diff --git a/LeetCodeNet.Tests/G0101_0200/S0152_maximum_product_subarray/SolutionTest.cs b/LeetCodeNet.Tests/G0101_0200/S0152_maximum_product_subarray/SolutionTest.cs
new file mode 100644
index 0000000..746014b
--- /dev/null
+++ b/LeetCodeNet.Tests/G0101_0200/S0152_maximum_product_subarray/SolutionTest.cs
@@ -0,0 +1,16 @@
+namespace LeetCodeNet.G0101_0200.S0152_maximum_product_subarray {
+
+using Xunit;
+
+public class SolutionTest {
+ [Fact]
+ public void MaxProduct() {
+ Assert.Equal(6, new Solution().MaxProduct(new int[] {2, 3, -2, 4}));
+ }
+
+ [Fact]
+ public void MaxProduct2() {
+ Assert.Equal(0, new Solution().MaxProduct(new int[] {-2, 0, -1}));
+ }
+}
+}
diff --git a/LeetCodeNet.Tests/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/SolutionTest.cs b/LeetCodeNet.Tests/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/SolutionTest.cs
new file mode 100644
index 0000000..97a4c51
--- /dev/null
+++ b/LeetCodeNet.Tests/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/SolutionTest.cs
@@ -0,0 +1,21 @@
+namespace LeetCodeNet.G0101_0200.S0153_find_minimum_in_rotated_sorted_array {
+
+using Xunit;
+
+public class SolutionTest {
+ [Fact]
+ public void FindMin() {
+ Assert.Equal(1, new Solution().FindMin(new int[] {3, 4, 5, 1, 2}));
+ }
+
+ [Fact]
+ public void FindMin2() {
+ Assert.Equal(0, new Solution().FindMin(new int[] {4, 5, 6, 7, 0, 1, 2}));
+ }
+
+ [Fact]
+ public void FindMin3() {
+ Assert.Equal(11, new Solution().FindMin(new int[] {11, 13, 15, 17}));
+ }
+}
+}
diff --git a/LeetCodeNet.Tests/G0101_0200/S0155_min_stack/MinStackTest.cs b/LeetCodeNet.Tests/G0101_0200/S0155_min_stack/MinStackTest.cs
new file mode 100644
index 0000000..1b72a85
--- /dev/null
+++ b/LeetCodeNet.Tests/G0101_0200/S0155_min_stack/MinStackTest.cs
@@ -0,0 +1,21 @@
+namespace LeetCodeNet.G0101_0200.S0155_min_stack {
+
+using Xunit;
+
+public class MinStackTest {
+ [Fact]
+ public void MinStack() {
+ MinStack minStack = new MinStack();
+ minStack.Push(-2);
+ minStack.Push(0);
+ minStack.Push(-3);
+ // return -3
+ Assert.Equal(-3, minStack.GetMin());
+ minStack.Pop();
+ // return 0
+ Assert.Equal(0, minStack.Top());
+ // return -2
+ Assert.Equal(-2, minStack.GetMin());
+ }
+}
+}
diff --git a/LeetCodeNet.Tests/G0101_0200/S0160_intersection_of_two_linked_lists/SolutionTest.cs b/LeetCodeNet.Tests/G0101_0200/S0160_intersection_of_two_linked_lists/SolutionTest.cs
new file mode 100644
index 0000000..b547040
--- /dev/null
+++ b/LeetCodeNet.Tests/G0101_0200/S0160_intersection_of_two_linked_lists/SolutionTest.cs
@@ -0,0 +1,22 @@
+namespace LeetCodeNet.G0101_0200.S0160_intersection_of_two_linked_lists {
+
+using Xunit;
+using LeetCodeNet.Com_github_leetcode;
+
+public class SolutionTest {
+ [Fact]
+ public void GetIntersectionNode() {
+ ListNode intersectionListNode = new ListNode(8, new ListNode(4, new ListNode(5)));
+ ListNode nodeA = new ListNode(4, new ListNode(1, intersectionListNode));
+ ListNode nodeB = new ListNode(5, new ListNode(6, new ListNode(1, intersectionListNode)));
+ Assert.Equal(8, new Solution().GetIntersectionNode(nodeA, nodeB).val);
+ }
+
+ [Fact]
+ public void GetIntersectionNode2() {
+ ListNode nodeA = new ListNode(4, new ListNode(1, new ListNode(2)));
+ ListNode nodeB = new ListNode(5, new ListNode(6, new ListNode(1, new ListNode(2))));
+ Assert.Equal(null, new Solution().GetIntersectionNode(nodeA, nodeB));
+ }
+}
+}
diff --git a/LeetCodeNet.Tests/G0101_0200/S0169_majority_element/SolutionTest.cs b/LeetCodeNet.Tests/G0101_0200/S0169_majority_element/SolutionTest.cs
new file mode 100644
index 0000000..51182de
--- /dev/null
+++ b/LeetCodeNet.Tests/G0101_0200/S0169_majority_element/SolutionTest.cs
@@ -0,0 +1,16 @@
+namespace LeetCodeNet.G0101_0200.S0169_majority_element {
+
+using Xunit;
+
+public class SolutionTest {
+ [Fact]
+ public void MajorityElement() {
+ Assert.Equal(3, new Solution().MajorityElement(new int[] {3, 2, 3}));
+ }
+
+ [Fact]
+ public void MajorityElement2() {
+ Assert.Equal(2, new Solution().MajorityElement(new int[] {2, 2, 1, 1, 1, 2, 2}));
+ }
+}
+}
diff --git a/LeetCodeNet.Tests/G0101_0200/S0189_rotate_array/SolutionTest.cs b/LeetCodeNet.Tests/G0101_0200/S0189_rotate_array/SolutionTest.cs
new file mode 100644
index 0000000..e80e04c
--- /dev/null
+++ b/LeetCodeNet.Tests/G0101_0200/S0189_rotate_array/SolutionTest.cs
@@ -0,0 +1,20 @@
+namespace LeetCodeNet.G0101_0200.S0189_rotate_array {
+
+using Xunit;
+
+public class SolutionTest {
+ [Fact]
+ public void Rotate() {
+ int[] array = new int[] {1, 2, 3, 4, 5, 6, 7};
+ new Solution().Rotate(array, 3);
+ Assert.Equal(new int[] {5, 6, 7, 1, 2, 3, 4}, array);
+ }
+
+ [Fact]
+ public void Rotate2() {
+ int[] array = new int[] {-1, -100, 3, 99};
+ new Solution().Rotate(array, 2);
+ Assert.Equal(new int[] {3, 99, -1, -100}, array);
+ }
+}
+}
diff --git a/LeetCodeNet.Tests/G0101_0200/S0198_house_robber/SolutionTest.cs b/LeetCodeNet.Tests/G0101_0200/S0198_house_robber/SolutionTest.cs
new file mode 100644
index 0000000..23ef2b0
--- /dev/null
+++ b/LeetCodeNet.Tests/G0101_0200/S0198_house_robber/SolutionTest.cs
@@ -0,0 +1,16 @@
+namespace LeetCodeNet.G0101_0200.S0198_house_robber {
+
+using Xunit;
+
+public class SolutionTest {
+ [Fact]
+ public void Rob() {
+ Assert.Equal(4, new Solution().Rob(new int[] {1, 2, 3, 1}));
+ }
+
+ [Fact]
+ public void Rob2() {
+ Assert.Equal(12, new Solution().Rob(new int[] {2, 7, 9, 3, 1}));
+ }
+}
+}
diff --git a/LeetCodeNet.Tests/G0101_0200/S0200_number_of_islands/SolutionTest.cs b/LeetCodeNet.Tests/G0101_0200/S0200_number_of_islands/SolutionTest.cs
new file mode 100644
index 0000000..91d4c65
--- /dev/null
+++ b/LeetCodeNet.Tests/G0101_0200/S0200_number_of_islands/SolutionTest.cs
@@ -0,0 +1,28 @@
+namespace LeetCodeNet.G0101_0200.S0200_number_of_islands {
+
+using Xunit;
+
+public class SolutionTest {
+ [Fact]
+ public void NumIslands() {
+ char[][] grid = new char[][] {
+ new char[] {'1', '1', '1', '1', '0'},
+ new char[] {'1', '1', '0', '1', '0'},
+ new char[] {'1', '1', '0', '0', '0'},
+ new char[] {'0', '0', '0', '0', '0'}
+ };
+ Assert.Equal(1, new Solution().NumIslands(grid));
+ }
+
+ [Fact]
+ public void NumIslands2() {
+ char[][] grid = new char[][] {
+ new char[] {'1', '1', '0', '0', '0'},
+ new char[] {'1', '1', '0', '0', '0'},
+ new char[] {'0', '0', '1', '0', '0'},
+ new char[] {'0', '0', '0', '1', '1'}
+ };
+ Assert.Equal(3, new Solution().NumIslands(grid));
+ }
+}
+}
diff --git a/LeetCodeNet/G0101_0200/S0152_maximum_product_subarray/Solution.cs b/LeetCodeNet/G0101_0200/S0152_maximum_product_subarray/Solution.cs
new file mode 100644
index 0000000..c7d4774
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0152_maximum_product_subarray/Solution.cs
@@ -0,0 +1,29 @@
+namespace LeetCodeNet.G0101_0200.S0152_maximum_product_subarray {
+
+// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Dynamic_Programming
+// #Dynamic_Programming_I_Day_6 #Level_2_Day_13_Dynamic_Programming #Udemy_Dynamic_Programming
+// #Big_O_Time_O(N)_Space_O(1) #2024_01_11_Time_71_ms_(90.35%)_Space_42.7_MB_(13.88%)
+
+public class Solution {
+ public int MaxProduct(int[] nums) {
+ int ans = int.MinValue;
+ int cprod = 1;
+ foreach (int j in nums) {
+ cprod = cprod * j;
+ ans = Math.Max(ans, cprod);
+ if (cprod == 0) {
+ cprod = 1;
+ }
+ }
+ cprod = 1;
+ for (int i = nums.Length - 1; i >= 0; i--) {
+ cprod = cprod * nums[i];
+ ans = Math.Max(ans, cprod);
+ if (cprod == 0) {
+ cprod = 1;
+ }
+ }
+ return ans;
+ }
+}
+}
diff --git a/LeetCodeNet/G0101_0200/S0152_maximum_product_subarray/readme.md b/LeetCodeNet/G0101_0200/S0152_maximum_product_subarray/readme.md
new file mode 100644
index 0000000..13f4e95
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0152_maximum_product_subarray/readme.md
@@ -0,0 +1,31 @@
+152\. Maximum Product Subarray
+
+Medium
+
+Given an integer array `nums`, find a contiguous non-empty subarray within the array that has the largest product, and return _the product_.
+
+It is **guaranteed** that the answer will fit in a **32-bit** integer.
+
+A **subarray** is a contiguous subsequence of the array.
+
+**Example 1:**
+
+**Input:** nums = [2,3,-2,4]
+
+**Output:** 6
+
+**Explanation:** [2,3] has the largest product 6.
+
+**Example 2:**
+
+**Input:** nums = [-2,0,-1]
+
+**Output:** 0
+
+**Explanation:** The result cannot be 2, because [-2,-1] is not a subarray.
+
+**Constraints:**
+
+* 1 <= nums.length <= 2 * 104
+* `-10 <= nums[i] <= 10`
+* The product of any prefix or suffix of `nums` is **guaranteed** to fit in a **32-bit** integer.
\ No newline at end of file
diff --git a/LeetCodeNet/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/Solution.cs b/LeetCodeNet/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/Solution.cs
new file mode 100644
index 0000000..a71c2a8
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/Solution.cs
@@ -0,0 +1,33 @@
+namespace LeetCodeNet.G0101_0200.S0153_find_minimum_in_rotated_sorted_array {
+
+// #Medium #Top_100_Liked_Questions #Array #Binary_Search #Algorithm_II_Day_2_Binary_Search
+// #Binary_Search_I_Day_12 #Udemy_Binary_Search #Big_O_Time_O(log_N)_Space_O(log_N)
+// #2024_01_11_Time_64_ms_(88.59%)_Space_40.9_MB_(17.68%)
+
+public class Solution {
+ private int FindMinUtil(int[] nums, int l, int r) {
+ if (l == r) {
+ return nums[l];
+ }
+ int mid = (l + r) / 2;
+ if (mid == l && nums[mid] < nums[r]) {
+ return nums[l];
+ }
+ if (mid - 1 >= 0 && nums[mid - 1] > nums[mid]) {
+ return nums[mid];
+ }
+ if (nums[mid] < nums[l]) {
+ return FindMinUtil(nums, l, mid - 1);
+ } else if (nums[mid] > nums[r]) {
+ return FindMinUtil(nums, mid + 1, r);
+ }
+ return FindMinUtil(nums, l, mid - 1);
+ }
+
+ public int FindMin(int[] nums) {
+ int l = 0;
+ int r = nums.Length - 1;
+ return FindMinUtil(nums, l, r);
+ }
+}
+}
diff --git a/LeetCodeNet/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/readme.md b/LeetCodeNet/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/readme.md
new file mode 100644
index 0000000..db0231d
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/readme.md
@@ -0,0 +1,46 @@
+153\. Find Minimum in Rotated Sorted Array
+
+Medium
+
+Suppose an array of length `n` sorted in ascending order is **rotated** between `1` and `n` times. For example, the array `nums = [0,1,2,4,5,6,7]` might become:
+
+* `[4,5,6,7,0,1,2]` if it was rotated `4` times.
+* `[0,1,2,4,5,6,7]` if it was rotated `7` times.
+
+Notice that **rotating** an array `[a[0], a[1], a[2], ..., a[n-1]]` 1 time results in the array `[a[n-1], a[0], a[1], a[2], ..., a[n-2]]`.
+
+Given the sorted rotated array `nums` of **unique** elements, return _the minimum element of this array_.
+
+You must write an algorithm that runs in `O(log n) time.`
+
+**Example 1:**
+
+**Input:** nums = [3,4,5,1,2]
+
+**Output:** 1
+
+**Explanation:** The original array was [1,2,3,4,5] rotated 3 times.
+
+**Example 2:**
+
+**Input:** nums = [4,5,6,7,0,1,2]
+
+**Output:** 0
+
+**Explanation:** The original array was [0,1,2,4,5,6,7] and it was rotated 4 times.
+
+**Example 3:**
+
+**Input:** nums = [11,13,15,17]
+
+**Output:** 11
+
+**Explanation:** The original array was [11,13,15,17] and it was rotated 4 times.
+
+**Constraints:**
+
+* `n == nums.length`
+* `1 <= n <= 5000`
+* `-5000 <= nums[i] <= 5000`
+* All the integers of `nums` are **unique**.
+* `nums` is sorted and rotated between `1` and `n` times.
\ No newline at end of file
diff --git a/LeetCodeNet/G0101_0200/S0155_min_stack/MinStack.cs b/LeetCodeNet/G0101_0200/S0155_min_stack/MinStack.cs
new file mode 100644
index 0000000..06fe7d5
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0155_min_stack/MinStack.cs
@@ -0,0 +1,59 @@
+namespace LeetCodeNet.G0101_0200.S0155_min_stack {
+
+// #Easy #Top_100_Liked_Questions #Top_Interview_Questions #Stack #Design
+// #Data_Structure_II_Day_14_Stack_Queue #Programming_Skills_II_Day_18 #Level_2_Day_16_Design
+// #Udemy_Design #Big_O_Time_O(1)_Space_O(N)
+// #2024_01_11_Time_105_ms_(95.77%)_Space_55.6_MB_(13.78%)
+
+public class MinStack {
+ private class Node {
+ public int min;
+ public int data;
+ public Node nextNode;
+ public Node previousNode;
+
+ public Node(int min, int data, Node previousNode, Node nextNode) {
+ this.min = min;
+ this.data = data;
+ this.previousNode = previousNode;
+ this.nextNode = nextNode;
+ }
+ }
+
+ private Node currentNode;
+
+ // initialize your data structure here.
+ public MinStack() {
+ // no initialization needed.
+ }
+
+ public void Push(int val) {
+ if (currentNode == null) {
+ currentNode = new Node(val, val, null, null);
+ } else {
+ currentNode.nextNode = new Node(Math.Min(currentNode.min, val), val, currentNode, null);
+ currentNode = currentNode.nextNode;
+ }
+ }
+
+ public void Pop() {
+ currentNode = currentNode.previousNode;
+ }
+
+ public int Top() {
+ return currentNode.data;
+ }
+
+ public int GetMin() {
+ return currentNode.min;
+ }
+}
+}
+/**
+ * Your MinStack object will be instantiated and called as such:
+ * MinStack obj = new MinStack();
+ * obj.Push(val);
+ * obj.Pop();
+ * int param_3 = obj.Top();
+ * int param_4 = obj.GetMin();
+ */
diff --git a/LeetCodeNet/G0101_0200/S0155_min_stack/readme.md b/LeetCodeNet/G0101_0200/S0155_min_stack/readme.md
new file mode 100644
index 0000000..f3a3af2
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0155_min_stack/readme.md
@@ -0,0 +1,39 @@
+155\. Min Stack
+
+Easy
+
+Design a stack that supports push, pop, top, and retrieving the minimum element in constant time.
+
+Implement the `MinStack` class:
+
+* `MinStack()` initializes the stack object.
+* `void push(int val)` pushes the element `val` onto the stack.
+* `void pop()` removes the element on the top of the stack.
+* `int top()` gets the top element of the stack.
+* `int getMin()` retrieves the minimum element in the stack.
+
+**Example 1:**
+
+**Input**
+
+ ["MinStack","push","push","push","getMin","pop","top","getMin"]
+ [[],[-2],[0],[-3],[],[],[],[]]
+
+**Output:** [null,null,null,null,-3,null,0,-2]
+
+**Explanation:**
+
+ MinStack minStack = new MinStack();
+ minStack.push(-2);
+ minStack.push(0);
+ minStack.push(-3);
+ minStack.getMin(); // return -3
+ minStack.pop();
+ minStack.top(); // return 0
+ minStack.getMin(); // return -2
+
+**Constraints:**
+
+* -231 <= val <= 231 - 1
+* Methods `pop`, `top` and `getMin` operations will always be called on **non-empty** stacks.
+* At most 3 * 104
calls will be made to `push`, `pop`, `top`, and `getMin`.
\ No newline at end of file
diff --git a/LeetCodeNet/G0101_0200/S0160_intersection_of_two_linked_lists/Solution.cs b/LeetCodeNet/G0101_0200/S0160_intersection_of_two_linked_lists/Solution.cs
new file mode 100644
index 0000000..026b572
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0160_intersection_of_two_linked_lists/Solution.cs
@@ -0,0 +1,28 @@
+namespace LeetCodeNet.G0101_0200.S0160_intersection_of_two_linked_lists {
+
+// #Easy #Top_100_Liked_Questions #Top_Interview_Questions #Hash_Table #Two_Pointers #Linked_List
+// #Data_Structure_II_Day_11_Linked_List #Udemy_Linked_List #Big_O_Time_O(M+N)_Space_O(1)
+// #2024_01_11_Time_118_ms_(53.65%)_Space_54.6_MB_(23.25%)
+
+using LeetCodeNet.Com_github_leetcode;
+
+/**
+ * Definition for singly-linked list.
+ * public class ListNode {
+ * public int val;
+ * public ListNode next;
+ * public ListNode(int x) { val = x; }
+ * }
+ */
+public class Solution {
+ public ListNode GetIntersectionNode(ListNode headA, ListNode headB) {
+ ListNode node1 = headA;
+ ListNode node2 = headB;
+ while (node1 != node2) {
+ node1 = node1 == null ? headB : node1.next;
+ node2 = node2 == null ? headA : node2.next;
+ }
+ return node1;
+ }
+}
+}
diff --git a/LeetCodeNet/G0101_0200/S0160_intersection_of_two_linked_lists/readme.md b/LeetCodeNet/G0101_0200/S0160_intersection_of_two_linked_lists/readme.md
new file mode 100644
index 0000000..de0e1d8
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0160_intersection_of_two_linked_lists/readme.md
@@ -0,0 +1,68 @@
+160\. Intersection of Two Linked Lists
+
+Easy
+
+Given the heads of two singly linked-lists `headA` and `headB`, return _the node at which the two lists intersect_. If the two linked lists have no intersection at all, return `null`.
+
+For example, the following two linked lists begin to intersect at node `c1`:
+
+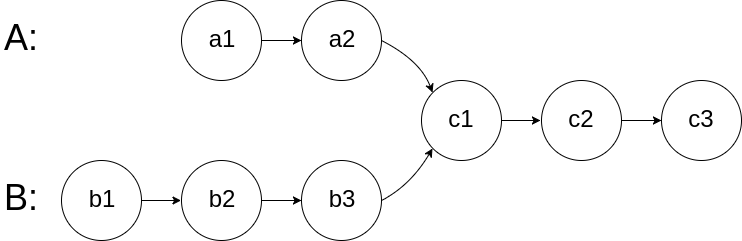
+
+The test cases are generated such that there are no cycles anywhere in the entire linked structure.
+
+**Note** that the linked lists must **retain their original structure** after the function returns.
+
+**Custom Judge:**
+
+The inputs to the **judge** are given as follows (your program is **not** given these inputs):
+
+* `intersectVal` - The value of the node where the intersection occurs. This is `0` if there is no intersected node.
+* `listA` - The first linked list.
+* `listB` - The second linked list.
+* `skipA` - The number of nodes to skip ahead in `listA` (starting from the head) to get to the intersected node.
+* `skipB` - The number of nodes to skip ahead in `listB` (starting from the head) to get to the intersected node.
+
+The judge will then create the linked structure based on these inputs and pass the two heads, `headA` and `headB` to your program. If you correctly return the intersected node, then your solution will be **accepted**.
+
+**Example 1:**
+
+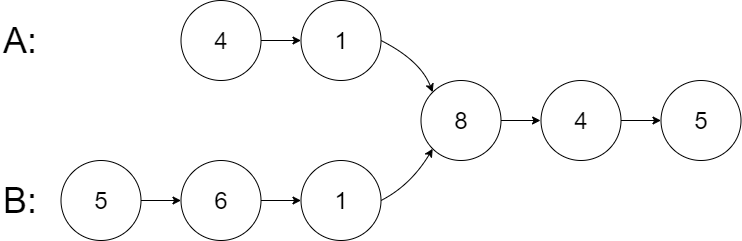
+
+**Input:** intersectVal = 8, listA = [4,1,8,4,5], listB = [5,6,1,8,4,5], skipA = 2, skipB = 3
+
+**Output:** Intersected at '8'
+
+**Explanation:** The intersected node's value is 8 (note that this must not be 0 if the two lists intersect). From the head of A, it reads as [4,1,8,4,5]. From the head of B, it reads as [5,6,1,8,4,5]. There are 2 nodes before the intersected node in A; There are 3 nodes before the intersected node in B.
+
+**Example 2:**
+
+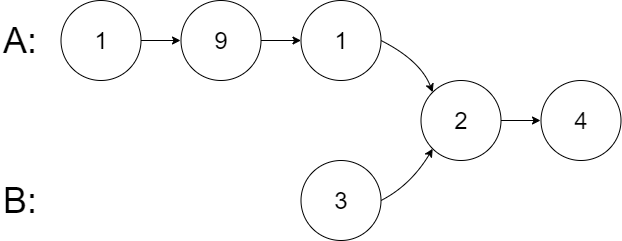
+
+**Input:** intersectVal = 2, listA = [1,9,1,2,4], listB = [3,2,4], skipA = 3, skipB = 1
+
+**Output:** Intersected at '2'
+
+**Explanation:** The intersected node's value is 2 (note that this must not be 0 if the two lists intersect). From the head of A, it reads as [1,9,1,2,4]. From the head of B, it reads as [3,2,4]. There are 3 nodes before the intersected node in A; There are 1 node before the intersected node in B.
+
+**Example 3:**
+
+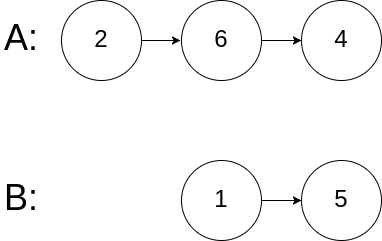
+
+**Input:** intersectVal = 0, listA = [2,6,4], listB = [1,5], skipA = 3, skipB = 2
+
+**Output:** No intersection
+
+**Explanation:** From the head of A, it reads as [2,6,4]. From the head of B, it reads as [1,5]. Since the two lists do not intersect, intersectVal must be 0, while skipA and skipB can be arbitrary values. Explanation: The two lists do not intersect, so return null.
+
+**Constraints:**
+
+* The number of nodes of `listA` is in the `m`.
+* The number of nodes of `listB` is in the `n`.
+* 0 <= m, n <= 3 * 104
+* 1 <= Node.val <= 105
+* `0 <= skipA <= m`
+* `0 <= skipB <= n`
+* `intersectVal` is `0` if `listA` and `listB` do not intersect.
+* `intersectVal == listA[skipA] == listB[skipB]` if `listA` and `listB` intersect.
+
+**Follow up:** Could you write a solution that runs in `O(n)` time and use only `O(1)` memory?
\ No newline at end of file
diff --git a/LeetCodeNet/G0101_0200/S0169_majority_element/Solution.cs b/LeetCodeNet/G0101_0200/S0169_majority_element/Solution.cs
new file mode 100644
index 0000000..c6ba7fd
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0169_majority_element/Solution.cs
@@ -0,0 +1,37 @@
+namespace LeetCodeNet.G0101_0200.S0169_majority_element {
+
+// #Easy #Top_100_Liked_Questions #Top_Interview_Questions #Array #Hash_Table #Sorting #Counting
+// #Divide_and_Conquer #Data_Structure_II_Day_1_Array #Udemy_Famous_Algorithm
+// #Big_O_Time_O(n)_Space_O(1) #2024_01_11_Time_98_ms_(66.71%)_Space_46.4_MB_(15.32%)
+
+public class Solution {
+ public int MajorityElement(int[] arr) {
+ int count = 1;
+ int majority = arr[0];
+ // For Potential Majority Element
+ for (int i = 1; i < arr.Length; i++) {
+ if (arr[i] == majority) {
+ count++;
+ } else {
+ if (count > 1) {
+ count--;
+ } else {
+ majority = arr[i];
+ }
+ }
+ }
+ // For Confirmation
+ count = 0;
+ foreach (int j in arr) {
+ if (j == majority) {
+ count++;
+ }
+ }
+ if (count >= (arr.Length / 2) + 1) {
+ return majority;
+ } else {
+ return -1;
+ }
+ }
+}
+}
diff --git a/LeetCodeNet/G0101_0200/S0169_majority_element/readme.md b/LeetCodeNet/G0101_0200/S0169_majority_element/readme.md
new file mode 100644
index 0000000..5ea5071
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0169_majority_element/readme.md
@@ -0,0 +1,27 @@
+169\. Majority Element
+
+Easy
+
+Given an array `nums` of size `n`, return _the majority element_.
+
+The majority element is the element that appears more than `⌊n / 2⌋` times. You may assume that the majority element always exists in the array.
+
+**Example 1:**
+
+**Input:** nums = [3,2,3]
+
+**Output:** 3
+
+**Example 2:**
+
+**Input:** nums = [2,2,1,1,1,2,2]
+
+**Output:** 2
+
+**Constraints:**
+
+* `n == nums.length`
+* 1 <= n <= 5 * 104
+* -231 <= nums[i] <= 231 - 1
+
+**Follow-up:** Could you solve the problem in linear time and in `O(1)` space?
\ No newline at end of file
diff --git a/LeetCodeNet/G0101_0200/S0189_rotate_array/Solution.cs b/LeetCodeNet/G0101_0200/S0189_rotate_array/Solution.cs
new file mode 100644
index 0000000..f3a814d
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0189_rotate_array/Solution.cs
@@ -0,0 +1,26 @@
+namespace LeetCodeNet.G0101_0200.S0189_rotate_array {
+
+// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Math #Two_Pointers
+// #Algorithm_I_Day_2_Two_Pointers #Udemy_Arrays #Big_O_Time_O(n)_Space_O(1)
+// #2024_01_11_Time_143_ms_(94.32%)_Space_62.9_MB_(24.95%)
+
+public class Solution {
+ private void Reverse(int[] nums, int l, int r) {
+ while (l <= r) {
+ int temp = nums[l];
+ nums[l] = nums[r];
+ nums[r] = temp;
+ l++;
+ r--;
+ }
+ }
+
+ public void Rotate(int[] nums, int k) {
+ int n = nums.Length;
+ int t = n - (k % n);
+ Reverse(nums, 0, t - 1);
+ Reverse(nums, t, n - 1);
+ Reverse(nums, 0, n - 1);
+ }
+}
+}
diff --git a/LeetCodeNet/G0101_0200/S0189_rotate_array/readme.md b/LeetCodeNet/G0101_0200/S0189_rotate_array/readme.md
new file mode 100644
index 0000000..390f269
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0189_rotate_array/readme.md
@@ -0,0 +1,39 @@
+189\. Rotate Array
+
+Medium
+
+Given an array, rotate the array to the right by `k` steps, where `k` is non-negative.
+
+**Example 1:**
+
+**Input:** nums = [1,2,3,4,5,6,7], k = 3
+
+**Output:** [5,6,7,1,2,3,4]
+
+**Explanation:**
+
+ rotate 1 steps to the right: [7,1,2,3,4,5,6]
+ rotate 2 steps to the right: [6,7,1,2,3,4,5]
+ rotate 3 steps to the right: [5,6,7,1,2,3,4]
+
+**Example 2:**
+
+**Input:** nums = [-1,-100,3,99], k = 2
+
+**Output:** [3,99,-1,-100]
+
+**Explanation:**
+
+ rotate 1 steps to the right: [99,-1,-100,3]
+ rotate 2 steps to the right: [3,99,-1,-100]
+
+**Constraints:**
+
+* 1 <= nums.length <= 105
+* -231 <= nums[i] <= 231 - 1
+* 0 <= k <= 105
+
+**Follow up:**
+
+* Try to come up with as many solutions as you can. There are at least **three** different ways to solve this problem.
+* Could you do it in-place with `O(1)` extra space?
\ No newline at end of file
diff --git a/LeetCodeNet/G0101_0200/S0198_house_robber/Solution.cs b/LeetCodeNet/G0101_0200/S0198_house_robber/Solution.cs
new file mode 100644
index 0000000..80a03e8
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0198_house_robber/Solution.cs
@@ -0,0 +1,28 @@
+namespace LeetCodeNet.G0101_0200.S0198_house_robber {
+
+// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Dynamic_Programming
+// #Algorithm_I_Day_12_Dynamic_Programming #Dynamic_Programming_I_Day_3
+// #Level_2_Day_12_Dynamic_Programming #Udemy_Dynamic_Programming #Big_O_Time_O(n)_Space_O(n)
+// #2024_01_11_Time_44_ms_(99.89%)_Space_39.8_MB_(13.60%)
+
+public class Solution {
+ public int Rob(int[] nums) {
+ if (nums.Length == 0) {
+ return 0;
+ }
+ if (nums.Length == 1) {
+ return nums[0];
+ }
+ if (nums.Length == 2) {
+ return Math.Max(nums[0], nums[1]);
+ }
+ int[] profit = new int[nums.Length];
+ profit[0] = nums[0];
+ profit[1] = Math.Max(nums[1], nums[0]);
+ for (int i = 2; i < nums.Length; i++) {
+ profit[i] = Math.Max(profit[i - 1], nums[i] + profit[i - 2]);
+ }
+ return profit[nums.Length - 1];
+ }
+}
+}
diff --git a/LeetCodeNet/G0101_0200/S0198_house_robber/readme.md b/LeetCodeNet/G0101_0200/S0198_house_robber/readme.md
new file mode 100644
index 0000000..4a93a57
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0198_house_robber/readme.md
@@ -0,0 +1,34 @@
+198\. House Robber
+
+Medium
+
+You are a professional robber planning to rob houses along a street. Each house has a certain amount of money stashed, the only constraint stopping you from robbing each of them is that adjacent houses have security systems connected and **it will automatically contact the police if two adjacent houses were broken into on the same night**.
+
+Given an integer array `nums` representing the amount of money of each house, return _the maximum amount of money you can rob tonight **without alerting the police**_.
+
+**Example 1:**
+
+**Input:** nums = [1,2,3,1]
+
+**Output:** 4
+
+**Explanation:**
+
+ Rob house 1 (money = 1) and then rob house 3 (money = 3).
+ Total amount you can rob = 1 + 3 = 4.
+
+**Example 2:**
+
+**Input:** nums = [2,7,9,3,1]
+
+**Output:** 12
+
+**Explanation:**
+
+ Rob house 1 (money = 2), rob house 3 (money = 9) and rob house 5 (money = 1).
+ Total amount you can rob = 2 + 9 + 1 = 12.
+
+**Constraints:**
+
+* `1 <= nums.length <= 100`
+* `0 <= nums[i] <= 400`
\ No newline at end of file
diff --git a/LeetCodeNet/G0101_0200/S0200_number_of_islands/Solution.cs b/LeetCodeNet/G0101_0200/S0200_number_of_islands/Solution.cs
new file mode 100644
index 0000000..28769b7
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0200_number_of_islands/Solution.cs
@@ -0,0 +1,36 @@
+namespace LeetCodeNet.G0101_0200.S0200_number_of_islands {
+
+// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Depth_First_Search
+// #Breadth_First_Search #Matrix #Union_Find
+// #Algorithm_II_Day_6_Breadth_First_Search_Depth_First_Search
+// #Graph_Theory_I_Day_1_Matrix_Related_Problems #Level_1_Day_9_Graph/BFS/DFS #Udemy_Graph
+// #Big_O_Time_O(M*N)_Space_O(M*N) #2024_01_11_Time_119_ms_(51.45%)_Space_51_MB_(45.02%)
+
+public class Solution {
+ public int NumIslands(char[][] grid) {
+ int islands = 0;
+ if (grid != null && grid.Length != 0 && grid[0].Length != 0) {
+ for (int i = 0; i < grid.Length; i++) {
+ for (int j = 0; j < grid[0].Length; j++) {
+ if (grid[i][j] == '1') {
+ Dfs(grid, i, j);
+ islands++;
+ }
+ }
+ }
+ }
+ return islands;
+ }
+
+ private void Dfs(char[][] grid, int x, int y) {
+ if (x < 0 || grid.Length <= x || y < 0 || grid[0].Length <= y || grid[x][y] != '1') {
+ return;
+ }
+ grid[x][y] = 'x';
+ Dfs(grid, x + 1, y);
+ Dfs(grid, x - 1, y);
+ Dfs(grid, x, y + 1);
+ Dfs(grid, x, y - 1);
+ }
+}
+}
diff --git a/LeetCodeNet/G0101_0200/S0200_number_of_islands/readme.md b/LeetCodeNet/G0101_0200/S0200_number_of_islands/readme.md
new file mode 100644
index 0000000..0c4292d
--- /dev/null
+++ b/LeetCodeNet/G0101_0200/S0200_number_of_islands/readme.md
@@ -0,0 +1,40 @@
+200\. Number of Islands
+
+Medium
+
+Given an `m x n` 2D binary grid `grid` which represents a map of `'1'`s (land) and `'0'`s (water), return _the number of islands_.
+
+An **island** is surrounded by water and is formed by connecting adjacent lands horizontally or vertically. You may assume all four edges of the grid are all surrounded by water.
+
+**Example 1:**
+
+**Input:**
+
+ grid = [
+ ["1","1","1","1","0"],
+ ["1","1","0","1","0"],
+ ["1","1","0","0","0"],
+ ["0","0","0","0","0"]
+ ]
+
+**Output:** 1
+
+**Example 2:**
+
+**Input:**
+
+ grid = [
+ ["1","1","0","0","0"],
+ ["1","1","0","0","0"],
+ ["0","0","1","0","0"],
+ ["0","0","0","1","1"]
+ ]
+
+**Output:** 3
+
+**Constraints:**
+
+* `m == grid.length`
+* `n == grid[i].length`
+* `1 <= m, n <= 300`
+* `grid[i][j]` is `'0'` or `'1'`.
\ No newline at end of file
diff --git a/README.md b/README.md
index 32ddde4..a534a00 100644
--- a/README.md
+++ b/README.md
@@ -148,6 +148,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0198 |[House Robber](LeetCodeNet/G0101_0200/S0198_house_robber/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Big_O_Time_O(n)_Space_O(n) | 44 | 99.89
#### Day 4
@@ -166,6 +167,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0152 |[Maximum Product Subarray](LeetCodeNet/G0101_0200/S0152_maximum_product_subarray/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Big_O_Time_O(N)_Space_O(1) | 71 | 90.35
#### Day 7
@@ -415,6 +417,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0155 |[Min Stack](LeetCodeNet/G0101_0200/S0155_min_stack/MinStack.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Stack, Design, Big_O_Time_O(1)_Space_O(N) | 105 | 95.77
#### Day 19
@@ -432,6 +435,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0200 |[Number of Islands](LeetCodeNet/G0101_0200/S0200_number_of_islands/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Depth_First_Search, Breadth_First_Search, Matrix, Union_Find, Big_O_Time_O(M\*N)_Space_O(M\*N) | 119 | 51.45
#### Day 2 Matrix Related Problems
@@ -602,6 +606,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0200 |[Number of Islands](LeetCodeNet/G0101_0200/S0200_number_of_islands/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Depth_First_Search, Breadth_First_Search, Matrix, Union_Find, Big_O_Time_O(M\*N)_Space_O(M\*N) | 119 | 51.45
#### Day 10 Dynamic Programming
@@ -708,6 +713,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0198 |[House Robber](LeetCodeNet/G0101_0200/S0198_house_robber/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Big_O_Time_O(n)_Space_O(n) | 44 | 99.89
| 0322 |[Coin Change](LeetCodeNet/G0301_0400/S0322_coin_change/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Breadth_First_Search, Big_O_Time_O(m\*n)_Space_O(amount) | 78 | 90.63
#### Day 13 Dynamic Programming
@@ -715,6 +721,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
| 0416 |[Partition Equal Subset Sum](LeetCodeNet/G0401_0500/S0416_partition_equal_subset_sum/Solution.cs)| Medium | Top_100_Liked_Questions, Array, Dynamic_Programming, Big_O_Time_O(n\*sums)_Space_O(n\*sums) | 95 | 97.38
+| 0152 |[Maximum Product Subarray](LeetCodeNet/G0101_0200/S0152_maximum_product_subarray/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Big_O_Time_O(N)_Space_O(1) | 71 | 90.35
#### Day 14 Sliding Window/Two Pointer
@@ -733,6 +740,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0155 |[Min Stack](LeetCodeNet/G0101_0200/S0155_min_stack/MinStack.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Stack, Design, Big_O_Time_O(1)_Space_O(N) | 105 | 95.77
| 0208 |[Implement Trie (Prefix Tree)](LeetCodeNet/G0201_0300/S0208_implement_trie_prefix_tree/Trie.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, String, Hash_Table, Design, Trie, Big_O_Time_O(word.length())_or_O(prefix.length())_Space_O(N) | 178 | 88.12
#### Day 17 Interval
@@ -783,6 +791,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
| 0033 |[Search in Rotated Sorted Array](LeetCodeNet/G0001_0100/S0033_search_in_rotated_sorted_array/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Binary_Search, Big_O_Time_O(log_n)_Space_O(1) | 63 | 96.89
+| 0153 |[Find Minimum in Rotated Sorted Array](LeetCodeNet/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/Solution.cs)| Medium | Top_100_Liked_Questions, Array, Binary_Search, Big_O_Time_O(log_N)_Space_O(log_N) | 64 | 88.59
#### Udemy Arrays
@@ -791,6 +800,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| 0121 |[Best Time to Buy and Sell Stock](LeetCodeNet/G0101_0200/S0121_best_time_to_buy_and_sell_stock/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Big_O_Time_O(N)_Space_O(1) | 328 | 35.43
| 0283 |[Move Zeroes](LeetCodeNet/G0201_0300/S0283_move_zeroes/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Array, Two_Pointers, Big_O_Time_O(n)_Space_O(1) | 133 | 96.30
| 0001 |[Two Sum](LeetCodeNet/G0001_0100/S0001_two_sum/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Array, Hash_Table, Big_O_Time_O(n)_Space_O(n) | 134 | 81.26
+| 0189 |[Rotate Array](LeetCodeNet/G0101_0200/S0189_rotate_array/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Math, Two_Pointers, Big_O_Time_O(n)_Space_O(1) | 143 | 94.32
| 0055 |[Jump Game](LeetCodeNet/G0001_0100/S0055_jump_game/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Greedy, Big_O_Time_O(n)_Space_O(1) | 189 | 38.02
| 0075 |[Sort Colors](LeetCodeNet/G0001_0100/S0075_sort_colors/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Sorting, Two_Pointers, Big_O_Time_O(n)_Space_O(1) | 98 | 93.59
| 0238 |[Product of Array Except Self](LeetCodeNet/G0201_0300/S0238_product_of_array_except_self/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Prefix_Sum, Big_O_Time_O(n^2)_Space_O(n) | 141 | 94.24
@@ -809,6 +819,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
| 0053 |[Maximum Subarray](LeetCodeNet/G0001_0100/S0053_maximum_subarray/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Divide_and_Conquer, Big_O_Time_O(n)_Space_O(1) | 270 | 38.35
+| 0169 |[Majority Element](LeetCodeNet/G0101_0200/S0169_majority_element/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Array, Hash_Table, Sorting, Counting, Divide_and_Conquer, Big_O_Time_O(n)_Space_O(1) | 98 | 66.71
#### Udemy Sorting Algorithms
@@ -834,6 +845,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| 0141 |[Linked List Cycle](LeetCodeNet/G0101_0200/S0141_linked_list_cycle/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Hash_Table, Two_Pointers, Linked_List, Big_O_Time_O(N)_Space_O(1) | 76 | 99.02
| 0206 |[Reverse Linked List](LeetCodeNet/G0201_0300/S0206_reverse_linked_list/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Linked_List, Recursion, Big_O_Time_O(N)_Space_O(1) | 57 | 95.02
| 0021 |[Merge Two Sorted Lists](LeetCodeNet/G0001_0100/S0021_merge_two_sorted_lists/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Linked_List, Recursion, Big_O_Time_O(m+n)_Space_O(m+n) | 69 | 92.74
+| 0160 |[Intersection of Two Linked Lists](LeetCodeNet/G0101_0200/S0160_intersection_of_two_linked_lists/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Hash_Table, Two_Pointers, Linked_List, Big_O_Time_O(M+N)_Space_O(1) | 118 | 53.65
| 0234 |[Palindrome Linked List](LeetCodeNet/G0201_0300/S0234_palindrome_linked_list/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Two_Pointers, Stack, Linked_List, Recursion, Big_O_Time_O(n)_Space_O(1) | 306 | 31.81
| 0138 |[Copy List with Random Pointer](LeetCodeNet/G0101_0200/S0138_copy_list_with_random_pointer/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Hash_Table, Linked_List, Big_O_Time_O(N)_Space_O(N) | 59 | 96.51
| 0025 |[Reverse Nodes in k-Group](LeetCodeNet/G0001_0100/S0025_reverse_nodes_in_k_group/Solution.cs)| Hard | Top_100_Liked_Questions, Linked_List, Recursion, Big_O_Time_O(n)_Space_O(k) | 75 | 86.97
@@ -862,12 +874,15 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0200 |[Number of Islands](LeetCodeNet/G0101_0200/S0200_number_of_islands/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Depth_First_Search, Breadth_First_Search, Matrix, Union_Find, Big_O_Time_O(M\*N)_Space_O(M\*N) | 119 | 51.45
#### Udemy Dynamic Programming
| | | | | |
|-|-|-|-|-|-
| 0139 |[Word Break](LeetCodeNet/G0101_0200/S0139_word_break/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, String, Hash_Table, Dynamic_Programming, Trie, Memoization, Big_O_Time_O(M+max\*N)_Space_O(M+N+max) | 64 | 98.44
+| 0152 |[Maximum Product Subarray](LeetCodeNet/G0101_0200/S0152_maximum_product_subarray/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Big_O_Time_O(N)_Space_O(1) | 71 | 90.35
+| 0198 |[House Robber](LeetCodeNet/G0101_0200/S0198_house_robber/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Big_O_Time_O(n)_Space_O(n) | 44 | 99.89
| 0070 |[Climbing Stairs](LeetCodeNet/G0001_0100/S0070_climbing_stairs/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Dynamic_Programming, Math, Memoization, Big_O_Time_O(n)_Space_O(n) | 15 | 94.90
| 0064 |[Minimum Path Sum](LeetCodeNet/G0001_0100/S0064_minimum_path_sum/Solution.cs)| Medium | Top_100_Liked_Questions, Array, Dynamic_Programming, Matrix, Big_O_Time_O(m\*n)_Space_O(m\*n) | 74 | 94.37
| 0300 |[Longest Increasing Subsequence](LeetCodeNet/G0201_0300/S0300_longest_increasing_subsequence/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Binary_Search, Big_O_Time_O(n\*log_n)_Space_O(n) | 80 | 89.11
@@ -895,6 +910,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0155 |[Min Stack](LeetCodeNet/G0101_0200/S0155_min_stack/MinStack.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Stack, Design, Big_O_Time_O(1)_Space_O(N) | 105 | 95.77
### Data Structure I
@@ -989,6 +1005,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
| 0136 |[Single Number](LeetCodeNet/G0101_0200/S0136_single_number/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Array, Bit_Manipulation, Big_O_Time_O(N)_Space_O(1) | 87 | 93.37
+| 0169 |[Majority Element](LeetCodeNet/G0101_0200/S0169_majority_element/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Array, Hash_Table, Sorting, Counting, Divide_and_Conquer, Big_O_Time_O(n)_Space_O(1) | 98 | 66.71
| 0015 |[3Sum](LeetCodeNet/G0001_0100/S0015_3sum/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Sorting, Two_Pointers, Big_O_Time_O(n\*log(n))_Space_O(n^2) | 173 | 75.85
#### Day 2 Array
@@ -1051,6 +1068,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0160 |[Intersection of Two Linked Lists](LeetCodeNet/G0101_0200/S0160_intersection_of_two_linked_lists/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Hash_Table, Two_Pointers, Linked_List, Big_O_Time_O(M+N)_Space_O(1) | 118 | 53.65
#### Day 12 Linked List
@@ -1068,6 +1086,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0155 |[Min Stack](LeetCodeNet/G0101_0200/S0155_min_stack/MinStack.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Stack, Design, Big_O_Time_O(1)_Space_O(N) | 105 | 95.77
#### Day 15 Tree
@@ -1121,6 +1140,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0189 |[Rotate Array](LeetCodeNet/G0101_0200/S0189_rotate_array/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Math, Two_Pointers, Big_O_Time_O(n)_Space_O(1) | 143 | 94.32
#### Day 3 Two Pointers
@@ -1178,6 +1198,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
| 0070 |[Climbing Stairs](LeetCodeNet/G0001_0100/S0070_climbing_stairs/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Dynamic_Programming, Math, Memoization, Big_O_Time_O(n)_Space_O(n) | 15 | 94.90
+| 0198 |[House Robber](LeetCodeNet/G0101_0200/S0198_house_robber/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Big_O_Time_O(n)_Space_O(n) | 44 | 99.89
#### Day 13 Bit Manipulation
@@ -1204,6 +1225,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0153 |[Find Minimum in Rotated Sorted Array](LeetCodeNet/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/Solution.cs)| Medium | Top_100_Liked_Questions, Array, Binary_Search, Big_O_Time_O(log_N)_Space_O(log_N) | 64 | 88.59
#### Day 3 Two Pointers
@@ -1227,6 +1249,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0200 |[Number of Islands](LeetCodeNet/G0101_0200/S0200_number_of_islands/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Depth_First_Search, Breadth_First_Search, Matrix, Union_Find, Big_O_Time_O(M\*N)_Space_O(M\*N) | 119 | 51.45
#### Day 7 Breadth First Search Depth First Search
@@ -1382,6 +1405,7 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| | | | | |
|-|-|-|-|-|-
+| 0153 |[Find Minimum in Rotated Sorted Array](LeetCodeNet/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/Solution.cs)| Medium | Top_100_Liked_Questions, Array, Binary_Search, Big_O_Time_O(log_N)_Space_O(log_N) | 64 | 88.59
## Algorithms
@@ -1417,6 +1441,14 @@ C#-based LeetCode algorithm problem solutions, regularly updated.
| 0208 |[Implement Trie (Prefix Tree)](LeetCodeNet/G0201_0300/S0208_implement_trie_prefix_tree/Trie.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, String, Hash_Table, Design, Trie, Level_2_Day_16_Design, Udemy_Trie_and_Heap, Big_O_Time_O(word.length())_or_O(prefix.length())_Space_O(N) | 178 | 88.12
| 0207 |[Course Schedule](LeetCodeNet/G0201_0300/S0207_course_schedule/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Depth_First_Search, Breadth_First_Search, Graph, Topological_Sort, Big_O_Time_O(N)_Space_O(N) | 95 | 91.94
| 0206 |[Reverse Linked List](LeetCodeNet/G0201_0300/S0206_reverse_linked_list/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Linked_List, Recursion, Data_Structure_I_Day_8_Linked_List, Algorithm_I_Day_10_Recursion_Backtracking, Level_1_Day_3_Linked_List, Udemy_Linked_List, Big_O_Time_O(N)_Space_O(1) | 57 | 95.02
+| 0200 |[Number of Islands](LeetCodeNet/G0101_0200/S0200_number_of_islands/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Depth_First_Search, Breadth_First_Search, Matrix, Union_Find, Algorithm_II_Day_6_Breadth_First_Search_Depth_First_Search, Graph_Theory_I_Day_1_Matrix_Related_Problems, Level_1_Day_9_Graph/BFS/DFS, Udemy_Graph, Big_O_Time_O(M\*N)_Space_O(M\*N) | 119 | 51.45
+| 0198 |[House Robber](LeetCodeNet/G0101_0200/S0198_house_robber/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Algorithm_I_Day_12_Dynamic_Programming, Dynamic_Programming_I_Day_3, Level_2_Day_12_Dynamic_Programming, Udemy_Dynamic_Programming, Big_O_Time_O(n)_Space_O(n) | 44 | 99.89
+| 0189 |[Rotate Array](LeetCodeNet/G0101_0200/S0189_rotate_array/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Math, Two_Pointers, Algorithm_I_Day_2_Two_Pointers, Udemy_Arrays, Big_O_Time_O(n)_Space_O(1) | 143 | 94.32
+| 0169 |[Majority Element](LeetCodeNet/G0101_0200/S0169_majority_element/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Array, Hash_Table, Sorting, Counting, Divide_and_Conquer, Data_Structure_II_Day_1_Array, Udemy_Famous_Algorithm, Big_O_Time_O(n)_Space_O(1) | 98 | 66.71
+| 0160 |[Intersection of Two Linked Lists](LeetCodeNet/G0101_0200/S0160_intersection_of_two_linked_lists/Solution.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Hash_Table, Two_Pointers, Linked_List, Data_Structure_II_Day_11_Linked_List, Udemy_Linked_List, Big_O_Time_O(M+N)_Space_O(1) | 118 | 53.65
+| 0155 |[Min Stack](LeetCodeNet/G0101_0200/S0155_min_stack/MinStack.cs)| Easy | Top_100_Liked_Questions, Top_Interview_Questions, Stack, Design, Data_Structure_II_Day_14_Stack_Queue, Programming_Skills_II_Day_18, Level_2_Day_16_Design, Udemy_Design, Big_O_Time_O(1)_Space_O(N) | 105 | 95.77
+| 0153 |[Find Minimum in Rotated Sorted Array](LeetCodeNet/G0101_0200/S0153_find_minimum_in_rotated_sorted_array/Solution.cs)| Medium | Top_100_Liked_Questions, Array, Binary_Search, Algorithm_II_Day_2_Binary_Search, Binary_Search_I_Day_12, Udemy_Binary_Search, Big_O_Time_O(log_N)_Space_O(log_N) | 64 | 88.59
+| 0152 |[Maximum Product Subarray](LeetCodeNet/G0101_0200/S0152_maximum_product_subarray/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Array, Dynamic_Programming, Dynamic_Programming_I_Day_6, Level_2_Day_13_Dynamic_Programming, Udemy_Dynamic_Programming, Big_O_Time_O(N)_Space_O(1) | 71 | 90.35
| 0148 |[Sort List](LeetCodeNet/G0101_0200/S0148_sort_list/Solution.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Sorting, Two_Pointers, Linked_List, Divide_and_Conquer, Merge_Sort, Level_2_Day_4_Linked_List, Big_O_Time_O(log(N))_Space_O(log(N)) | 141 | 45.08
| 0146 |[LRU Cache](LeetCodeNet/G0101_0200/S0146_lru_cache/LRUCache.cs)| Medium | Top_100_Liked_Questions, Top_Interview_Questions, Hash_Table, Design, Linked_List, Doubly_Linked_List, Udemy_Linked_List, Big_O_Time_O(1)_Space_O(capacity) | 780 | 34.57
| 0142 |[Linked List Cycle II](LeetCodeNet/G0101_0200/S0142_linked_list_cycle_ii/Solution.cs)| Medium | Top_100_Liked_Questions, Hash_Table, Two_Pointers, Linked_List, Data_Structure_II_Day_10_Linked_List, Level_1_Day_4_Linked_List, Udemy_Linked_List, Big_O_Time_O(N)_Space_O(1) | 72 | 94.58