-
Notifications
You must be signed in to change notification settings - Fork 1.1k
CTkSegmentedButton
Tom Schimansky edited this page Dec 7, 2022
·
10 revisions
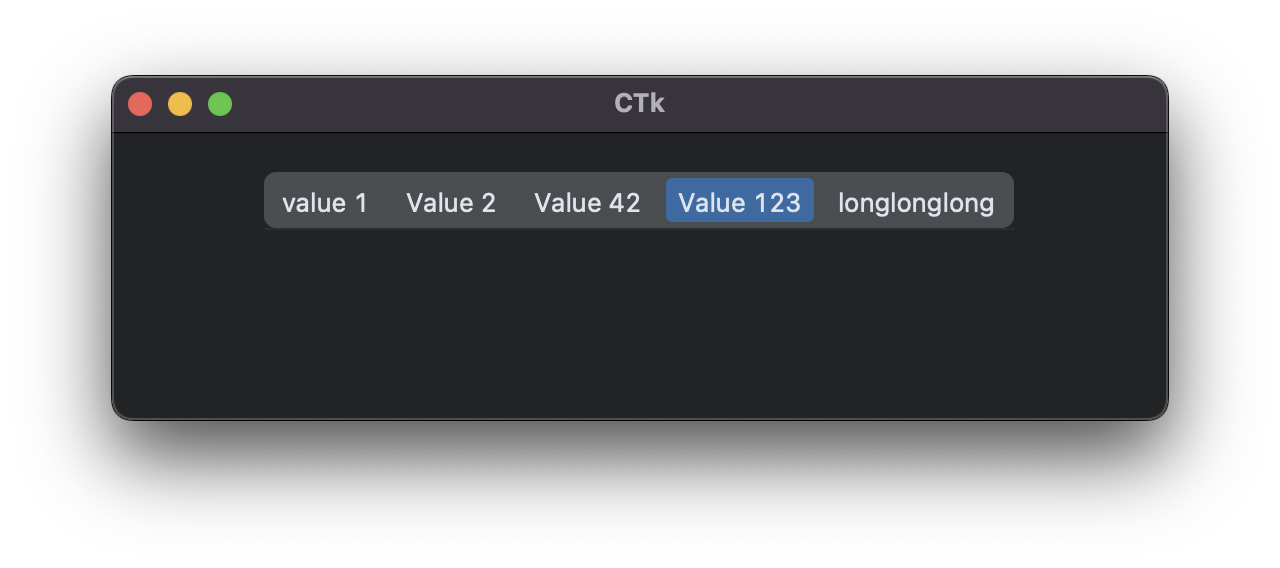
Without variable:
def segmented_button_callback(value):
print("segmented button clicked:", value)
segemented_button = customtkinter.CTkSegmentedButton(master=app,
values=["Value 1", "Value 2", "Value 3"],
command=segmented_button_callback)
segemented_button.pack(padx=20, pady=10)
segemented_button.set("Value 1") # set initial value
With variable:
segemented_button_var = customtkinter.StringVar(value="Value 1") # set initial value
segemented_button = customtkinter.CTkSegmentedButton(master=app,
values=["Value 1", "Value 2", "Value 3"],
variable=segemented_button_var)
segemented_button.pack(padx=20, pady=10)
argument | value |
---|---|
master | root, frame, top-level |
width | box width in px |
height | box height in px |
corner_radius | corner radius in px |
border_width | space in px between buttons and the edges of the widget |
fg_color | color around the buttons, tuple: (light_color, dark_color) or single color |
selected_color | color of the selected button, tuple: (light_color, dark_color) or single color |
selected_hover_color | hover color of selected button, tuple: (light_color, dark_color) or single color |
unselected_color | color of the unselected buttons, tuple: (light_color, dark_color) or single color or "transparent" |
unselected_hover_color | hover color of unselected buttons, tuple: (light_color, dark_color) or single color |
text_color | text color, tuple: (light_color, dark_color) or single color |
text_color_disabled | text color when disabled, tuple: (light_color, dark_color) or single color |
font | button text font, tuple: (font_name, size) |
values | list of string values for the buttons, can't be empty |
variable | StringVar to control the current selected value |
state | "normal" (standard) or "disabled" (not clickable, darker color) |
command | function will be called when the dropdown is clicked manually |
dynamic_resizing | enable/disable automatic resizing when text is too big to fit: True (standard), False |
-
All attributes can be configured and updated.
segemented_button.configure(state=..., command=..., values=[...], variable=..., ...)
-
Get values of all attributes specified as string.
-
Set to specific value. If value is not in values list, no button will be selected.
-
Get current string value.
-
Insert new value at given index into segmented button. Value will be also inserted into the values list.
-
Move existing value to new index position.
-
Remove value from segmented button and values list. If value is currently selected, no button will be selected afterwards.
CustomTkinter by Tom Schimansky 2022
The Github Wiki is outdated, the new documentation can be found at: