-
Notifications
You must be signed in to change notification settings - Fork 1.1k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
2 changed files
with
152 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,85 @@ | ||
# [1110. 删点成林](https://leetcode.cn/problems/delete-nodes-and-return-forest/) | ||
|
||
- 标签:树、深度优先搜索、数组、哈希表、二叉树 | ||
- 难度:中等 | ||
|
||
## 题目链接 | ||
|
||
- [1110. 删点成林 - 力扣](https://leetcode.cn/problems/delete-nodes-and-return-forest/) | ||
|
||
## 题目大意 | ||
|
||
**描述**:给定二叉树的根节点 $root$,树上每个节点都有一个不同的值。 | ||
|
||
如果节点值在 $to\underline{}delete$ 中出现,我们就把该节点从树上删去,最后得到一个森林(一些不相交的树构成的集合)。 | ||
|
||
**要求**:返回森林中的每棵树。你可以按任意顺序组织答案。 | ||
|
||
**说明**: | ||
|
||
- 树中的节点数最大为 $1000$。 | ||
- 每个节点都有一个介于 $1$ 到 $1000$ 之间的值,且各不相同。 | ||
- $to\underline{}delete.length \le 1000$。 | ||
- $to\underline{}delete$ 包含一些从 $1$ 到 $1000$、各不相同的值。 | ||
|
||
**示例**: | ||
|
||
- 示例 1: | ||
|
||
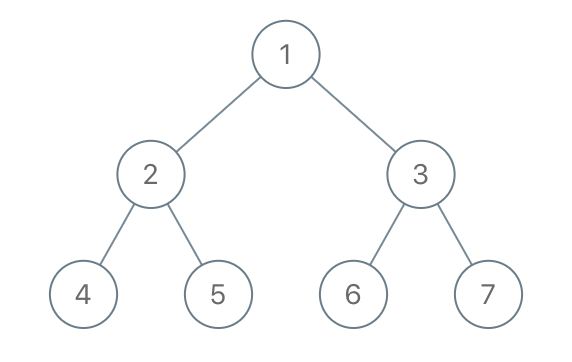 | ||
|
||
```python | ||
输入:root = [1,2,3,4,5,6,7], to_delete = [3,5] | ||
输出:[[1,2,null,4],[6],[7]] | ||
``` | ||
|
||
- 示例 2: | ||
|
||
```python | ||
输入:root = [1,2,4,null,3], to_delete = [3] | ||
输出:[[1,2,4]] | ||
``` | ||
|
||
## 解题思路 | ||
|
||
### 思路 1:深度优先搜索 | ||
|
||
将待删除节点数组 $to\underline{}delete$ 转为集合 $deletes$,则每次能以 $O(1)$ 的时间复杂度判断节点值是否在待删除节点数组中。 | ||
|
||
如果当前节点值在待删除节点数组中,则删除当前节点后,需要将其左右子树作为一棵树的节点加入到答案数组中。 | ||
|
||
而如果当前节点值不在待删除节点数组中, | ||
|
||
### 思路 1:代码 | ||
|
||
```Python | ||
class Solution: | ||
def delNodes(self, root: Optional[TreeNode], to_delete: List[int]) -> List[TreeNode]: | ||
forest = [] | ||
deletes = set(to_delete) | ||
def dfs(root): | ||
if not root: | ||
return None | ||
root.left = dfs(root.left) | ||
root.right = dfs(root.right) | ||
|
||
if root.val in deletes: | ||
if root.left: | ||
forest.append(root.left) | ||
if root.right: | ||
forest.append(root.right) | ||
return None | ||
else: | ||
return root | ||
|
||
|
||
if dfs(root): | ||
forest.append(root) | ||
return forest | ||
``` | ||
|
||
### 思路 1:复杂度分析 | ||
|
||
- **时间复杂度**:$O(n)$,其中 $n$ 为二叉树中节点个数。 | ||
- **空间复杂度**:$O(n)$。 | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,67 @@ | ||
# [1641. 统计字典序元音字符串的数目](https://leetcode.cn/problems/count-sorted-vowel-strings/) | ||
|
||
- 标签:数学、动态规划、组合数学 | ||
- 难度:中等 | ||
|
||
## 题目链接 | ||
|
||
- [1641. 统计字典序元音字符串的数目 - 力扣](https://leetcode.cn/problems/count-sorted-vowel-strings/) | ||
|
||
## 题目大意 | ||
|
||
**描述**:给定一个整数 $n$。 | ||
|
||
**要求**:返回长度为 $n$、仅由原音($a$、$e$、$i$、$o$、$u$)组成且按字典序排序的字符串数量。 | ||
|
||
**说明**: | ||
|
||
- 字符串 $a$ 按字典序排列需要满足:对于所有有效的 $i$,$s[i]$ 在字母表中的位置总是与 $s[i + 1]$ 相同或在 $s[i+1] $之前。 | ||
- $1 \le n \le 50$。 | ||
|
||
**示例**: | ||
|
||
- 示例 1: | ||
|
||
```python | ||
输入:n = 1 | ||
输出:5 | ||
解释:仅由元音组成的 5 个字典序字符串为 ["a","e","i","o","u"] | ||
``` | ||
|
||
- 示例 2: | ||
|
||
```python | ||
输入:n = 2 | ||
输出:15 | ||
解释:仅由元音组成的 15 个字典序字符串为 | ||
["aa","ae","ai","ao","au","ee","ei","eo","eu","ii","io","iu","oo","ou","uu"] | ||
注意,"ea" 不是符合题意的字符串,因为 'e' 在字母表中的位置比 'a' 靠后 | ||
``` | ||
|
||
## 解题思路 | ||
|
||
### 思路 1:组和数学 | ||
|
||
题目要求按照字典序排列,则如果确定了每个元音的出现次数可以确定一个序列。 | ||
|
||
对于长度为 $n$ 的序列,$a$、$e$、$i$、$o$、$u$ 出现次数加起来为 $n$ 次,且顺序为 $a…a \rightarrow e…e \rightarrow i…i \rightarrow o…o \rightarrow u…u$。 | ||
|
||
我们可以看作是将 $n$ 分隔成了 $5$ 份,每一份对应一个原音字母的数量。 | ||
|
||
我们可以使用「隔板法」的方式,看作有 $n$ 个球,$4$ 个板子,将 $n$ 个球分隔成 $5$ 份。 | ||
|
||
则一共有 $n + 4$ 个位置可以放板子,总共需要放 $4$ 个板子,则答案为 $C_{n + 4}^4$,其中 $C$ 为组和数。 | ||
|
||
### 思路 1:代码 | ||
|
||
```Python | ||
class Solution: | ||
def countVowelStrings(self, n: int) -> int: | ||
return comb(n + 4, 4) | ||
``` | ||
|
||
### 思路 1:复杂度分析 | ||
|
||
- **时间复杂度**:$O(| \sum |)$,其中 $\sum$ 为字符集,本题中 $| \sum | = 5$ 。 | ||
- **空间复杂度**:$O(1)$。 | ||
|