-
Notifications
You must be signed in to change notification settings - Fork 21
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
13 changed files
with
482 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
30 changes: 30 additions & 0 deletions
30
src/main/kotlin/g0501_0600/s0589_n_ary_tree_preorder_traversal/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
package g0501_0600.s0589_n_ary_tree_preorder_traversal | ||
|
||
// #Easy #Depth_First_Search #Tree #Stack #Programming_Skills_I_Day_5_Function #Level_1_Day_6_Tree | ||
// #2023_01_31_Time_233_ms_(84.02%)_Space_39.3_MB_(37.63%) | ||
|
||
import com_github_leetcode.Node | ||
|
||
/* | ||
* Definition for a Node. | ||
* class Node(var `val`: Int) { | ||
* var children: List<Node?> = listOf() | ||
* } | ||
*/ | ||
class Solution { | ||
fun preorder(root: Node?): List<Int> { | ||
val res: MutableList<Int> = ArrayList() | ||
preorderHelper(res, root) | ||
return res | ||
} | ||
|
||
private fun preorderHelper(res: MutableList<Int>, root: Node?) { | ||
if (root == null) { | ||
return | ||
} | ||
res.add(root.`val`) | ||
for (node in root.neighbors) { | ||
preorderHelper(res, node) | ||
} | ||
} | ||
} |
31 changes: 31 additions & 0 deletions
31
src/main/kotlin/g0501_0600/s0589_n_ary_tree_preorder_traversal/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
589\. N-ary Tree Preorder Traversal | ||
|
||
Easy | ||
|
||
Given the `root` of an n-ary tree, return _the preorder traversal of its nodes' values_. | ||
|
||
Nary-Tree input serialization is represented in their level order traversal. Each group of children is separated by the null value (See examples) | ||
|
||
**Example 1:** | ||
|
||
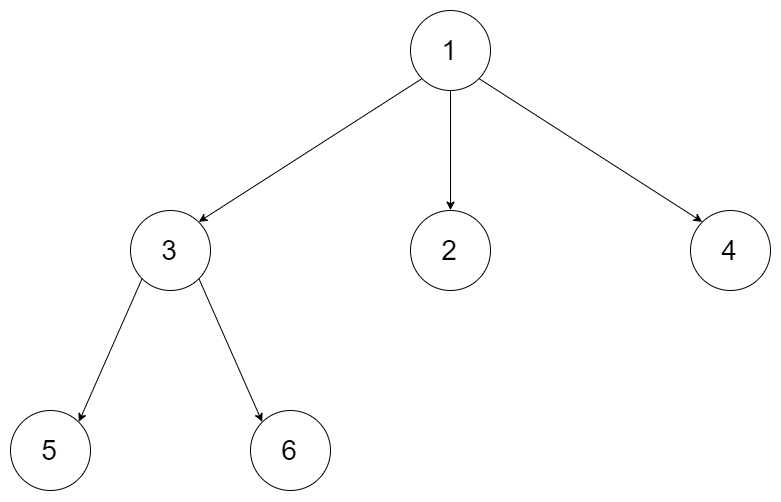 | ||
|
||
**Input:** root = [1,null,3,2,4,null,5,6] | ||
|
||
**Output:** [1,3,5,6,2,4] | ||
|
||
**Example 2:** | ||
|
||
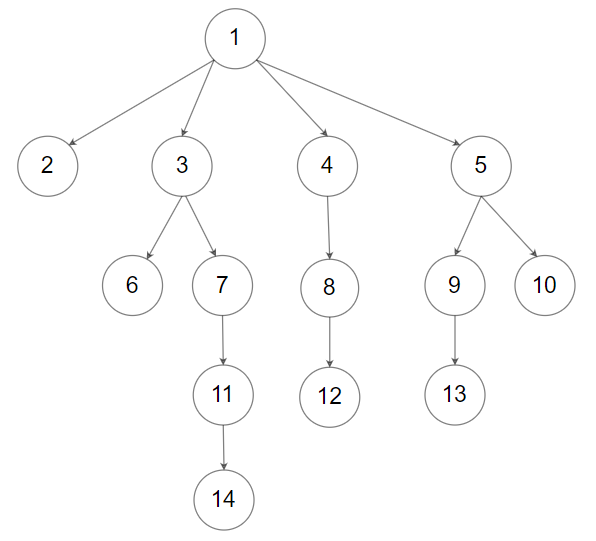 | ||
|
||
**Input:** root = [1,null,2,3,4,5,null,null,6,7,null,8,null,9,10,null,null,11,null,12,null,13,null,null,14] | ||
|
||
**Output:** [1,2,3,6,7,11,14,4,8,12,5,9,13,10] | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the tree is in the range <code>[0, 10<sup>4</sup>]</code>. | ||
* <code>0 <= Node.val <= 10<sup>4</sup></code> | ||
* The height of the n-ary tree is less than or equal to `1000`. | ||
|
||
**Follow up:** Recursive solution is trivial, could you do it iteratively? |
34 changes: 34 additions & 0 deletions
34
src/main/kotlin/g0501_0600/s0590_n_ary_tree_postorder_traversal/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
package g0501_0600.s0590_n_ary_tree_postorder_traversal | ||
|
||
// #Easy #Depth_First_Search #Tree #Stack #2023_01_31_Time_237_ms_(88.10%)_Space_38.9_MB_(76.19%) | ||
|
||
import com_github_leetcode.Node | ||
|
||
/* | ||
* Definition for a Node. | ||
* class Node(var `val`: Int) { | ||
* var children: List<Node?> = listOf() | ||
* } | ||
*/ | ||
class Solution { | ||
private var ans: ArrayList<Int>? = null | ||
|
||
fun postorder(root: Node?): List<Int> { | ||
ans = ArrayList() | ||
recursion(root) | ||
if (root != null) { | ||
ans!!.add(root.`val`) | ||
} | ||
return ans as ArrayList<Int> | ||
} | ||
|
||
private fun recursion(root: Node?) { | ||
if (root == null) { | ||
return | ||
} | ||
for (child in root.neighbors) { | ||
recursion(child) | ||
ans!!.add(child.`val`) | ||
} | ||
} | ||
} |
31 changes: 31 additions & 0 deletions
31
src/main/kotlin/g0501_0600/s0590_n_ary_tree_postorder_traversal/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
590\. N-ary Tree Postorder Traversal | ||
|
||
Easy | ||
|
||
Given the `root` of an n-ary tree, return _the postorder traversal of its nodes' values_. | ||
|
||
Nary-Tree input serialization is represented in their level order traversal. Each group of children is separated by the null value (See examples) | ||
|
||
**Example 1:** | ||
|
||
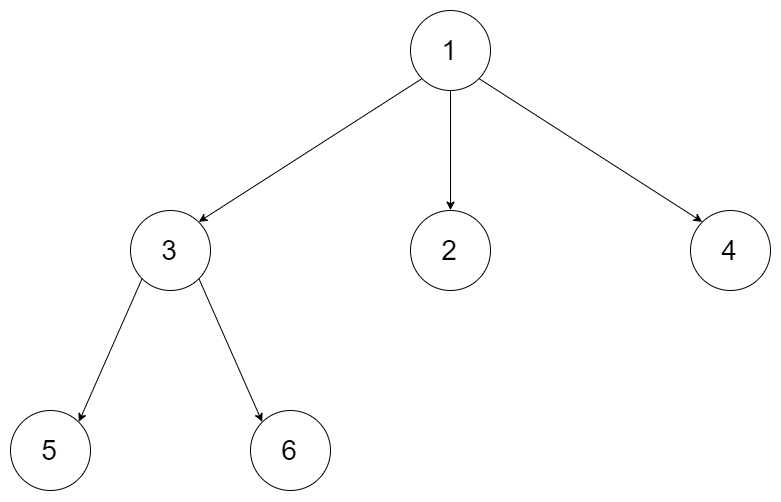 | ||
|
||
**Input:** root = [1,null,3,2,4,null,5,6] | ||
|
||
**Output:** [5,6,3,2,4,1] | ||
|
||
**Example 2:** | ||
|
||
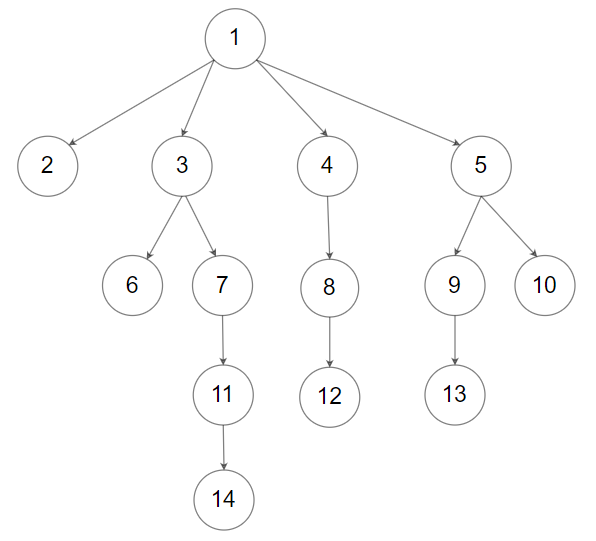 | ||
|
||
**Input:** root = [1,null,2,3,4,5,null,null,6,7,null,8,null,9,10,null,null,11,null,12,null,13,null,null,14] | ||
|
||
**Output:** [2,6,14,11,7,3,12,8,4,13,9,10,5,1] | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the tree is in the range <code>[0, 10<sup>4</sup>]</code>. | ||
* <code>0 <= Node.val <= 10<sup>4</sup></code> | ||
* The height of the n-ary tree is less than or equal to `1000`. | ||
|
||
**Follow up:** Recursive solution is trivial, could you do it iteratively? |
59 changes: 59 additions & 0 deletions
59
src/main/kotlin/g0501_0600/s0591_tag_validator/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,59 @@ | ||
package g0501_0600.s0591_tag_validator | ||
|
||
// #Hard #String #Stack #2023_01_31_Time_177_ms_(100.00%)_Space_35.4_MB_(100.00%) | ||
|
||
import java.util.ArrayDeque | ||
import java.util.Deque | ||
|
||
class Solution { | ||
fun isValid(code: String): Boolean { | ||
val stack: Deque<String> = ArrayDeque() | ||
var i = 0 | ||
while (i < code.length) { | ||
if (i > 0 && stack.isEmpty()) { | ||
return false | ||
} | ||
if (code.startsWith("<![CDATA[", i)) { | ||
// "<![CDATA[" length is 9 | ||
val j = i + 9 | ||
i = code.indexOf("]]>", j) | ||
if (i < 0) { | ||
return false | ||
} | ||
// "]]>" length is 3 | ||
i += 3 | ||
} else if (code.startsWith("</", i)) { | ||
val j = i + 2 | ||
i = code.indexOf(">", j) | ||
if (i < 0 || i == j || i - j > 9) { | ||
return false | ||
} | ||
for (k in j until i) { | ||
if (!Character.isUpperCase(code[k])) { | ||
return false | ||
} | ||
} | ||
val s = code.substring(j, i++) | ||
if (stack.isEmpty() || stack.pop() != s) { | ||
return false | ||
} | ||
} else if (code.startsWith("<", i)) { | ||
val j = i + 1 | ||
i = code.indexOf(">", j) | ||
if (i < 0 || i == j || i - j > 9) { | ||
return false | ||
} | ||
for (k in j until i) { | ||
if (!Character.isUpperCase(code[k])) { | ||
return false | ||
} | ||
} | ||
val s = code.substring(j, i++) | ||
stack.push(s) | ||
} else { | ||
i++ | ||
} | ||
} | ||
return stack.isEmpty() | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,59 @@ | ||
591\. Tag Validator | ||
|
||
Hard | ||
|
||
Given a string representing a code snippet, implement a tag validator to parse the code and return whether it is valid. | ||
|
||
A code snippet is valid if all the following rules hold: | ||
|
||
1. The code must be wrapped in a **valid closed tag**. Otherwise, the code is invalid. | ||
2. A **closed tag** (not necessarily valid) has exactly the following format : `<TAG_NAME>TAG_CONTENT</TAG_NAME>`. Among them, `<TAG_NAME>` is the start tag, and `</TAG_NAME>` is the end tag. The TAG\_NAME in start and end tags should be the same. A closed tag is **valid** if and only if the TAG\_NAME and TAG\_CONTENT are valid. | ||
3. A **valid** `TAG_NAME` only contain **upper-case letters**, and has length in range [1,9]. Otherwise, the `TAG_NAME` is **invalid**. | ||
4. A **valid** `TAG_CONTENT` may contain other **valid closed tags**, **cdata** and any characters (see note1) **EXCEPT** unmatched `<`, unmatched start and end tag, and unmatched or closed tags with invalid TAG\_NAME. Otherwise, the `TAG_CONTENT` is **invalid**. | ||
5. A start tag is unmatched if no end tag exists with the same TAG\_NAME, and vice versa. However, you also need to consider the issue of unbalanced when tags are nested. | ||
6. A `<` is unmatched if you cannot find a subsequent `>`. And when you find a `<` or `</`, all the subsequent characters until the next `>` should be parsed as TAG\_NAME (not necessarily valid). | ||
7. The cdata has the following format : `<![CDATA[CDATA_CONTENT]]>`. The range of `CDATA_CONTENT` is defined as the characters between `<![CDATA[` and the **first subsequent** `]]>`. | ||
8. `CDATA_CONTENT` may contain **any characters**. The function of cdata is to forbid the validator to parse `CDATA_CONTENT`, so even it has some characters that can be parsed as tag (no matter valid or invalid), you should treat it as **regular characters**. | ||
|
||
**Example 1:** | ||
|
||
**Input:** code = "<DIV>This is the first line <![CDATA[<div>]]></DIV>" | ||
|
||
**Output:** true | ||
|
||
**Explanation:** | ||
|
||
The code is wrapped in a closed tag : <DIV> and </DIV>. | ||
The TAG_NAME is valid, the TAG_CONTENT consists of some characters and cdata. | ||
Although CDATA_CONTENT has an unmatched start tag with invalid TAG_NAME, it should be considered as plain text, not parsed as a tag. | ||
So TAG_CONTENT is valid, and then the code is valid. Thus return true. | ||
|
||
**Example 2:** | ||
|
||
**Input:** code = "<DIV>>> ![cdata[]] <![CDATA[<div>]>]]>]]>>]</DIV>" | ||
|
||
**Output:** true | ||
|
||
**Explanation:** | ||
|
||
We first separate the code into : start_tag|tag_content|end_tag. | ||
start_tag -> "<DIV>" | ||
end_tag -> "</DIV>" | ||
tag_content could also be separated into : text1|cdata|text2. | ||
text1 -> ">> ![cdata[]] " | ||
cdata -> "<![CDATA[<div>]>]]>", where the CDATA_CONTENT is "<div>]>" | ||
text2 -> "]]>>]" The reason why start_tag is NOT "<DIV>>>" is because of the rule 6. | ||
The reason why cdata is NOT "<![CDATA[<div>]>]]>]]>" is because of the rule 7. | ||
|
||
**Example 3:** | ||
|
||
**Input:** code = "<A> <B> </A> </B>" | ||
|
||
**Output:** false | ||
|
||
**Explanation:** Unbalanced. If "<A>" is closed, then "<B>" must be unmatched, and vice versa. | ||
|
||
**Constraints:** | ||
|
||
* `1 <= code.length <= 500` | ||
* `code` consists of English letters, digits, `'<'`, `'>'`, `'/'`, `'!'`, `'['`, `']'`, `'.'`, and `' '`. |
39 changes: 39 additions & 0 deletions
39
src/main/kotlin/g0501_0600/s0592_fraction_addition_and_subtraction/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,39 @@ | ||
package g0501_0600.s0592_fraction_addition_and_subtraction | ||
|
||
// #Medium #String #Math #Simulation #2023_01_31_Time_164_ms_(100.00%)_Space_35.9_MB_(66.67%) | ||
|
||
class Solution { | ||
private fun gcd(a: Int, b: Int): Int { | ||
return if (a % b == 0) b else gcd(b, a % b) | ||
} | ||
|
||
private fun format(a: Int, b: Int): String { | ||
val gcd = Math.abs(gcd(a, b)) | ||
return (a / gcd).toString() + "/" + b / gcd | ||
} | ||
|
||
private fun parse(s: String): IntArray { | ||
val idx = s.indexOf("/") | ||
return intArrayOf(s.substring(0, idx).toInt(), s.substring(idx + 1).toInt()) | ||
} | ||
|
||
fun fractionAddition(expression: String): String { | ||
var rst = intArrayOf(0, 1) | ||
val list: MutableList<IntArray> = ArrayList() | ||
var sb = StringBuilder().append(expression[0]) | ||
for (i in 1 until expression.length) { | ||
val c = expression[i] | ||
if (c == '+' || c == '-') { | ||
list.add(parse(sb.toString())) | ||
sb = StringBuilder().append(c) | ||
} else { | ||
sb.append(c) | ||
} | ||
} | ||
list.add(parse(sb.toString())) | ||
for (num in list) { | ||
rst = intArrayOf(rst[0] * num[1] + rst[1] * num[0], rst[1] * num[1]) | ||
} | ||
return format(rst[0], rst[1]) | ||
} | ||
} |
33 changes: 33 additions & 0 deletions
33
src/main/kotlin/g0501_0600/s0592_fraction_addition_and_subtraction/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,33 @@ | ||
592\. Fraction Addition and Subtraction | ||
|
||
Medium | ||
|
||
Given a string `expression` representing an expression of fraction addition and subtraction, return the calculation result in string format. | ||
|
||
The final result should be an [irreducible fraction](https://en.wikipedia.org/wiki/Irreducible_fraction). If your final result is an integer, change it to the format of a fraction that has a denominator `1`. So in this case, `2` should be converted to `2/1`. | ||
|
||
**Example 1:** | ||
|
||
**Input:** expression = "-1/2+1/2" | ||
|
||
**Output:** "0/1" | ||
|
||
**Example 2:** | ||
|
||
**Input:** expression = "-1/2+1/2+1/3" | ||
|
||
**Output:** "1/3" | ||
|
||
**Example 3:** | ||
|
||
**Input:** expression = "1/3-1/2" | ||
|
||
**Output:** "-1/6" | ||
|
||
**Constraints:** | ||
|
||
* The input string only contains `'0'` to `'9'`, `'/'`, `'+'` and `'-'`. So does the output. | ||
* Each fraction (input and output) has the format `±numerator/denominator`. If the first input fraction or the output is positive, then `'+'` will be omitted. | ||
* The input only contains valid **irreducible fractions**, where the **numerator** and **denominator** of each fraction will always be in the range `[1, 10]`. If the denominator is `1`, it means this fraction is actually an integer in a fraction format defined above. | ||
* The number of given fractions will be in the range `[1, 10]`. | ||
* The numerator and denominator of the **final result** are guaranteed to be valid and in the range of **32-bit** int. |
Oops, something went wrong.