-
-
Notifications
You must be signed in to change notification settings - Fork 90
Usage
Souvik edited this page Jan 29, 2022
·
6 revisions
This is how the notion page looks for this example:
const { Client } = require("@notionhq/client");
const { NotionToMarkdown } = require("notion-to-md");
// or
// import {NotionToMarkdown} from "notion-to-md";
const notion = new Client({
auth: "your integration token",
});
// passing notion client as
const n2m = new NotionToMarkdown({ notionClient: notion });
(async () => {
const mdblocks = await n2m.pageToMarkdown("target_page_id");
const mdString = n2m.toMarkdownString(mdblocks);
//writing to file
fs.writeFile("test.md", mdString, (err) => {
console.log(err);
});
})();
Output:
Example notion page:
const { Client } = require("@notionhq/client");
const { NotionToMarkdown } = require("notion-to-md");
const notion = new Client({
auth: "your integration token",
});
// passing notion client to the option
const n2m = new NotionToMarkdown({ notionClient: notion });
(async () => {
// notice second argument, totalPage.
// checkout API section to know more about totalPage
const x = await n2m.pageToMarkdown("target_page_id", 2);
console.log(x);
})();
Output:
[
{
"parent": "# heading 1",
"children": []
},
{
"parent": "- bullet 1",
"children": [
{
"parent": "- bullet 1.1",
"children": []
},
{
"parent": "- bullet 1.2",
"children": []
}
]
},
{
"parent": "- bullet 2",
"children": []
},
{
"parent": "- [ ] check box 1",
"children": [
{
"parent": "- [x] check box 1.2",
"children": []
},
{
"parent": "- [ ] check box 1.3",
"children": []
}
]
},
{
"parent": "- [ ] checkbox 2",
"children": []
}
]
same notion page as before
const { Client } = require("@notionhq/client");
const { NotionToMarkdown } = require("notion-to-md");
const notion = new Client({
auth: "your integration token",
});
// passing notion client to the option
const n2m = new NotionToMarkdown({ notionClient: notion });
(async () => {
// get all blocks in the page
const { results } = await notion.blocks.children.list({
block_id,
});
//convert to markdown
const x = await n2m.blocksToMarkdown(results);
console.log(x);
})();
Output: same as the previous one
- only takes a single notion block and returns the corresponding markdown string
const { NotionToMarkdown } = require("notion-to-md");
// passing notion client to the option
const n2m = new NotionToMarkdown({ notionClient: notion });
const result = n2m.blockToMarkdown(block);
console.log(result);
result:
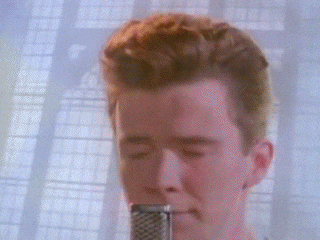