diff --git a/README.md b/README.md
index 7496f6a..3d79530 100644
--- a/README.md
+++ b/README.md
@@ -1,18 +1,19 @@
-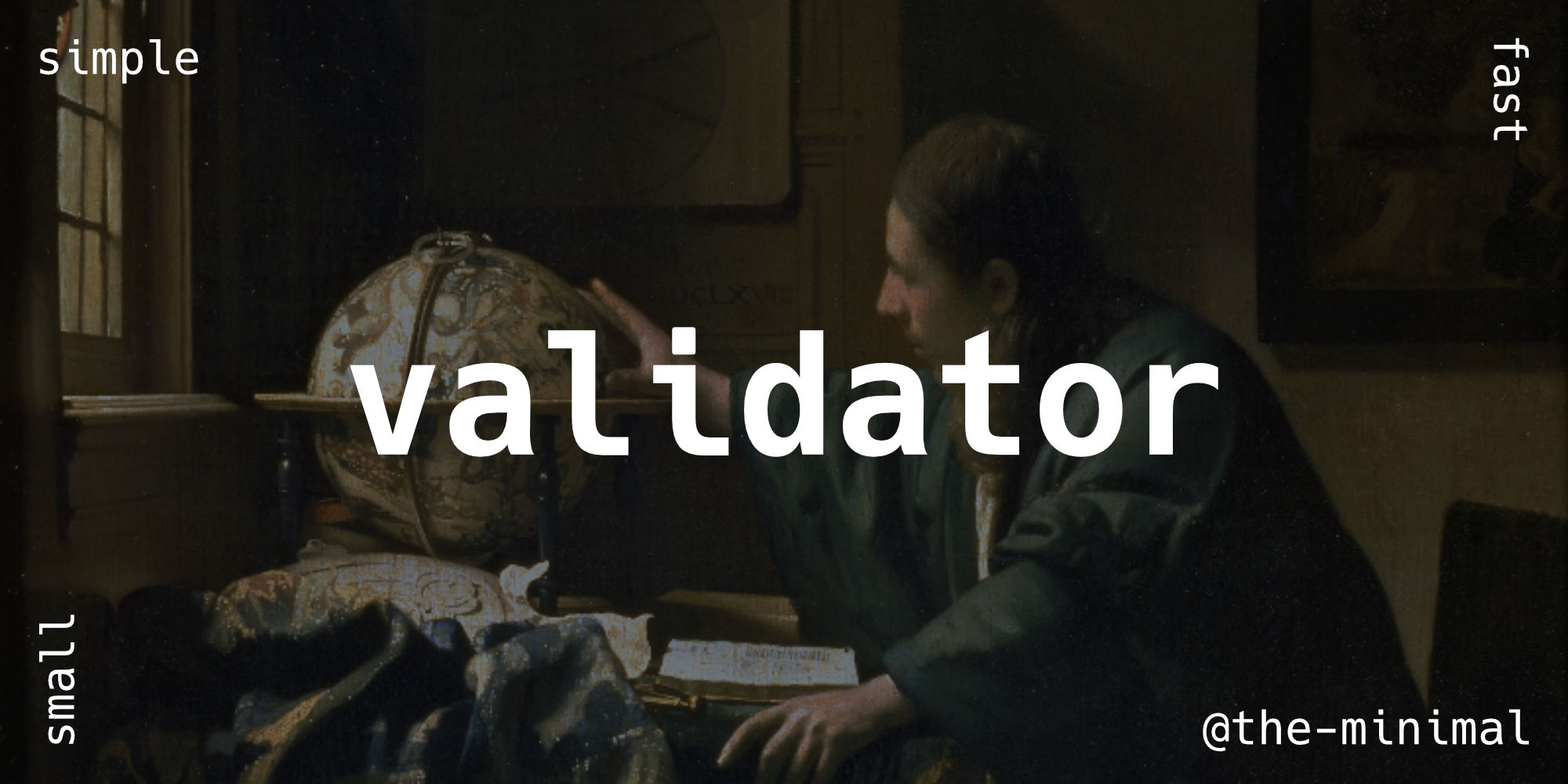
+# Validator: A Minimal Data Validation Library
-Joe was in search of a simple data validation library that he could learn in 15 minutes.
+Validator is a highly optimized data validation library designed for simplicity, performance, and ease of use. It aims to meet the needs of developers who require minimal blocking time and low CPU/memory overhead.
-He envisioned a tool with minimal blocking time and low CPU/memory overhead.
+## Features
-He hoped for something he could easily extend to meet his specific requirements.
+- Data validation only
+- Small learning curve
+- Minimal runtime overhead
+- Tiny bundle size
+- Tree-shakeable
+- Static type inference
+- Composable and modular
+- 100% Test Coverage
-> Are you like Joe? If so, then `Validator` might just be what you're looking for!
-
-## Stubborn opinions
-
-`Validator` is pretty stubborn and doesn't want to be a Jack of all trades.
-
-As a result it has many opinions that might not sit well with some folks.
+## Opinionated
No data transformation
@@ -129,111 +130,70 @@ As a result it has many opinions that might not sit well with some folks.
-## Focused features
-
-`Validator` is a watchful eye that plagues your editor with errors if you feed it data you haven't agreed upon, but otherwise, it stays quiet as a mouse.
-
-- Everything is an `Assertion`
-- `Assertion`s are simple functions
-- `Assertion`s are composed together
-- `Assertion`s are type-safe
-- `Assertion`s are tree-shakeable
-
-## Incredible numbers
-
-`Validator` is an obsessed overachiever who wants to be the smallest and fastest one on the track.
-
-- 40 `Assertion`s
-- 835 bytes bundle
-- ~ 5x faster data validation than `Zod`
-- ~ 200x less memory consumption than `Zod`
-- ~ 50x faster type-checking than `Zod`
-- 1 runtime dependency
- - [@the-minimal/error](https://github.com/the-minimal/error) (135 bytes)
-- 100% test coverage
-
-## Simple examples
-
-
- How do I validate types?
-
- ```ts
- assert(string, "Hello, World!");
- assert(number, 420);
- assert(boolean, true);
- ```
-
-
-
-
- How do I validate values?
-
- ```ts
- value(26);
- notValue(0);
- minValue(18);
- maxValue(100);
- rangeValue(18, 100);
- ```
+## Performance Metrics
-
+Validator is engineered to deliver exceptional performance:
-
- How do I validate lenghts?
+- **Bundle Size**: 835 bytes (minified and gzipped)
+- **Speed**: Approximately 5x faster than Zod for data validation
+- **Memory Usage**: Approximately 200x less memory consumption than Zod
+- **Type-checking**: Approximately 50x faster than Zod
+- **Dependencies**: Only one in-house dependency
+- **Test Coverage**: 100%
- ```ts
- length(5);
- notLength(0);
- minLength(8);
- maxLength(16);
- rangeLength(8, 16);
- ```
+## Usage Examples
-
+### Validating Types
-
- How do I combine validations?
+```ts
+assert(string, "Hello, World!");
+assert(number, 420);
+assert(boolean, true);
+```
- ```ts
- const register = object({
- email: and([string, rangeLength(5, 35), email]),
- password: and([string, rangeLength(8, 16)]),
- role: union(["ADMIN", "USER"]),
- friends: array(string)
- });
-
- assert(
- register,
- "Oh no this is gonna throw"
- );
-
- assert(
- register
- {
- email: "yamiteru@icloud.com",
- password: "Test123456",
- role: "ADMIN",
- friends: ["Joe"]
- }
- );
- ```
+### Validating Values
-
+```ts
+value(26);
+notValue(0);
+minValue(18);
+maxValue(100);
+rangeValue(18, 100);
+```
-## Great journey
+### Validating Lengths
-Are you really Joe?
+```ts
+length(5);
+notLength(0);
+minLength(8);
+maxLength(16);
+rangeLength(8, 16);
+```
-Do you dare to let this demon command you?
+### Combining Validations
+
+```ts
+const register = object({
+ email: and([string, rangeLength(5, 35), email]),
+ password: and([string, rangeLength(8, 16)]),
+ role: union(["ADMIN", "USER"]),
+ friends: array(string)
+});
+
+assert(
+ register,
+ {
+ email: "yamiteru@icloud.com",
+ password: "Test123456",
+ role: "ADMIN",
+ friends: ["Joe"]
+ }
+);
+```
-If so, repeat the spell below and good luck on your journey!
+## Installation
```bash
yarn add @the-minimal/validator
```
-
-## Notes
-
-- All reported sizes are for minified and gzipped code
-- Reproducible and highly detailed benchmarks are on the way
-- `Validation` and `Assertion` are the same things
diff --git a/docs/the-minimal-validator.jpg b/docs/the-minimal-validator.jpg
deleted file mode 100644
index 9f431b1..0000000
Binary files a/docs/the-minimal-validator.jpg and /dev/null differ