-
Notifications
You must be signed in to change notification settings - Fork 8
Schema
James edited this page Jun 25, 2017
·
2 revisions
runtime validation, easy fluent api creation with types
interface ValidationFunction {
(arg: any): any
}
// all `is` validators, or any custom added ones
type Type =
| ValidationFunction
| '?'
| '|'
| '[]'
| 'string'
| 'number'
| 'date'
| 'boolean'
| 'function'
| 'error'
| 'map'
| 'set'
| 'object'
| 'regexp'
| 'array'
| 'symbol'
| 'real'
| 'iterator'
| 'objWithKeys'
| 'null'
| 'undefined'
interface Schemable {
(key: string | any): Type | Schemable
}
type Schema = Schemable | Type
const typed = new Chain()
// can be used shorthand
.method('short')
// .onValid((val, c) => c.set('eh', val))
.onInvalid((error, arg, instance) => log.data(error).echo(false))
.type(x => typeof x === 'string')
.build()
typed.short('string')
typed.short(!'boolean')
const Chain = require('chain-able')
// to use for debugging or instance checks
class CommentChain extends Chain {}
const chain = new CommentChain()
chain
.methods()
.onInvalid((error, arg, instance) => console.error(error))
.schema({
enabled: 'boolean',
data: '!string',
name: '?string',
location: 'number|number[]',
// nested
dates: {
created: {
at: 'date',
},
updated: {
at: 'date',
pretty: 'string',
},
},
})
chain
.dates({created: {at: new Date()}})
.location(1)
.enabled(true)
.name('string')
.name(['strings!'])
.name(['? is optional :-)'])
// validates with .merge or .set as well
chain.merge({data: {notString: true}})
// invalid
chain.enabled('not boolean')
chain.data('not valid')
result:
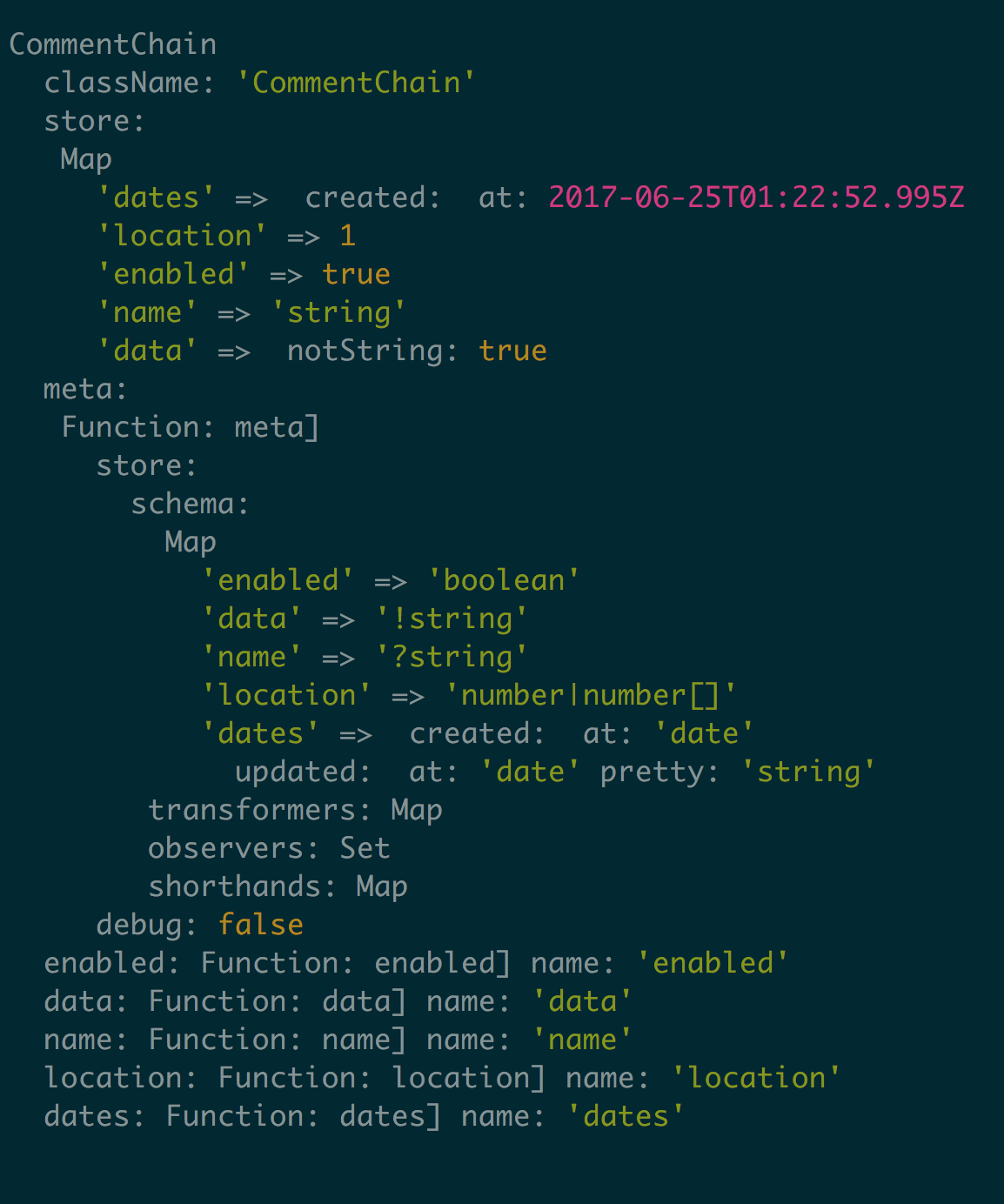
export const is = {
isArray,
isString,
isNumber,
isFunction,
isObj,
isObjWithKeys,
isEnumerable,
isError,
isMap,
isSet,
isIterator,
isDate,
isRegExp,
isPureObj,
isSymbol,
isReal,
isBoolean,
isNull,
isUndefined,
isTrue,
toS,
// only available as `chain-able/deps/is/*` without the `is`
// @example `chain-able/deps/is/stringOrNumber`, `chain-able/deps/is/dot`
isNotEmptyArray,
isStringOrNumber,
isNullOrUndef,
isFalse,
isDot,
isMapish,
}
- is available on MethodChain
- uses the traverser
- is source
- is tests
- schema source
- schema tests
- TypeDefs