-
Notifications
You must be signed in to change notification settings - Fork 21
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
12 changed files
with
367 additions
and
0 deletions.
There are no files selected for viewing
58 changes: 58 additions & 0 deletions
58
src/main/kotlin/g0001_0100/s0073_set_matrix_zeroes/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,58 @@ | ||
package g0001_0100.s0073_set_matrix_zeroes | ||
|
||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Hash_Table #Matrix | ||
// #Udemy_2D_Arrays/Matrix #2022_08_31_Time_255_ms_(100.00%)_Space_45.7_MB_(91.72%) | ||
|
||
class Solution { | ||
// Approach: Use first row and first column for storing whether in future | ||
// the entire row or column needs to be marked 0 | ||
fun setZeroes(matrix: Array<IntArray>) { | ||
val m = matrix.size | ||
val n: Int = matrix[0].size | ||
var row0 = false | ||
var col0 = false | ||
// Check if 0th col needs to be market all 0s in future | ||
for (ints in matrix) { | ||
if (ints[0] == 0) { | ||
col0 = true | ||
break | ||
} | ||
} | ||
// Check if 0th row needs to be market all 0s in future | ||
for (i in 0 until n) { | ||
if (matrix[0][i] == 0) { | ||
row0 = true | ||
break | ||
} | ||
} | ||
// Store the signals in 0th row and column | ||
for (i in 1 until m) { | ||
for (j in 1 until n) { | ||
if (matrix[i][j] == 0) { | ||
matrix[i][0] = 0 | ||
matrix[0][j] = 0 | ||
} | ||
} | ||
} | ||
// Mark 0 for all cells based on signal from 0th row and 0th column | ||
for (i in 1 until m) { | ||
for (j in 1 until n) { | ||
if (matrix[i][0] == 0 || matrix[0][j] == 0) { | ||
matrix[i][j] = 0 | ||
} | ||
} | ||
} | ||
// Set 0th column | ||
for (i in 0 until m) { | ||
if (col0) { | ||
matrix[i][0] = 0 | ||
} | ||
} | ||
// Set 0th row | ||
for (i in 0 until n) { | ||
if (row0) { | ||
matrix[0][i] = 0 | ||
} | ||
} | ||
} | ||
} |
36 changes: 36 additions & 0 deletions
36
src/main/kotlin/g0001_0100/s0073_set_matrix_zeroes/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,36 @@ | ||
73\. Set Matrix Zeroes | ||
|
||
Medium | ||
|
||
Given an `m x n` integer matrix `matrix`, if an element is `0`, set its entire row and column to `0`'s. | ||
|
||
You must do it [in place](https://en.wikipedia.org/wiki/In-place_algorithm). | ||
|
||
**Example 1:** | ||
|
||
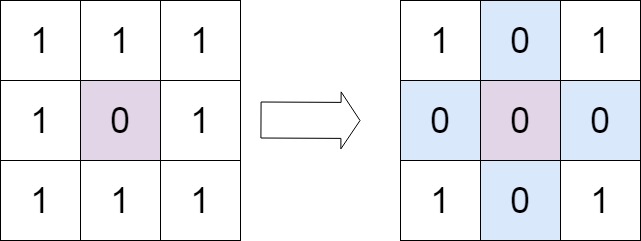 | ||
|
||
**Input:** matrix = [[1,1,1],[1,0,1],[1,1,1]] | ||
|
||
**Output:** [[1,0,1],[0,0,0],[1,0,1]] | ||
|
||
**Example 2:** | ||
|
||
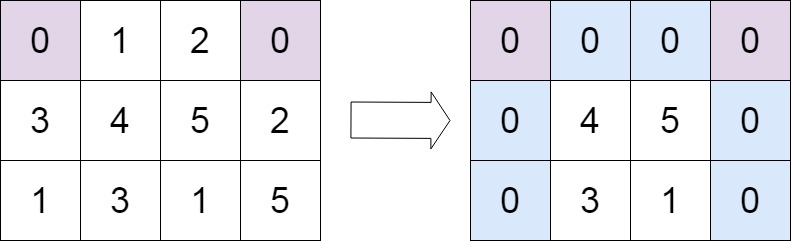 | ||
|
||
**Input:** matrix = [[0,1,2,0],[3,4,5,2],[1,3,1,5]] | ||
|
||
**Output:** [[0,0,0,0],[0,4,5,0],[0,3,1,0]] | ||
|
||
**Constraints:** | ||
|
||
* `m == matrix.length` | ||
* `n == matrix[0].length` | ||
* `1 <= m, n <= 200` | ||
* <code>-2<sup>31</sup> <= matrix[i][j] <= 2<sup>31</sup> - 1</code> | ||
|
||
**Follow up:** | ||
|
||
* A straightforward solution using `O(mn)` space is probably a bad idea. | ||
* A simple improvement uses `O(m + n)` space, but still not the best solution. | ||
* Could you devise a constant space solution? |
27 changes: 27 additions & 0 deletions
27
src/main/kotlin/g0001_0100/s0074_search_a_2d_matrix/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,27 @@ | ||
package g0001_0100.s0074_search_a_2d_matrix | ||
|
||
// #Medium #Top_100_Liked_Questions #Array #Binary_Search #Matrix #Data_Structure_I_Day_5_Array | ||
// #Algorithm_II_Day_1_Binary_Search #Binary_Search_I_Day_8 #Level_2_Day_8_Binary_Search | ||
// #Udemy_2D_Arrays/Matrix #2022_08_31_Time_290_ms_(40.17%)_Space_35.4_MB_(96.48%) | ||
|
||
class Solution { | ||
fun searchMatrix(matrix: Array<IntArray>, target: Int): Boolean { | ||
val endRow = matrix.size | ||
val endCol: Int = matrix[0].size | ||
var targetRow = 0 | ||
var result = false | ||
for (i in 0 until endRow) { | ||
if (matrix[i][endCol - 1] >= target) { | ||
targetRow = i | ||
break | ||
} | ||
} | ||
for (i in 0 until endCol) { | ||
if (matrix[targetRow][i] == target) { | ||
result = true | ||
break | ||
} | ||
} | ||
return result | ||
} | ||
} |
31 changes: 31 additions & 0 deletions
31
src/main/kotlin/g0001_0100/s0074_search_a_2d_matrix/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
74\. Search a 2D Matrix | ||
|
||
Medium | ||
|
||
Write an efficient algorithm that searches for a value `target` in an `m x n` integer matrix `matrix`. This matrix has the following properties: | ||
|
||
* Integers in each row are sorted from left to right. | ||
* The first integer of each row is greater than the last integer of the previous row. | ||
|
||
**Example 1:** | ||
|
||
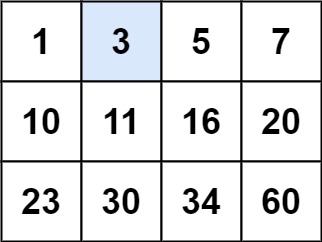 | ||
|
||
**Input:** matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]], target = 3 | ||
|
||
**Output:** true | ||
|
||
**Example 2:** | ||
|
||
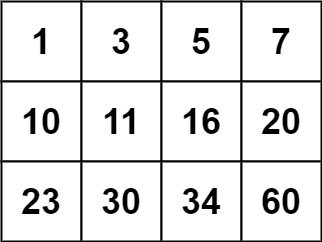 | ||
|
||
**Input:** matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]], target = 13 | ||
|
||
**Output:** false | ||
|
||
**Constraints:** | ||
|
||
* `m == matrix.length` | ||
* `n == matrix[i].length` | ||
* `1 <= m, n <= 100` | ||
* <code>-10<sup>4</sup> <= matrix[i][j], target <= 10<sup>4</sup></code> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,25 @@ | ||
package g0001_0100.s0075_sort_colors | ||
|
||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Sorting #Two_Pointers | ||
// #Data_Structure_II_Day_2_Array #Udemy_Arrays | ||
// #2022_08_31_Time_198_ms_(85.66%)_Space_34.8_MB_(84.84%) | ||
|
||
class Solution { | ||
fun sortColors(nums: IntArray) { | ||
var zeroes = 0 | ||
var ones = 0 | ||
for (i in nums.indices) { | ||
if (nums[i] == 0) { | ||
nums[zeroes++] = 0 | ||
} else if (nums[i] == 1) { | ||
ones++ | ||
} | ||
} | ||
for (j in zeroes until zeroes + ones) { | ||
nums[j] = 1 | ||
} | ||
for (k in zeroes + ones until nums.size) { | ||
nums[k] = 2 | ||
} | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,29 @@ | ||
75\. Sort Colors | ||
|
||
Medium | ||
|
||
Given an array `nums` with `n` objects colored red, white, or blue, sort them **[in-place](https://en.wikipedia.org/wiki/In-place_algorithm)** so that objects of the same color are adjacent, with the colors in the order red, white, and blue. | ||
|
||
We will use the integers `0`, `1`, and `2` to represent the color red, white, and blue, respectively. | ||
|
||
You must solve this problem without using the library's sort function. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [2,0,2,1,1,0] | ||
|
||
**Output:** [0,0,1,1,2,2] | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [2,0,1] | ||
|
||
**Output:** [0,1,2] | ||
|
||
**Constraints:** | ||
|
||
* `n == nums.length` | ||
* `1 <= n <= 300` | ||
* `nums[i]` is either `0`, `1`, or `2`. | ||
|
||
**Follow up:** Could you come up with a one-pass algorithm using only constant extra space? |
34 changes: 34 additions & 0 deletions
34
src/main/kotlin/g0001_0100/s0076_minimum_window_substring/Solution.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
package g0001_0100.s0076_minimum_window_substring | ||
|
||
// #Hard #Top_100_Liked_Questions #Top_Interview_Questions #String #Hash_Table #Sliding_Window | ||
// #Level_2_Day_14_Sliding_Window/Two_Pointer | ||
// #2022_08_31_Time_346_ms_(85.20%)_Space_39.3_MB_(93.88%) | ||
|
||
class Solution { | ||
fun minWindow(s: String, t: String): String { | ||
val map = IntArray(128) | ||
for (i in 0 until t.length) { | ||
map[t[i] - 'A']++ | ||
} | ||
var count = t.length | ||
var begin = 0 | ||
var end = 0 | ||
var d = Int.MAX_VALUE | ||
var head = 0 | ||
while (end < s.length) { | ||
if (map[s[end++] - 'A']-- > 0) { | ||
count-- | ||
} | ||
while (count == 0) { | ||
if (end - begin < d) { | ||
d = end - begin | ||
head = begin | ||
} | ||
if (map[s[begin++] - 'A']++ == 0) { | ||
count++ | ||
} | ||
} | ||
} | ||
return if (d == Int.MAX_VALUE) "" else s.substring(head, head + d) | ||
} | ||
} |
42 changes: 42 additions & 0 deletions
42
src/main/kotlin/g0001_0100/s0076_minimum_window_substring/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,42 @@ | ||
76\. Minimum Window Substring | ||
|
||
Hard | ||
|
||
Given two strings `s` and `t` of lengths `m` and `n` respectively, return _the **minimum window substring** of_ `s` _such that every character in_ `t` _(**including duplicates**) is included in the window. If there is no such substring__, return the empty string_ `""`_._ | ||
|
||
The testcases will be generated such that the answer is **unique**. | ||
|
||
A **substring** is a contiguous sequence of characters within the string. | ||
|
||
**Example 1:** | ||
|
||
**Input:** s = "ADOBECODEBANC", t = "ABC" | ||
|
||
**Output:** "BANC" | ||
|
||
**Explanation:** The minimum window substring "BANC" includes 'A', 'B', and 'C' from string t. | ||
|
||
**Example 2:** | ||
|
||
**Input:** s = "a", t = "a" | ||
|
||
**Output:** "a" | ||
|
||
**Explanation:** The entire string s is the minimum window. | ||
|
||
**Example 3:** | ||
|
||
**Input:** s = "a", t = "aa" | ||
|
||
**Output:** "" | ||
|
||
**Explanation:** Both 'a's from t must be included in the window. Since the largest window of s only has one 'a', return empty string. | ||
|
||
**Constraints:** | ||
|
||
* `m == s.length` | ||
* `n == t.length` | ||
* <code>1 <= m, n <= 10<sup>5</sup></code> | ||
* `s` and `t` consist of uppercase and lowercase English letters. | ||
|
||
**Follow up:** Could you find an algorithm that runs in `O(m + n)` time? |
23 changes: 23 additions & 0 deletions
23
src/test/kotlin/g0001_0100/s0073_set_matrix_zeroes/SolutionTest.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,23 @@ | ||
package g0001_0100.s0073_set_matrix_zeroes | ||
|
||
import org.hamcrest.CoreMatchers.equalTo | ||
import org.hamcrest.MatcherAssert.assertThat | ||
import org.junit.jupiter.api.Test | ||
|
||
internal class SolutionTest { | ||
@Test | ||
fun setZeroes() { | ||
var array = arrayOf(intArrayOf(1, 1, 1), intArrayOf(1, 0, 1), intArrayOf(1, 1, 1)) | ||
var expected = arrayOf(intArrayOf(1, 0, 1), intArrayOf(0, 0, 0), intArrayOf(1, 0, 1)) | ||
Solution().setZeroes(array) | ||
assertThat(array, equalTo(expected)) | ||
} | ||
|
||
@Test | ||
fun setZeroes2() { | ||
var array = arrayOf(intArrayOf(0, 1, 2, 0), intArrayOf(3, 4, 5, 2), intArrayOf(1, 3, 1, 5)) | ||
var expected = arrayOf(intArrayOf(0, 0, 0, 0), intArrayOf(0, 4, 5, 0), intArrayOf(0, 3, 1, 0)) | ||
Solution().setZeroes(array) | ||
assertThat(array, equalTo(expected)) | ||
} | ||
} |
17 changes: 17 additions & 0 deletions
17
src/test/kotlin/g0001_0100/s0074_search_a_2d_matrix/SolutionTest.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
package g0001_0100.s0074_search_a_2d_matrix | ||
|
||
import org.hamcrest.CoreMatchers.equalTo | ||
import org.hamcrest.MatcherAssert.assertThat | ||
import org.junit.jupiter.api.Test | ||
|
||
internal class SolutionTest { | ||
@Test | ||
fun searchMatrix() { | ||
assertThat(Solution().searchMatrix(arrayOf(intArrayOf(1, 3, 5, 7), intArrayOf(10, 11, 16, 20), intArrayOf(23, 30, 34, 60)), 3), equalTo(true)) | ||
} | ||
|
||
@Test | ||
fun searchMatrix2() { | ||
assertThat(Solution().searchMatrix(arrayOf(intArrayOf(1, 3, 5, 7), intArrayOf(10, 11, 16, 20), intArrayOf(23, 30, 34, 60)), 13), equalTo(false)) | ||
} | ||
} |
23 changes: 23 additions & 0 deletions
23
src/test/kotlin/g0001_0100/s0075_sort_colors/SolutionTest.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,23 @@ | ||
package g0001_0100.s0075_sort_colors | ||
|
||
import org.hamcrest.CoreMatchers.equalTo | ||
import org.hamcrest.MatcherAssert.assertThat | ||
import org.junit.jupiter.api.Test | ||
|
||
internal class SolutionTest { | ||
@Test | ||
fun sortColors() { | ||
var array = intArrayOf(2, 0, 2, 1, 1, 0) | ||
Solution().sortColors(array) | ||
var expected = intArrayOf(0, 0, 1, 1, 2, 2) | ||
assertThat(array, equalTo(expected)) | ||
} | ||
|
||
@Test | ||
fun sortColors2() { | ||
var array = intArrayOf(2, 0, 1) | ||
Solution().sortColors(array) | ||
var expected = intArrayOf(0, 1, 2) | ||
assertThat(array, equalTo(expected)) | ||
} | ||
} |
22 changes: 22 additions & 0 deletions
22
src/test/kotlin/g0001_0100/s0076_minimum_window_substring/SolutionTest.kt
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,22 @@ | ||
package g0001_0100.s0076_minimum_window_substring | ||
|
||
import org.hamcrest.CoreMatchers.equalTo | ||
import org.hamcrest.MatcherAssert.assertThat | ||
import org.junit.jupiter.api.Test | ||
|
||
internal class SolutionTest { | ||
@Test | ||
fun minWindow() { | ||
assertThat(Solution().minWindow("ADOBECODEBANC", "ABC"), equalTo("BANC")) | ||
} | ||
|
||
@Test | ||
fun minWindow2() { | ||
assertThat(Solution().minWindow("a", "a"), equalTo("a")) | ||
} | ||
|
||
@Test | ||
fun minWindow3() { | ||
assertThat(Solution().minWindow("a", "aa"), equalTo("")) | ||
} | ||
} |