forked from LeetCode-in-Net/LeetCode-in-Net
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
16 changed files
with
518 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,33 @@ | ||
namespace LeetCodeNet.Com_github_leetcode { | ||
|
||
public class TreeUtils { | ||
/* | ||
* This method is to construct a normal binary tree. The input reads like | ||
* this for [5, 3, 6, 2, 4, null, null, 1], i.e. preorder: | ||
5 | ||
/ \ | ||
3 6 | ||
/ \ / \ | ||
2 4 # # | ||
/ | ||
1 | ||
*/ | ||
public static TreeNode ConstructBinaryTree(List<int?> treeValues) { | ||
TreeNode root = new TreeNode(treeValues[0]); | ||
Queue<TreeNode> queue = new Queue<TreeNode>(); | ||
queue.Enqueue(root); | ||
for (int i = 1; i < treeValues.Count; i++) { | ||
TreeNode curr = queue.Dequeue(); | ||
if (treeValues[i] != null) { | ||
curr.left = new TreeNode(treeValues[i]); | ||
queue.Enqueue(curr.left); | ||
} | ||
if (++i < treeValues.Count && treeValues[i] != null) { | ||
curr.right = new TreeNode(treeValues[i]); | ||
queue.Enqueue(curr.right); | ||
} | ||
} | ||
return root; | ||
} | ||
} | ||
} |
25 changes: 25 additions & 0 deletions
25
LeetCodeNet.Tests/G0101_0200/S0102_binary_tree_level_order_traversal/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,25 @@ | ||
namespace LeetCodeNet.G0101_0200.S0102_binary_tree_level_order_traversal { | ||
|
||
using System; | ||
using Xunit; | ||
using Com_github_leetcode; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void LevelOrder1() { | ||
TreeNode root = TreeUtils.ConstructBinaryTree(new List<int?> { 3, 9, 20, null, null, 15, 7 }); | ||
Assert.Equal(new List<IList<int>> { new List<int> { 3 }, new List<int> { 9, 20 }, new List<int> { 15, 7 } }, new Solution().LevelOrder(root)); | ||
} | ||
|
||
[Fact] | ||
public void LevelOrder2() { | ||
TreeNode root = TreeUtils.ConstructBinaryTree(new List<int?> { 1 }); | ||
Assert.Equal(new List<IList<int>> { new List<int> { 1 } }, new Solution().LevelOrder(root)); | ||
} | ||
|
||
[Fact] | ||
public void LevelOrder3() { | ||
Assert.Equal(new List<IList<int>> { }, new Solution().LevelOrder(null)); | ||
} | ||
} | ||
} |
20 changes: 20 additions & 0 deletions
20
LeetCodeNet.Tests/G0101_0200/S0104_maximum_depth_of_binary_tree/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,20 @@ | ||
namespace LeetCodeNet.G0101_0200.S0104_maximum_depth_of_binary_tree { | ||
|
||
using System; | ||
using Xunit; | ||
using Com_github_leetcode; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void MaxDepth() { | ||
TreeNode root = TreeUtils.ConstructBinaryTree(new List<int?> { 3, 9, 20, null, null, 15, 7 }); | ||
Assert.Equal(3, new Solution().MaxDepth(root)); | ||
} | ||
|
||
[Fact] | ||
public void MaxDepth2() { | ||
TreeNode root = TreeUtils.ConstructBinaryTree(new List<int?> { 1, null, 2 }); | ||
Assert.Equal(2, new Solution().MaxDepth(root)); | ||
} | ||
} | ||
} |
25 changes: 25 additions & 0 deletions
25
...0101_0200/S0105_construct_binary_tree_from_preorder_and_inorder_traversal/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,25 @@ | ||
namespace LeetCodeNet.G0101_0200.S0105_construct_binary_tree_from_preorder_and_inorder_traversal { | ||
|
||
using System; | ||
using Xunit; | ||
using LeetCodeNet.Com_github_leetcode; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void BuildTree() { | ||
int[] preorder = { 3, 9, 20, 15, 7 }; | ||
int[] inorder = { 9, 3, 15, 20, 7 }; | ||
TreeNode actual = new Solution().BuildTree(preorder, inorder); | ||
Assert.Equal("3,9,20,15,7", actual.ToString()); | ||
} | ||
|
||
[Fact] | ||
public void BuildTree2() { | ||
int[] preorder = { -1 }; | ||
int[] inorder = { -1 }; | ||
TreeNode actual = new Solution().BuildTree(preorder, inorder); | ||
Assert.Equal("-1", actual.ToString()); | ||
} | ||
|
||
} | ||
} |
22 changes: 22 additions & 0 deletions
22
LeetCodeNet.Tests/G0101_0200/S0114_flatten_binary_tree_to_linked_list/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,22 @@ | ||
namespace LeetCodeNet.G0101_0200.S0114_flatten_binary_tree_to_linked_list { | ||
|
||
using System; | ||
using Xunit; | ||
using LeetCodeNet.Com_github_leetcode; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void Flatten() { | ||
TreeNode root = TreeUtils.ConstructBinaryTree(new List<int?> { 1, 2, 5, 3, 4, null, 6 }); | ||
new Solution().Flatten(root); | ||
Assert.Equal("1,null,2,null,3,null,4,null,5,null,6", root.ToString()); | ||
} | ||
|
||
[Fact] | ||
public void Flatten2() { | ||
TreeNode root = TreeUtils.ConstructBinaryTree(new List<int?> { 0 }); | ||
new Solution().Flatten(root); | ||
Assert.Equal("0", root.ToString()); | ||
} | ||
} | ||
} |
17 changes: 17 additions & 0 deletions
17
LeetCodeNet.Tests/G0101_0200/S0121_best_time_to_buy_and_sell_stock/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
namespace LeetCodeNet.G0101_0200.S0121_best_time_to_buy_and_sell_stock { | ||
|
||
using System; | ||
using Xunit; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void MaxProfit() { | ||
Assert.Equal(5, new Solution().MaxProfit(new int[] { 7, 1, 5, 3, 6, 4 })); | ||
} | ||
|
||
[Fact] | ||
public void MaxProfit2() { | ||
Assert.Equal(0, new Solution().MaxProfit(new int[] { 7, 6, 4, 3, 1 })); | ||
} | ||
} | ||
} |
53 changes: 53 additions & 0 deletions
53
LeetCodeNet/G0101_0200/S0102_binary_tree_level_order_traversal/Solution.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,53 @@ | ||
namespace LeetCodeNet.G0101_0200.S0102_binary_tree_level_order_traversal { | ||
|
||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Breadth_First_Search #Tree | ||
// #Binary_Tree #Data_Structure_I_Day_11_Tree #Level_1_Day_6_Tree #Udemy_Tree_Stack_Queue | ||
// #Big_O_Time_O(N)_Space_O(N) #2024_01_09_Time_97_ms_(98.14%)_Space_47.4_MB_(12.94%) | ||
|
||
using LeetCodeNet.Com_github_leetcode; | ||
|
||
/** | ||
* Definition for a binary tree node. | ||
* public class TreeNode { | ||
* public int val; | ||
* public TreeNode left; | ||
* public TreeNode right; | ||
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) { | ||
* this.val = val; | ||
* this.left = left; | ||
* this.right = right; | ||
* } | ||
* } | ||
*/ | ||
public class Solution { | ||
public IList<IList<int>> LevelOrder(TreeNode root) { | ||
IList<IList<int>> result = new List<IList<int>>(); | ||
if (root == null) { | ||
return result; | ||
} | ||
Queue<TreeNode> queue = new Queue<TreeNode>(); | ||
queue.Enqueue(root); | ||
queue.Enqueue(null); | ||
List<int> level = new List<int>(); | ||
while (queue.Count > 0) { | ||
root = queue.Dequeue(); | ||
while (queue.Count > 0 && root != null) { | ||
level.Add((int)root.val); | ||
if (root.left != null) { | ||
queue.Enqueue(root.left); | ||
} | ||
if (root.right != null) { | ||
queue.Enqueue(root.right); | ||
} | ||
root = queue.Dequeue(); | ||
} | ||
result.Add(level); | ||
level = new List<int>(); | ||
if (queue.Count > 0) { | ||
queue.Enqueue(null); | ||
} | ||
} | ||
return result; | ||
} | ||
} | ||
} |
30 changes: 30 additions & 0 deletions
30
LeetCodeNet/G0101_0200/S0102_binary_tree_level_order_traversal/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
102\. Binary Tree Level Order Traversal | ||
|
||
Medium | ||
|
||
Given the `root` of a binary tree, return _the level order traversal of its nodes' values_. (i.e., from left to right, level by level). | ||
|
||
**Example 1:** | ||
|
||
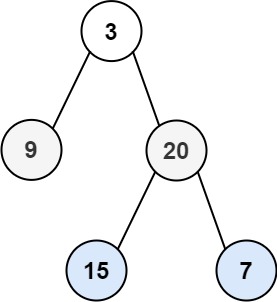 | ||
|
||
**Input:** root = [3,9,20,null,null,15,7] | ||
|
||
**Output:** [[3],[9,20],[15,7]] | ||
|
||
**Example 2:** | ||
|
||
**Input:** root = [1] | ||
|
||
**Output:** [[1]] | ||
|
||
**Example 3:** | ||
|
||
**Input:** root = [] | ||
|
||
**Output:** [] | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the tree is in the range `[0, 2000]`. | ||
* `-1000 <= Node.val <= 1000` |
37 changes: 37 additions & 0 deletions
37
LeetCodeNet/G0101_0200/S0104_maximum_depth_of_binary_tree/Solution.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,37 @@ | ||
namespace LeetCodeNet.G0101_0200.S0104_maximum_depth_of_binary_tree { | ||
|
||
// #Easy #Top_100_Liked_Questions #Top_Interview_Questions #Depth_First_Search #Breadth_First_Search | ||
// #Tree #Binary_Tree #Data_Structure_I_Day_11_Tree | ||
// #Programming_Skills_I_Day_10_Linked_List_and_Tree #Udemy_Tree_Stack_Queue | ||
// #Big_O_Time_O(N)_Space_O(H) #2024_01_09_Time_65_ms_(93.31%)_Space_42.3_MB_(9.74%) | ||
|
||
using LeetCodeNet.Com_github_leetcode; | ||
|
||
/** | ||
* Definition for a binary tree node. | ||
* public class TreeNode { | ||
* public int val; | ||
* public TreeNode left; | ||
* public TreeNode right; | ||
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) { | ||
* this.val = val; | ||
* this.left = left; | ||
* this.right = right; | ||
* } | ||
* } | ||
*/ | ||
public class Solution { | ||
public int MaxDepth(TreeNode root) { | ||
return FindDepth(root, 0); | ||
} | ||
|
||
private int FindDepth(TreeNode node, int currentDepth) { | ||
if (node == null) { | ||
return 0; | ||
} | ||
currentDepth++; | ||
return 1 | ||
+ Math.Max(FindDepth(node.left, currentDepth), FindDepth(node.right, currentDepth)); | ||
} | ||
} | ||
} |
38 changes: 38 additions & 0 deletions
38
LeetCodeNet/G0101_0200/S0104_maximum_depth_of_binary_tree/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,38 @@ | ||
104\. Maximum Depth of Binary Tree | ||
|
||
Easy | ||
|
||
Given the `root` of a binary tree, return _its maximum depth_. | ||
|
||
A binary tree's **maximum depth** is the number of nodes along the longest path from the root node down to the farthest leaf node. | ||
|
||
**Example 1:** | ||
|
||
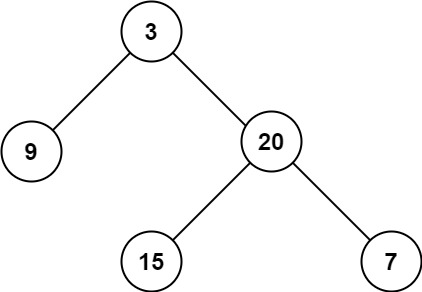 | ||
|
||
**Input:** root = [3,9,20,null,null,15,7] | ||
|
||
**Output:** 3 | ||
|
||
**Example 2:** | ||
|
||
**Input:** root = [1,null,2] | ||
|
||
**Output:** 2 | ||
|
||
**Example 3:** | ||
|
||
**Input:** root = [] | ||
|
||
**Output:** 0 | ||
|
||
**Example 4:** | ||
|
||
**Input:** root = [0] | ||
|
||
**Output:** 1 | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the tree is in the range <code>[0, 10<sup>4</sup>]</code>. | ||
* `-100 <= Node.val <= 100` |
50 changes: 50 additions & 0 deletions
50
...et/G0101_0200/S0105_construct_binary_tree_from_preorder_and_inorder_traversal/Solution.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,50 @@ | ||
namespace LeetCodeNet.G0101_0200.S0105_construct_binary_tree_from_preorder_and_inorder_traversal { | ||
|
||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Hash_Table #Tree #Binary_Tree | ||
// #Divide_and_Conquer #Data_Structure_II_Day_15_Tree #Big_O_Time_O(N)_Space_O(N) | ||
// #2024_01_09_Time_53_ms_(99.83%)_Space_42.5_MB_(46.06%) | ||
|
||
using LeetCodeNet.Com_github_leetcode; | ||
|
||
/** | ||
* Definition for a binary tree node. | ||
* public class TreeNode { | ||
* public int val; | ||
* public TreeNode left; | ||
* public TreeNode right; | ||
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) { | ||
* this.val = val; | ||
* this.left = left; | ||
* this.right = right; | ||
* } | ||
* } | ||
*/ | ||
public class Solution { | ||
private int j; | ||
private Dictionary<int, int> map = new Dictionary<int, int>(); | ||
|
||
public int Get(int key) { | ||
return map[key]; | ||
} | ||
|
||
private TreeNode Answer(int[] preorder, int[] inorder, int start, int end) { | ||
if (start > end || j > preorder.Length) { | ||
return null; | ||
} | ||
int value = preorder[j++]; | ||
int index = Get(value); | ||
TreeNode node = new TreeNode(value); | ||
node.left = Answer(preorder, inorder, start, index - 1); | ||
node.right = Answer(preorder, inorder, index + 1, end); | ||
return node; | ||
} | ||
|
||
public TreeNode BuildTree(int[] preorder, int[] inorder) { | ||
j = 0; | ||
for (int i = 0; i < preorder.Length; i++) { | ||
map.Add(inorder[i], i); | ||
} | ||
return Answer(preorder, inorder, 0, preorder.Length - 1); | ||
} | ||
} | ||
} |
29 changes: 29 additions & 0 deletions
29
..._0200/S0105_construct_binary_tree_from_preorder_and_inorder_traversal/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,29 @@ | ||
105\. Construct Binary Tree from Preorder and Inorder Traversal | ||
|
||
Medium | ||
|
||
Given two integer arrays `preorder` and `inorder` where `preorder` is the preorder traversal of a binary tree and `inorder` is the inorder traversal of the same tree, construct and return _the binary tree_. | ||
|
||
**Example 1:** | ||
|
||
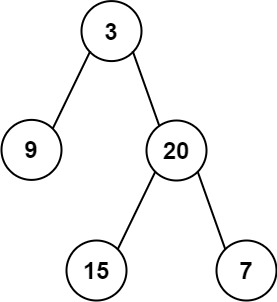 | ||
|
||
**Input:** preorder = [3,9,20,15,7], inorder = [9,3,15,20,7] | ||
|
||
**Output:** [3,9,20,null,null,15,7] | ||
|
||
**Example 2:** | ||
|
||
**Input:** preorder = [-1], inorder = [-1] | ||
|
||
**Output:** [-1] | ||
|
||
**Constraints:** | ||
|
||
* `1 <= preorder.length <= 3000` | ||
* `inorder.length == preorder.length` | ||
* `-3000 <= preorder[i], inorder[i] <= 3000` | ||
* `preorder` and `inorder` consist of **unique** values. | ||
* Each value of `inorder` also appears in `preorder`. | ||
* `preorder` is **guaranteed** to be the preorder traversal of the tree. | ||
* `inorder` is **guaranteed** to be the inorder traversal of the tree. |
Oops, something went wrong.