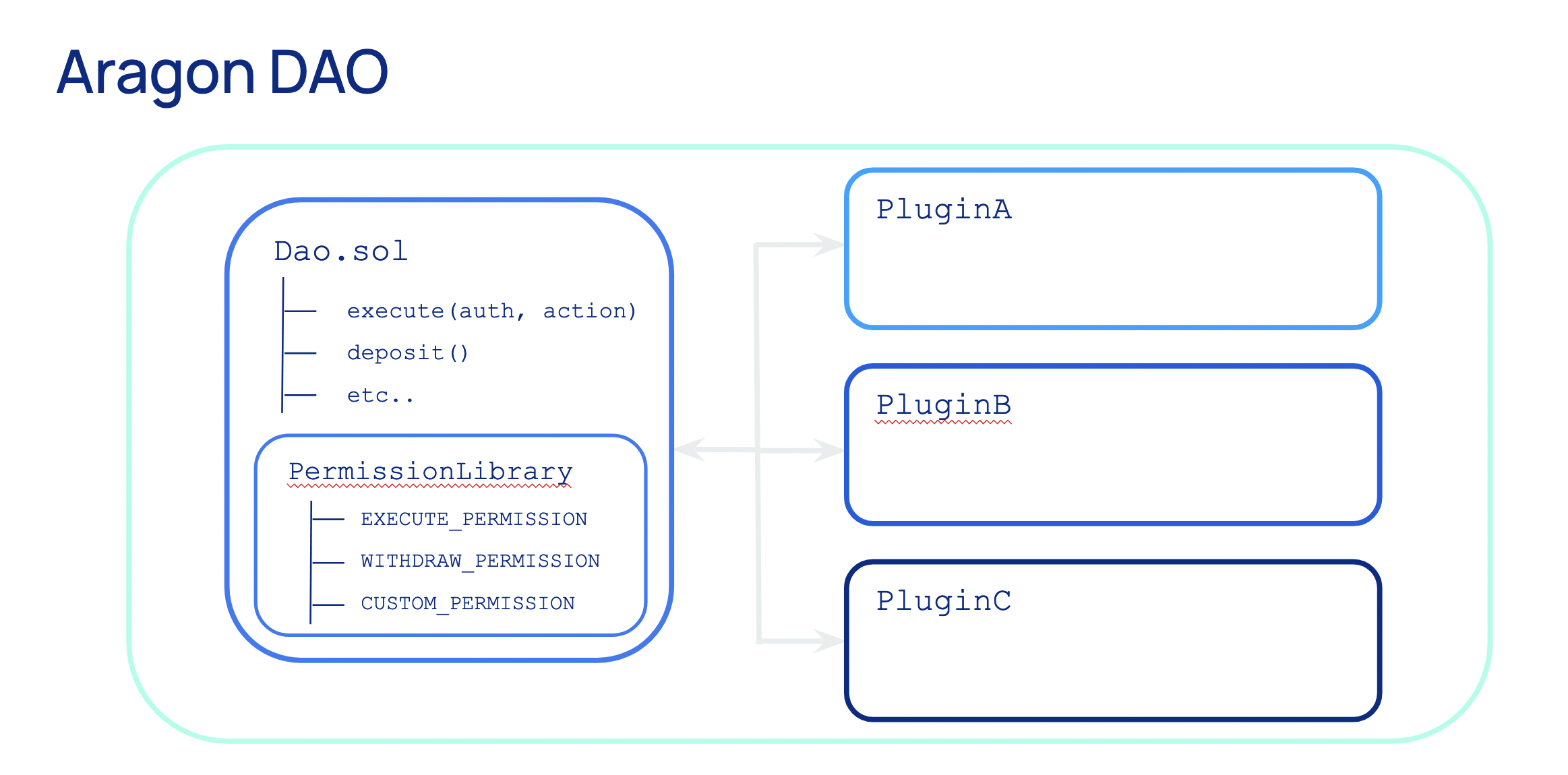
-
-On the technical level, plugins are composed of two key contracts:
-
-- ⚡️ The Plugin implementation contract: containing all of the logic and functionality for your DAO, and
-- 👩🏻🏫 The Plugin Setup contract: containing the installation, uninstallation and upgrade instructions for your plugin.
-
-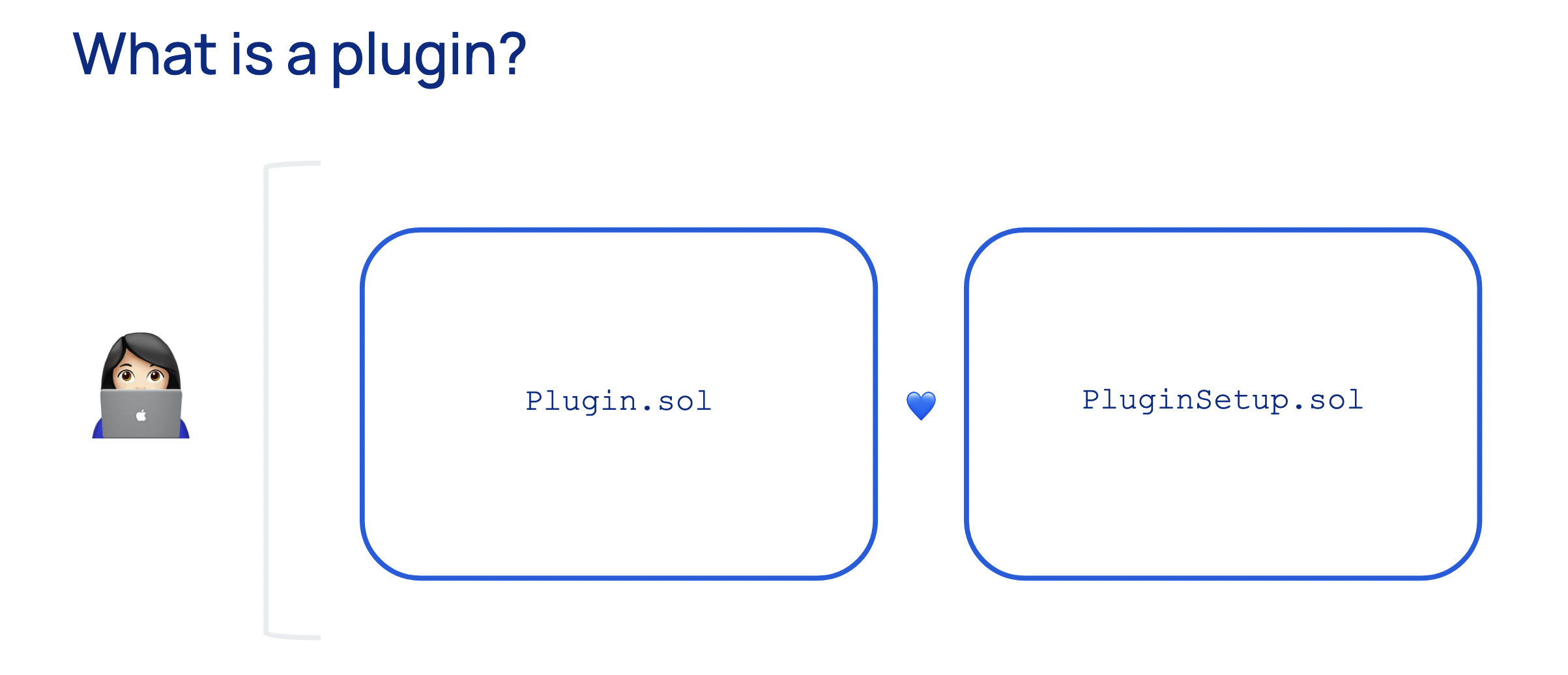
-
-Through plugins, we provide a secure, flexible way for on-chain organizations to iterate as they grow.
-
-We enable everyone to experiment with governance at the speed of software!
-
-Check out our How-To-Guides on:
-
-- [How to Develop your own Plugin](./02-plugin-development/index.md)
-- [How to Operate your DAO](./01-dao/index.md)
diff --git a/packages/contracts/docs/developer-portal/03-reference-guide/index.md b/packages/contracts/docs/developer-portal/03-reference-guide/index.md
deleted file mode 100644
index 499bb5499..000000000
--- a/packages/contracts/docs/developer-portal/03-reference-guide/index.md
+++ /dev/null
@@ -1,7 +0,0 @@
----
-title: Reference Guide
----
-
-## Reference Guide
-
-This reference is automatically generated from the [Solidity NatSpec comments](https://docs.soliditylang.org/en/develop/natspec-format.html) of the contracts in the [aragon/osx GitHub](https://github.com/aragon/osx) repository.
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/aragon-osx.svg b/packages/contracts/docs/developer-portal/04-framework-lifecycle/aragon-osx.svg
deleted file mode 100644
index 9f58c8dd6..000000000
--- a/packages/contracts/docs/developer-portal/04-framework-lifecycle/aragon-osx.svg
+++ /dev/null
@@ -1 +0,0 @@
-
\ No newline at end of file
diff --git a/packages/contracts/docs/developer-portal/index.md b/packages/contracts/docs/developer-portal/index.md
deleted file mode 100644
index e3e43afbf..000000000
--- a/packages/contracts/docs/developer-portal/index.md
+++ /dev/null
@@ -1,61 +0,0 @@
----
-title: Aragon OSx
-sidebar_label: Introduction to Aragon OSx
-sidebar_position: 0
----
-
-## The Contracts Behind the Protocol
-
-The Aragon OSx protocol is the foundation layer of the new Aragon stack. It allows users to create, manage, and customize DAOs in a way that is lean, adaptable, and secure.
-
-The Aragon OSx protocol architecture is composed of two key sections:
-
-- **Core contracts**: the primitives the end user will interact with. It is composed of 3 parts:
- - **DAO contract:** the main contract of our protocol. It holds a DAO's assets and possible actions.
- - **Permissions**: govern interactions between the plugins, DAOs, and any other address - allowing them (or not) to execute actions on behalf of and within the DAO.
- - **Plugins**: base templates of plugins.
-- **Framework contracts**: in charge of creating and registering each deployed DAO or plugin. It contains:
- - **DAO and Plugin Repository Factories**: creates DAOs or plugins.
- - **DAO and Plugin Registries**: registers into our protocol those DAOs or plugins.
- - **Plugin Setup Processor:** installs and uninstalls plugins into DAOs.
-
-Through permissions and plugins, DAO builders are able to build and customize their DAO to suit their needs.
-
-## Getting Started
-
-Users interact with the Aragon OSx protocol through the [Aragon App](https://app.aragon.org), the [Aragon SDK](https://devs.aragon.org/docs/sdk), or directly calling on the [protocol contracts](https://github.com/aragon/osx) - as well as through any third-party projects built using our stack.
-
-To **add the contracts to your project**, open a terminal in the root folder of your Solidity project and run:
-
-```shell
-yarn add @aragon/osx
-```
-
-Then, to use the contracts within your project, **import the contracts** through something like:
-
-
-
-```solidity title="MyCoolPlugin.sol"
-// SPDX-License-Identifier: AGPL-3.0-or-later
-pragma solidity 0.8.21;
-
-import {Plugin, IDAO} from '@aragon/osx/core/plugin/Plugin.sol';
-
-contract MyCoolPlugin is Plugin {
- // ...
-}
-```
-
-## Customize your DAO
-
-DAO Plugins are the best way to customize your DAO. These are modular extendable pieces of software which you can install or uninstall from your DAO as it evolves and grows.
-
-To learn more about plugins, check out our guide [here](./02-how-to-guides/02-plugin-development/index.md).
-
-### Walkthrough
-
-This documentation is divided into conceptual and practical sections as well as the reference guide.
-
-- Conceptual [How It Works articles](01-how-it-works/index.md) explain the architecture behind our protocol.
-- Practical [How-to Guides](02-how-to-guides/index.md) explain how to use and leverage our protocol.
-- The [Reference Guide](03-reference-guide/index.md) generated from the NatSpec comments of the latest `@aragon/osx` release documents each individual Solididty contract, function, and variable.
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/01-systems.md b/packages/contracts/docs/framework-lifecycle/01-systems.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/01-systems.md
rename to packages/contracts/docs/framework-lifecycle/01-systems.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/02-semver.md b/packages/contracts/docs/framework-lifecycle/02-semver.md
similarity index 96%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/02-semver.md
rename to packages/contracts/docs/framework-lifecycle/02-semver.md
index 7f549bca7..3d44c9e4e 100644
--- a/packages/contracts/docs/developer-portal/04-framework-lifecycle/02-semver.md
+++ b/packages/contracts/docs/framework-lifecycle/02-semver.md
@@ -38,7 +38,7 @@ We now classify [smart contract changes](01-systems.md#smart-contracts) accordin
### Storage
- Addition
- Be aware that public constatns/immutables affect the `interfaceId`.
+ Be aware that public constants/immutables affect the `interfaceId`.
Implementation
- **IF** contract is **NOT** upgradeable
@@ -86,7 +86,7 @@ The reordering / removal of fields in structs being stored in dynamic arrays or
- not easily possible if the base class introduces storage and therefore needs a storage gap itself, which is likely the case
- new contract needs to inherit from the previous implementation
- - methods affected must be virtual to be overriden
+ - methods affected must be virtual to be overridden
- initialization gets convoluted
- the double initialization problem must be avoided
@@ -108,7 +108,7 @@ See the [OpenZeppelin article on multiple inheritance](https://docs.openzeppelin
- Change
- Body
- A change to a function body can change the behaviour of the function requiring dependent contracts or software systems to change
+ A change to a function body can change the behavior of the function requiring dependent contracts or software systems to change
- **IF** used by SDK
@@ -117,17 +117,17 @@ See the [OpenZeppelin article on multiple inheritance](https://docs.openzeppelin
- **IF** used by App
- [ ] adapt App
- Behavioural changes that can be **major** / breaking
+ Behavioral changes that can be **major** / breaking
- additional checks that result in external contracts reverting
- making different internal or external calls
- different execution or storing logic
- Behavioural changes that can be **minor**
+ Behavioral changes that can be **minor**
- storing new values
- Non-behavioural changes that classify as **patch**
+ Non-behavioral changes that classify as **patch**
- gas optimizations
@@ -212,7 +212,7 @@ Inheriting contracts or depending software systems must adapt.
Logic using the constants / immutable variables must be adapted
-Be aware that public constatns/immutables change the `interfaceId`.
+Be aware that public constants/immutables change the `interfaceId`.
### Compiler Version
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/00-abstract-contract.md b/packages/contracts/docs/framework-lifecycle/03-osx-components/00-abstract-contract.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/00-abstract-contract.md
rename to packages/contracts/docs/framework-lifecycle/03-osx-components/00-abstract-contract.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/01-factory-contract.md b/packages/contracts/docs/framework-lifecycle/03-osx-components/01-factory-contract.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/01-factory-contract.md
rename to packages/contracts/docs/framework-lifecycle/03-osx-components/01-factory-contract.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/02-upgradeable-contract.md b/packages/contracts/docs/framework-lifecycle/03-osx-components/02-upgradeable-contract.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/02-upgradeable-contract.md
rename to packages/contracts/docs/framework-lifecycle/03-osx-components/02-upgradeable-contract.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/03-non-upgradeable-contract.md b/packages/contracts/docs/framework-lifecycle/03-osx-components/03-non-upgradeable-contract.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/03-non-upgradeable-contract.md
rename to packages/contracts/docs/framework-lifecycle/03-osx-components/03-non-upgradeable-contract.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/04-registry-contract.md b/packages/contracts/docs/framework-lifecycle/03-osx-components/04-registry-contract.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/04-registry-contract.md
rename to packages/contracts/docs/framework-lifecycle/03-osx-components/04-registry-contract.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/05-plugin-setup-processor.md b/packages/contracts/docs/framework-lifecycle/03-osx-components/05-plugin-setup-processor.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/05-plugin-setup-processor.md
rename to packages/contracts/docs/framework-lifecycle/03-osx-components/05-plugin-setup-processor.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/06-plugin-build.md b/packages/contracts/docs/framework-lifecycle/03-osx-components/06-plugin-build.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/06-plugin-build.md
rename to packages/contracts/docs/framework-lifecycle/03-osx-components/06-plugin-build.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/07-plugin-setup.md b/packages/contracts/docs/framework-lifecycle/03-osx-components/07-plugin-setup.md
similarity index 95%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/07-plugin-setup.md
rename to packages/contracts/docs/framework-lifecycle/03-osx-components/07-plugin-setup.md
index f5059cc71..1581239fd 100644
--- a/packages/contracts/docs/developer-portal/04-framework-lifecycle/03-osx-components/07-plugin-setup.md
+++ b/packages/contracts/docs/framework-lifecycle/03-osx-components/07-plugin-setup.md
@@ -58,7 +58,7 @@ Conduct all necessary actions to prepare the installation of a plugin.
## Implement `prepareUninstallation`
-Conduct all necessary actions to prepare the uninstallation of a plugin and to decomission it.
+Conduct all necessary actions to prepare the uninstallation of a plugin and to decommission it.
- decode `_payload._data` if required
@@ -76,7 +76,7 @@ Conduct all necessary actions to prepare the uninstallation of a plugin and to d
over from the `_currentBuild` to the new build by
- - deploying / decomissioning helpers return the addresses of the prevailing ones via the `preparedSetupData.helpers` array of addresses.
+ - deploying / decommissioning helpers return the addresses of the prevailing ones via the `preparedSetupData.helpers` array of addresses.
- requesting to grant new / revoke existing permissions and returning them via the `preparedSetupData.permissions` array of permission structs.
## Update Metadata
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/01-protocol-version.md b/packages/contracts/docs/framework-lifecycle/04-sub-processes/01-protocol-version.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/01-protocol-version.md
rename to packages/contracts/docs/framework-lifecycle/04-sub-processes/01-protocol-version.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/02-contract-initialization.md b/packages/contracts/docs/framework-lifecycle/04-sub-processes/02-contract-initialization.md
similarity index 94%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/02-contract-initialization.md
rename to packages/contracts/docs/framework-lifecycle/04-sub-processes/02-contract-initialization.md
index ad82efbc9..12c90b31b 100644
--- a/packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/02-contract-initialization.md
+++ b/packages/contracts/docs/framework-lifecycle/04-sub-processes/02-contract-initialization.md
@@ -58,7 +58,7 @@ Accordingly, `v1.2.0` is the 5th version and our `reinitializer()` number is `5`
First, change the `reinitializer(5)` and make sure that there is a test for it.
-Then do all the initialzation changes and write a note, in which version they have been introduced (for easier traceability).
+Then do all the initialization changes and write a note, in which version they have been introduced (for easier traceability).
```solidity
function initialize(uint256 calldata _a, address calldata _c) external reinitializer(5) {
@@ -89,7 +89,7 @@ function initializeFrom(
uint8[3] calldata _previousProtocolVersion,
bytes calldata _initData
) external reinitializer(5) {
- // Ensure that the previous and current protocol MAJOR version numbers match. If not, revert because the upgrade is incompatible since breaking changes occured.
+ // Ensure that the previous and current protocol MAJOR version numbers match. If not, revert because the upgrade is incompatible since breaking changes occurred.
if (_previousProtocolVersion[0] != protocolVersion()[0]) {
revert('Incompatible upgrade');
}
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/03-testing.md b/packages/contracts/docs/framework-lifecycle/04-sub-processes/03-testing.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/03-testing.md
rename to packages/contracts/docs/framework-lifecycle/04-sub-processes/03-testing.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/04-documentation.md b/packages/contracts/docs/framework-lifecycle/04-sub-processes/04-documentation.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/04-documentation.md
rename to packages/contracts/docs/framework-lifecycle/04-sub-processes/04-documentation.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/05-deployment.md b/packages/contracts/docs/framework-lifecycle/04-sub-processes/05-deployment.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/05-deployment.md
rename to packages/contracts/docs/framework-lifecycle/04-sub-processes/05-deployment.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/06-aragon-update.md b/packages/contracts/docs/framework-lifecycle/04-sub-processes/06-aragon-update.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/04-sub-processes/06-aragon-update.md
rename to packages/contracts/docs/framework-lifecycle/04-sub-processes/06-aragon-update.md
diff --git a/packages/contracts/docs/developer-portal/04-framework-lifecycle/index.md b/packages/contracts/docs/framework-lifecycle/index.md
similarity index 100%
rename from packages/contracts/docs/developer-portal/04-framework-lifecycle/index.md
rename to packages/contracts/docs/framework-lifecycle/index.md