diff --git a/src/main/kotlin/g0001_0100/s0001_two_sum/readme.md b/src/main/kotlin/g0001_0100/s0001_two_sum/readme.md
new file mode 100644
index 000000000..f8ef247b7
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0001_two_sum/readme.md
@@ -0,0 +1,38 @@
+1\. Two Sum
+
+Easy
+
+Given an array of integers `nums` and an integer `target`, return _indices of the two numbers such that they add up to `target`_.
+
+You may assume that each input would have **_exactly_ one solution**, and you may not use the _same_ element twice.
+
+You can return the answer in any order.
+
+**Example 1:**
+
+**Input:** nums = [2,7,11,15], target = 9
+
+**Output:** [0,1]
+
+**Output:** Because nums[0] + nums[1] == 9, we return [0, 1].
+
+**Example 2:**
+
+**Input:** nums = [3,2,4], target = 6
+
+**Output:** [1,2]
+
+**Example 3:**
+
+**Input:** nums = [3,3], target = 6
+
+**Output:** [0,1]
+
+**Constraints:**
+
+* 2 <= nums.length <= 104
+* -109 <= nums[i] <= 109
+* -109 <= target <= 109
+* **Only one valid answer exists.**
+
+**Follow-up:** Can you come up with an algorithm that is less than O(n2)
time complexity?
\ No newline at end of file
diff --git a/src/main/kotlin/g0001_0100/s0002_add_two_numbers/readme.md b/src/main/kotlin/g0001_0100/s0002_add_two_numbers/readme.md
new file mode 100644
index 000000000..173c988e0
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0002_add_two_numbers/readme.md
@@ -0,0 +1,35 @@
+2\. Add Two Numbers
+
+Medium
+
+You are given two **non-empty** linked lists representing two non-negative integers. The digits are stored in **reverse order**, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
+
+You may assume the two numbers do not contain any leading zero, except the number 0 itself.
+
+**Example 1:**
+
+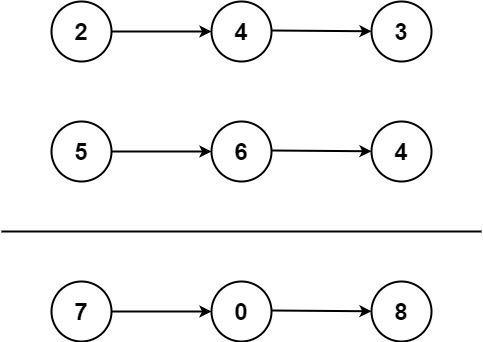
+
+**Input:** l1 = [2,4,3], l2 = [5,6,4]
+
+**Output:** [7,0,8]
+
+**Explanation:** 342 + 465 = 807.
+
+**Example 2:**
+
+**Input:** l1 = [0], l2 = [0]
+
+**Output:** [0]
+
+**Example 3:**
+
+**Input:** l1 = [9,9,9,9,9,9,9], l2 = [9,9,9,9]
+
+**Output:** [8,9,9,9,0,0,0,1]
+
+**Constraints:**
+
+* The number of nodes in each linked list is in the range `[1, 100]`.
+* `0 <= Node.val <= 9`
+* It is guaranteed that the list represents a number that does not have leading zeros.
\ No newline at end of file
diff --git a/src/main/kotlin/g0001_0100/s0003_longest_substring_without_repeating_characters/readme.md b/src/main/kotlin/g0001_0100/s0003_longest_substring_without_repeating_characters/readme.md
new file mode 100644
index 000000000..8b44615cd
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0003_longest_substring_without_repeating_characters/readme.md
@@ -0,0 +1,40 @@
+3\. Longest Substring Without Repeating Characters
+
+Medium
+
+Given a string `s`, find the length of the **longest substring** without repeating characters.
+
+**Example 1:**
+
+**Input:** s = "abcabcbb"
+
+**Output:** 3
+
+**Explanation:** The answer is "abc", with the length of 3.
+
+**Example 2:**
+
+**Input:** s = "bbbbb"
+
+**Output:** 1
+
+**Explanation:** The answer is "b", with the length of 1.
+
+**Example 3:**
+
+**Input:** s = "pwwkew"
+
+**Output:** 3
+
+**Explanation:** The answer is "wke", with the length of 3. Notice that the answer must be a substring, "pwke" is a subsequence and not a substring.
+
+**Example 4:**
+
+**Input:** s = ""
+
+**Output:** 0
+
+**Constraints:**
+
+* 0 <= s.length <= 5 * 104
+* `s` consists of English letters, digits, symbols and spaces.
\ No newline at end of file
diff --git a/src/main/kotlin/g0001_0100/s0004_median_of_two_sorted_arrays/readme.md b/src/main/kotlin/g0001_0100/s0004_median_of_two_sorted_arrays/readme.md
new file mode 100644
index 000000000..18d9ada0f
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0004_median_of_two_sorted_arrays/readme.md
@@ -0,0 +1,50 @@
+4\. Median of Two Sorted Arrays
+
+Hard
+
+Given two sorted arrays `nums1` and `nums2` of size `m` and `n` respectively, return **the median** of the two sorted arrays.
+
+The overall run time complexity should be `O(log (m+n))`.
+
+**Example 1:**
+
+**Input:** nums1 = [1,3], nums2 = [2]
+
+**Output:** 2.00000
+
+**Explanation:** merged array = [1,2,3] and median is 2.
+
+**Example 2:**
+
+**Input:** nums1 = [1,2], nums2 = [3,4]
+
+**Output:** 2.50000
+
+**Explanation:** merged array = [1,2,3,4] and median is (2 + 3) / 2 = 2.5.
+
+**Example 3:**
+
+**Input:** nums1 = [0,0], nums2 = [0,0]
+
+**Output:** 0.00000
+
+**Example 4:**
+
+**Input:** nums1 = [], nums2 = [1]
+
+**Output:** 1.00000
+
+**Example 5:**
+
+**Input:** nums1 = [2], nums2 = []
+
+**Output:** 2.00000
+
+**Constraints:**
+
+* `nums1.length == m`
+* `nums2.length == n`
+* `0 <= m <= 1000`
+* `0 <= n <= 1000`
+* `1 <= m + n <= 2000`
+* -106 <= nums1[i], nums2[i] <= 106
\ No newline at end of file
diff --git a/src/main/kotlin/g0001_0100/s0005_longest_palindromic_substring/readme.md b/src/main/kotlin/g0001_0100/s0005_longest_palindromic_substring/readme.md
new file mode 100644
index 000000000..a481f2790
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0005_longest_palindromic_substring/readme.md
@@ -0,0 +1,34 @@
+5\. Longest Palindromic Substring
+
+Medium
+
+Given a string `s`, return _the longest palindromic substring_ in `s`.
+
+**Example 1:**
+
+**Input:** s = "babad"
+
+**Output:** "bab" **Note:** "aba" is also a valid answer.
+
+**Example 2:**
+
+**Input:** s = "cbbd"
+
+**Output:** "bb"
+
+**Example 3:**
+
+**Input:** s = "a"
+
+**Output:** "a"
+
+**Example 4:**
+
+**Input:** s = "ac"
+
+**Output:** "a"
+
+**Constraints:**
+
+* `1 <= s.length <= 1000`
+* `s` consist of only digits and English letters.
\ No newline at end of file
diff --git a/src/main/kotlin/g0001_0100/s0006_zigzag_conversion/readme.md b/src/main/kotlin/g0001_0100/s0006_zigzag_conversion/readme.md
new file mode 100644
index 000000000..afa33c203
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0006_zigzag_conversion/readme.md
@@ -0,0 +1,39 @@
+6\. Zigzag Conversion
+
+Medium
+
+The string `"PAYPALISHIRING"` is written in a zigzag pattern on a given number of rows like this: (you may want to display this pattern in a fixed font for better legibility)
+
+P A H N A P L S I I G Y I R
+
+And then read line by line: `"PAHNAPLSIIGYIR"`
+
+Write the code that will take a string and make this conversion given a number of rows:
+
+string convert(string s, int numRows);
+
+**Example 1:**
+
+**Input:** s = "PAYPALISHIRING", numRows = 3
+
+**Output:** "PAHNAPLSIIGYIR"
+
+**Example 2:**
+
+**Input:** s = "PAYPALISHIRING", numRows = 4
+
+**Output:** "PINALSIGYAHRPI"
+
+**Explanation:** P I N A L S I G Y A H R P I
+
+**Example 3:**
+
+**Input:** s = "A", numRows = 1
+
+**Output:** "A"
+
+**Constraints:**
+
+* `1 <= s.length <= 1000`
+* `s` consists of English letters (lower-case and upper-case), `','` and `'.'`.
+* `1 <= numRows <= 1000`
\ No newline at end of file
diff --git a/src/main/kotlin/g0001_0100/s0007_reverse_integer/readme.md b/src/main/kotlin/g0001_0100/s0007_reverse_integer/readme.md
new file mode 100644
index 000000000..42257ea9b
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0007_reverse_integer/readme.md
@@ -0,0 +1,35 @@
+7\. Reverse Integer
+
+Medium
+
+Given a signed 32-bit integer `x`, return `x` _with its digits reversed_. If reversing `x` causes the value to go outside the signed 32-bit integer range [-231, 231 - 1]
, then return `0`.
+
+**Assume the environment does not allow you to store 64-bit integers (signed or unsigned).**
+
+**Example 1:**
+
+**Input:** x = 123
+
+**Output:** 321
+
+**Example 2:**
+
+**Input:** x = -123
+
+**Output:** -321
+
+**Example 3:**
+
+**Input:** x = 120
+
+**Output:** 21
+
+**Example 4:**
+
+**Input:** x = 0
+
+**Output:** 0
+
+**Constraints:**
+
+* -231 <= x <= 231 - 1
\ No newline at end of file
diff --git a/src/main/kotlin/g0001_0100/s0008_string_to_integer_atoi/readme.md b/src/main/kotlin/g0001_0100/s0008_string_to_integer_atoi/readme.md
new file mode 100644
index 000000000..43dd87fc0
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0008_string_to_integer_atoi/readme.md
@@ -0,0 +1,113 @@
+8\. String to Integer (atoi)
+
+Medium
+
+Implement the `myAtoi(string s)` function, which converts a string to a 32-bit signed integer (similar to C/C++'s `atoi` function).
+
+The algorithm for `myAtoi(string s)` is as follows:
+
+1. Read in and ignore any leading whitespace.
+2. Check if the next character (if not already at the end of the string) is `'-'` or `'+'`. Read this character in if it is either. This determines if the final result is negative or positive respectively. Assume the result is positive if neither is present.
+3. Read in next the characters until the next non-digit character or the end of the input is reached. The rest of the string is ignored.
+4. Convert these digits into an integer (i.e. `"123" -> 123`, `"0032" -> 32`). If no digits were read, then the integer is `0`. Change the sign as necessary (from step 2).
+5. If the integer is out of the 32-bit signed integer range [-231, 231 - 1]
, then clamp the integer so that it remains in the range. Specifically, integers less than -231
should be clamped to -231
, and integers greater than 231 - 1
should be clamped to 231 - 1
.
+6. Return the integer as the final result.
+
+**Note:**
+
+* Only the space character `' '` is considered a whitespace character.
+* **Do not ignore** any characters other than the leading whitespace or the rest of the string after the digits.
+
+**Example 1:**
+
+**Input:** s = "42"
+
+**Output:** 42
+
+**Explanation:** The underlined characters are what is read in, the caret is the current reader position.
+
+ Step 1: "42" (no characters read because there is no leading whitespace)
+ ^
+ Step 2: "42" (no characters read because there is neither a '-' nor '+')
+ ^
+ Step 3: "42" ("42" is read in)
+ ^
+
+The parsed integer is 42. Since 42 is in the range [-231, 231 - 1], the final result is 42.
+
+**Example 2:**
+
+**Input:** s = " -42"
+
+**Output:** -42
+
+**Explanation:**
+
+ Step 1: " -42" (leading whitespace is read and ignored)
+ ^
+ Step 2: " -42" ('-' is read, so the result should be negative)
+ ^
+ Step 3: " -42" ("42" is read in)
+ ^
+ The parsed integer is -42.
+
+Since -42 is in the range [-231, 231 - 1], the final result is -42.
+
+**Example 3:**
+
+**Input:** s = "4193 with words"
+
+**Output:** 4193
+
+**Explanation:**
+
+ Step 1: "4193 with words" (no characters read because there is no leading whitespace)
+ ^
+ Step 2: "4193 with words" (no characters read because there is neither a '-' nor '+')
+ ^
+ Step 3: "4193 with words" ("4193" is read in; reading stops because the next character is a non-digit)
+ ^
+ The parsed integer is 4193.
+
+Since 4193 is in the range [-231, 231 - 1], the final result is 4193.
+
+**Example 4:**
+
+**Input:** s = "words and 987"
+
+**Output:** 0
+
+**Explanation:**
+
+ Step 1: "words and 987" (no characters read because there is no leading whitespace)
+ ^
+ Step 2: "words and 987" (no characters read because there is neither a '-' nor '+')
+ ^
+ Step 3: "words and 987" (reading stops immediately because there is a non-digit 'w')
+ ^
+ The parsed integer is 0 because no digits were read.
+
+Since 0 is in the range [-231, 231 - 1], the final result is 0.
+
+**Example 5:**
+
+**Input:** s = "-91283472332"
+
+**Output:** -2147483648
+
+**Explanation:**
+
+ Step 1: "-91283472332" (no characters read because there is no leading whitespace)
+ ^
+ Step 2: "-91283472332" ('-' is read, so the result should be negative)
+ ^
+ Step 3: "-91283472332" ("91283472332" is read in)
+ ^
+ The parsed integer is -91283472332.
+
+Since -91283472332 is less than the lower bound of the range [-231, 231 - 1], the final result is clamped to -231 = -2147483648.
+
+**Constraints:**
+
+* `0 <= s.length <= 200`
+* `s` consists of English letters (lower-case and upper-case), digits (`0-9`), `' '`, `'+'`, `'-'`, and `'.'`.
\ No newline at end of file
diff --git a/src/main/kotlin/g0001_0100/s0009_palindrome_number/readme.md b/src/main/kotlin/g0001_0100/s0009_palindrome_number/readme.md
new file mode 100644
index 000000000..f45588bb0
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0009_palindrome_number/readme.md
@@ -0,0 +1,41 @@
+9\. Palindrome Number
+
+Easy
+
+Given an integer `x`, return `true` if `x` is palindrome integer.
+
+An integer is a **palindrome** when it reads the same backward as forward. For example, `121` is palindrome while `123` is not.
+
+**Example 1:**
+
+**Input:** x = 121
+
+**Output:** true
+
+**Example 2:**
+
+**Input:** x = -121
+
+**Output:** false
+
+**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
+
+**Example 3:**
+
+**Input:** x = 10
+
+**Output:** false
+
+**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
+
+**Example 4:**
+
+**Input:** x = -101
+
+**Output:** false
+
+**Constraints:**
+
+* -231 <= x <= 231 - 1
+
+**Follow up:** Could you solve it without converting the integer to a string?
\ No newline at end of file
diff --git a/src/main/kotlin/g0001_0100/s0010_regular_expression_matching/readme.md b/src/main/kotlin/g0001_0100/s0010_regular_expression_matching/readme.md
new file mode 100644
index 000000000..88edfba85
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0010_regular_expression_matching/readme.md
@@ -0,0 +1,56 @@
+10\. Regular Expression Matching
+
+Hard
+
+Given an input string `s` and a pattern `p`, implement regular expression matching with support for `'.'` and `'*'` where:
+
+* `'.'` Matches any single character.
+* `'*'` Matches zero or more of the preceding element.
+
+The matching should cover the **entire** input string (not partial).
+
+**Example 1:**
+
+**Input:** s = "aa", p = "a"
+
+**Output:** false
+
+**Explanation:** "a" does not match the entire string "aa".
+
+**Example 2:**
+
+**Input:** s = "aa", p = "a\*"
+
+**Output:** true
+
+**Explanation:** '\*' means zero or more of the preceding element, 'a'. Therefore, by repeating 'a' once, it becomes "aa".
+
+**Example 3:**
+
+**Input:** s = "ab", p = ".\*"
+
+**Output:** true
+
+**Explanation:** ".\*" means "zero or more (\*) of any character (.)".
+
+**Example 4:**
+
+**Input:** s = "aab", p = "c\*a\*b"
+
+**Output:** true
+
+**Explanation:** c can be repeated 0 times, a can be repeated 1 time. Therefore, it matches "aab".
+
+**Example 5:**
+
+**Input:** s = "mississippi", p = "mis\*is\*p\*."
+
+**Output:** false
+
+**Constraints:**
+
+* `1 <= s.length <= 20`
+* `1 <= p.length <= 30`
+* `s` contains only lowercase English letters.
+* `p` contains only lowercase English letters, `'.'`, and `'*'`.
+* It is guaranteed for each appearance of the character `'*'`, there will be a previous valid character to match.
\ No newline at end of file
diff --git a/src/main/kotlin/g0001_0100/s0011_container_with_most_water/readme.md b/src/main/kotlin/g0001_0100/s0011_container_with_most_water/readme.md
new file mode 100644
index 000000000..56788449e
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0011_container_with_most_water/readme.md
@@ -0,0 +1,41 @@
+11\. Container With Most Water
+
+Medium
+
+Given `n` non-negative integers a1, a2, ..., an
, where each represents a point at coordinate (i, ai)
. `n` vertical lines are drawn such that the two endpoints of the line `i` is at (i, ai)
and `(i, 0)`. Find two lines, which, together with the x-axis forms a container, such that the container contains the most water.
+
+**Notice** that you may not slant the container.
+
+**Example 1:**
+
+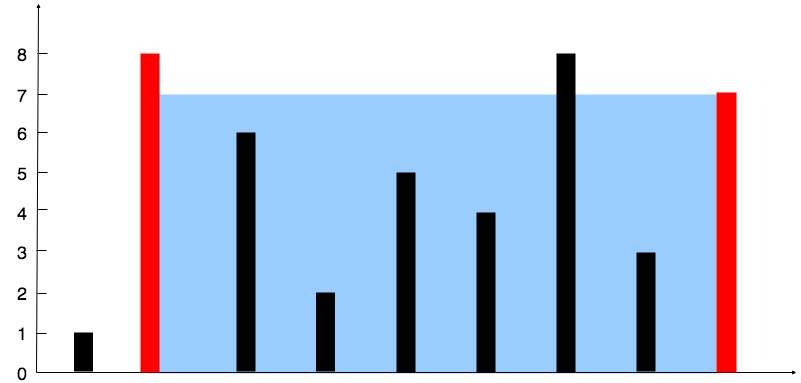
+
+**Input:** height = [1,8,6,2,5,4,8,3,7]
+
+**Output:** 49
+
+**Explanation:** The above vertical lines are represented by array [1,8,6,2,5,4,8,3,7]. In this case, the max area of water (blue section) the container can contain is 49.
+
+**Example 2:**
+
+**Input:** height = [1,1]
+
+**Output:** 1
+
+**Example 3:**
+
+**Input:** height = [4,3,2,1,4]
+
+**Output:** 16
+
+**Example 4:**
+
+**Input:** height = [1,2,1]
+
+**Output:** 2
+
+**Constraints:**
+
+* `n == height.length`
+* 2 <= n <= 105
+* 0 <= height[i] <= 104
\ No newline at end of file
diff --git a/src/main/kotlin/g0001_0100/s0012_integer_to_roman/readme.md b/src/main/kotlin/g0001_0100/s0012_integer_to_roman/readme.md
new file mode 100644
index 000000000..0470e12f6
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0012_integer_to_roman/readme.md
@@ -0,0 +1,62 @@
+12\. Integer to Roman
+
+Medium
+
+Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
+
+ Symbol Value
+ I 1
+ V 5
+ X 10
+ L 50
+ C 100
+ D 500
+ M 1000
+
+For example, `2` is written as `II` in Roman numeral, just two one's added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
+
+Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
+
+* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
+* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
+* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
+
+Given an integer, convert it to a roman numeral.
+
+**Example 1:**
+
+**Input:** num = 3
+
+**Output:** "III"
+
+**Example 2:**
+
+**Input:** num = 4
+
+**Output:** "IV"
+
+**Example 3:**
+
+**Input:** num = 9
+
+**Output:** "IX"
+
+**Example 4:**
+
+**Input:** num = 58
+
+**Output:** "LVIII"
+
+**Explanation:** L = 50, V = 5, III = 3.
+
+**Example 5:**
+
+**Input:** num = 1994
+
+**Output:** "MCMXCIV"
+
+**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
+
+**Constraints:**
+
+* `1 <= num <= 3999`
diff --git a/src/main/kotlin/g0001_0100/s0013_roman_to_integer/readme.md b/src/main/kotlin/g0001_0100/s0013_roman_to_integer/readme.md
new file mode 100644
index 000000000..60c47f12c
--- /dev/null
+++ b/src/main/kotlin/g0001_0100/s0013_roman_to_integer/readme.md
@@ -0,0 +1,64 @@
+13\. Roman to Integer
+
+Easy
+
+Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
+
+ Symbol Value
+ I 1
+ V 5
+ X 10
+ L 50
+ C 100
+ D 500
+ M 1000
+
+For example, `2` is written as `II` in Roman numeral, just two one's added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
+
+Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
+
+* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
+* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
+* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
+
+Given a roman numeral, convert it to an integer.
+
+**Example 1:**
+
+**Input:** s = "III"
+
+**Output:** 3
+
+**Example 2:**
+
+**Input:** s = "IV"
+
+**Output:** 4
+
+**Example 3:**
+
+**Input:** s = "IX"
+
+**Output:** 9
+
+**Example 4:**
+
+**Input:** s = "LVIII"
+
+**Output:** 58
+
+**Explanation:** L = 50, V= 5, III = 3.
+
+**Example 5:**
+
+**Input:** s = "MCMXCIV"
+
+**Output:** 1994
+
+**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
+
+**Constraints:**
+
+* `1 <= s.length <= 15`
+* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
+* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`.
diff --git a/src/main/kotlin/g0801_0900/s0864_shortest_path_to_get_all_keys/readme.md b/src/main/kotlin/g0801_0900/s0864_shortest_path_to_get_all_keys/readme.md
new file mode 100644
index 000000000..1ca2d8be9
--- /dev/null
+++ b/src/main/kotlin/g0801_0900/s0864_shortest_path_to_get_all_keys/readme.md
@@ -0,0 +1,55 @@
+864\. Shortest Path to Get All Keys
+
+Hard
+
+You are given an `m x n` grid `grid` where:
+
+* `'.'` is an empty cell.
+* `'#'` is a wall.
+* `'@'` is the starting point.
+* Lowercase letters represent keys.
+* Uppercase letters represent locks.
+
+You start at the starting point and one move consists of walking one space in one of the four cardinal directions. You cannot walk outside the grid, or walk into a wall.
+
+If you walk over a key, you can pick it up and you cannot walk over a lock unless you have its corresponding key.
+
+For some `1 <= k <= 6`, there is exactly one lowercase and one uppercase letter of the first `k` letters of the English alphabet in the grid. This means that there is exactly one key for each lock, and one lock for each key; and also that the letters used to represent the keys and locks were chosen in the same order as the English alphabet.
+
+Return _the lowest number of moves to acquire all keys_. If it is impossible, return `-1`.
+
+**Example 1:**
+
+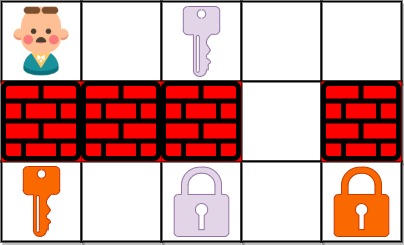
+
+**Input:** grid = ["@.a.#","###.#","b.A.B"]
+
+**Output:** 8
+
+**Explanation:** Note that the goal is to obtain all the keys not to open all the locks.
+
+**Example 2:**
+
+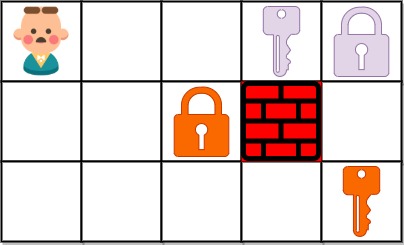
+
+**Input:** grid = ["@..aA","..B#.","....b"]
+
+**Output:** 6
+
+**Example 3:**
+
+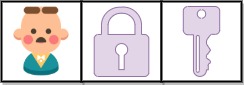
+
+**Input:** grid = ["@Aa"]
+
+**Output:** -1
+
+**Constraints:**
+
+* `m == grid.length`
+* `n == grid[i].length`
+* `1 <= m, n <= 30`
+* `grid[i][j]` is either an English letter, `'.'`, `'#'`, or `'@'`.
+* The number of keys in the grid is in the range `[1, 6]`.
+* Each key in the grid is **unique**.
+* Each key in the grid has a matching lock.
\ No newline at end of file