forked from LeetCode-in-Scala/LeetCode-in-Scala
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
31 changed files
with
1,039 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
72 changes: 72 additions & 0 deletions
72
src/main/scala/g0001_0100/s0023_merge_k_sorted_lists/Solution.scala
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,72 @@ | ||
package g0001_0100.s0023_merge_k_sorted_lists | ||
|
||
// #Hard #Top_100_Liked_Questions #Top_Interview_Questions #Heap_Priority_Queue #Linked_List | ||
// #Divide_and_Conquer #Merge_Sort #Big_O_Time_O(k*n*log(k))_Space_O(log(k)) | ||
// #2023_10_30_Time_549_ms_(94.74%)_Space_59.6_MB_(89.47%) | ||
|
||
import com_github_leetcode.ListNode | ||
|
||
/* | ||
* Definition for singly-linked list. | ||
* class ListNode(_x: Int = 0, _next: ListNode = null) { | ||
* var next: ListNode = _next | ||
* var x: Int = _x | ||
* } | ||
*/ | ||
object Solution { | ||
def mergeKLists(lists: Array[ListNode]): ListNode = { | ||
if (lists.isEmpty) { | ||
null | ||
} else { | ||
mergeKLists(lists, 0, lists.length) | ||
} | ||
} | ||
|
||
def mergeKLists(lists: Array[ListNode], leftIndex: Int, rightIndex: Int): ListNode = { | ||
if (rightIndex > leftIndex + 1) { | ||
val mid = (leftIndex + rightIndex) / 2 | ||
val left = mergeKLists(lists, leftIndex, mid) | ||
val right = mergeKLists(lists, mid, rightIndex) | ||
mergeTwoLists(left, right) | ||
} else { | ||
lists(leftIndex) | ||
} | ||
} | ||
|
||
@SuppressWarnings(Array("scala:S1871")) | ||
def mergeTwoLists(left: ListNode, right: ListNode): ListNode = { | ||
if (left == null) { | ||
right | ||
} else if (right == null) { | ||
left | ||
} else { | ||
var res: ListNode = null | ||
var (l, r) = (left, right) | ||
if (l.x <= r.x) { | ||
res = l | ||
l = l.next | ||
} else { | ||
res = r | ||
r = r.next | ||
} | ||
var node = res | ||
while (l != null || r != null) { | ||
if (l == null) { | ||
node.next = r | ||
r = r.next | ||
} else if (r == null) { | ||
node.next = l | ||
l = l.next | ||
} else if (l.x <= r.x) { | ||
node.next = l | ||
l = l.next | ||
} else { | ||
node.next = r | ||
r = r.next | ||
} | ||
node = node.next | ||
} | ||
res | ||
} | ||
} | ||
} |
36 changes: 36 additions & 0 deletions
36
src/main/scala/g0001_0100/s0023_merge_k_sorted_lists/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,36 @@ | ||
23\. Merge k Sorted Lists | ||
|
||
Hard | ||
|
||
You are given an array of `k` linked-lists `lists`, each linked-list is sorted in ascending order. | ||
|
||
_Merge all the linked-lists into one sorted linked-list and return it._ | ||
|
||
**Example 1:** | ||
|
||
**Input:** lists = [[1,4,5],[1,3,4],[2,6]] | ||
|
||
**Output:** [1,1,2,3,4,4,5,6] | ||
|
||
**Explanation:** The linked-lists are: [ 1->4->5, 1->3->4, 2->6 ] merging them into one sorted list: 1->1->2->3->4->4->5->6 | ||
|
||
**Example 2:** | ||
|
||
**Input:** lists = [] | ||
|
||
**Output:** [] | ||
|
||
**Example 3:** | ||
|
||
**Input:** lists = [[]] | ||
|
||
**Output:** [] | ||
|
||
**Constraints:** | ||
|
||
* `k == lists.length` | ||
* `0 <= k <= 10^4` | ||
* `0 <= lists[i].length <= 500` | ||
* `-10^4 <= lists[i][j] <= 10^4` | ||
* `lists[i]` is sorted in **ascending order**. | ||
* The sum of `lists[i].length` won't exceed `10^4`. |
51 changes: 51 additions & 0 deletions
51
src/main/scala/g0001_0100/s0024_swap_nodes_in_pairs/Solution.scala
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,51 @@ | ||
package g0001_0100.s0024_swap_nodes_in_pairs | ||
|
||
// #Medium #Top_100_Liked_Questions #Linked_List #Recursion #Data_Structure_II_Day_12_Linked_List | ||
// #Udemy_Linked_List #Big_O_Time_O(n)_Space_O(1) | ||
// #2023_10_30_Time_441_ms_(95.65%)_Space_56.9_MB_(52.17%) | ||
|
||
import com_github_leetcode.ListNode | ||
|
||
/* | ||
* Definition for singly-linked list. | ||
* class ListNode(_x: Int = 0, _next: ListNode = null) { | ||
* var next: ListNode = _next | ||
* var x: Int = _x | ||
* } | ||
*/ | ||
object Solution { | ||
def swapPairs(head: ListNode): ListNode = { | ||
if (head == null) { | ||
return null | ||
} | ||
val len = getLength(head) | ||
reverse(head, len) | ||
} | ||
|
||
private def getLength(curr: ListNode): Int = { | ||
var cnt = 0 | ||
var current = curr | ||
while (current != null) { | ||
cnt += 1 | ||
current = current.next | ||
} | ||
cnt | ||
} | ||
|
||
private def reverse(head: ListNode, len: Int): ListNode = { | ||
if (len < 2) { | ||
return head | ||
} | ||
var curr = head | ||
var prev: ListNode = null | ||
var next: ListNode = null | ||
for (i <- 0 until 2) { | ||
next = curr.next | ||
curr.next = prev | ||
prev = curr | ||
curr = next | ||
} | ||
head.next = reverse(curr, len - 2) | ||
prev | ||
} | ||
} |
30 changes: 30 additions & 0 deletions
30
src/main/scala/g0001_0100/s0024_swap_nodes_in_pairs/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
24\. Swap Nodes in Pairs | ||
|
||
Medium | ||
|
||
Given a linked list, swap every two adjacent nodes and return its head. You must solve the problem without modifying the values in the list's nodes (i.e., only nodes themselves may be changed.) | ||
|
||
**Example 1:** | ||
|
||
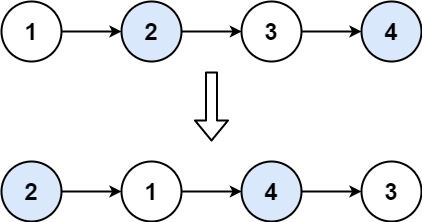 | ||
|
||
**Input:** head = [1,2,3,4] | ||
|
||
**Output:** [2,1,4,3] | ||
|
||
**Example 2:** | ||
|
||
**Input:** head = [] | ||
|
||
**Output:** [] | ||
|
||
**Example 3:** | ||
|
||
**Input:** head = [1] | ||
|
||
**Output:** [1] | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the list is in the range `[0, 100]`. | ||
* `0 <= Node.val <= 100` |
48 changes: 48 additions & 0 deletions
48
src/main/scala/g0001_0100/s0025_reverse_nodes_in_k_group/Solution.scala
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,48 @@ | ||
package g0001_0100.s0025_reverse_nodes_in_k_group | ||
|
||
// #Hard #Top_100_Liked_Questions #Linked_List #Recursion #Data_Structure_II_Day_13_Linked_List | ||
// #Udemy_Linked_List #Big_O_Time_O(n)_Space_O(k) | ||
// #2023_10_30_Time_520_ms_(80.00%)_Space_58_MB_(50.00%) | ||
|
||
import com_github_leetcode.ListNode | ||
|
||
/* | ||
* Definition for singly-linked list. | ||
* class ListNode(_x: Int = 0, _next: ListNode = null) { | ||
* var next: ListNode = _next | ||
* var x: Int = _x | ||
* } | ||
*/ | ||
object Solution { | ||
def reverseKGroup(head: ListNode, k: Int): ListNode = { | ||
if (head == null || head.next == null || k == 1) { | ||
return head | ||
} | ||
var j = 0 | ||
var len = head | ||
// Loop for checking the length of the linked list; if the linked list is less than k, then return as it is. | ||
while (j < k) { | ||
if (len == null) { | ||
return head | ||
} | ||
len = len.next | ||
j += 1 | ||
} | ||
// Reverse linked list logic applied here. | ||
var c = head | ||
var n: ListNode = null | ||
var prev: ListNode = null | ||
var i = 0 | ||
// Traverse the while loop for K times to reverse the nodes in K groups. | ||
while (i != k) { | ||
n = c.next | ||
c.next = prev | ||
prev = c | ||
c = n | ||
i += 1 | ||
} | ||
// head.x is pointing to the next K group linked list; recursion for further remaining linked list. | ||
head.next = reverseKGroup(n, k) | ||
prev | ||
} | ||
} |
Oops, something went wrong.