forked from LeetCode-in-Scala/LeetCode-in-Scala
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
33 changed files
with
968 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,42 @@ | ||
package com_github_leetcode | ||
|
||
import scala.collection.mutable | ||
|
||
class TreeNode(var value: Int, var left: TreeNode = null, var right: TreeNode = null) { | ||
override def toString: String = { | ||
if (left == null && right == null) { | ||
value.toString | ||
} else { | ||
val root = value.toString | ||
val leftValue = Option(left).map(_.toString).getOrElse("null") | ||
val rightValue = Option(right).map(_.toString).getOrElse("null") | ||
s"$root,$leftValue,$rightValue" | ||
} | ||
} | ||
} | ||
|
||
object TreeNode { | ||
def create(treeValues: List[Option[Int]]): TreeNode = { | ||
if (treeValues.isEmpty) { | ||
null | ||
} else { | ||
val root = new TreeNode(treeValues.head.getOrElse(0)) | ||
val queue = mutable.Queue(root) | ||
var i = 1 | ||
while (i < treeValues.length) { | ||
val curr = queue.dequeue() | ||
if (treeValues(i).isDefined) { | ||
curr.left = new TreeNode(treeValues(i).get) | ||
queue.enqueue(curr.left) | ||
} | ||
i += 1 | ||
if (i < treeValues.length && treeValues(i).isDefined) { | ||
curr.right = new TreeNode(treeValues(i).get) | ||
queue.enqueue(curr.right) | ||
} | ||
i += 1 | ||
} | ||
root | ||
} | ||
} | ||
} |
41 changes: 41 additions & 0 deletions
41
src/main/scala/g0001_0100/s0094_binary_tree_inorder_traversal/Solution.scala
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,41 @@ | ||
package g0001_0100.s0094_binary_tree_inorder_traversal | ||
|
||
// #Easy #Top_100_Liked_Questions #Top_Interview_Questions #Depth_First_Search #Tree #Binary_Tree | ||
// #Stack #Data_Structure_I_Day_10_Tree #Udemy_Tree_Stack_Queue #Big_O_Time_O(n)_Space_O(n) | ||
// #2023_11_03_Time_456_ms_(68.42%)_Space_57.3_MB_(7.89%) | ||
|
||
import com_github_leetcode.TreeNode | ||
import scala.collection.mutable.ListBuffer | ||
|
||
/* | ||
* Definition for a binary tree node. | ||
* class TreeNode(_value: Int = 0, _left: TreeNode = null, _right: TreeNode = null) { | ||
* var value: Int = _value | ||
* var left: TreeNode = _left | ||
* var right: TreeNode = _right | ||
* } | ||
*/ | ||
object Solution { | ||
def inorderTraversal(root: TreeNode): List[Int] = { | ||
if (root == null) { | ||
List.empty | ||
} else { | ||
val answer = ListBuffer[Int]() | ||
inorderTraversal(root, answer) | ||
answer.toList | ||
} | ||
} | ||
|
||
private def inorderTraversal(root: TreeNode, answer: ListBuffer[Int]): Unit = { | ||
if (root == null) { | ||
return | ||
} | ||
if (root.left != null) { | ||
inorderTraversal(root.left, answer) | ||
} | ||
answer += root.value | ||
if (root.right != null) { | ||
inorderTraversal(root.right, answer) | ||
} | ||
} | ||
} |
48 changes: 48 additions & 0 deletions
48
src/main/scala/g0001_0100/s0094_binary_tree_inorder_traversal/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,48 @@ | ||
94\. Binary Tree Inorder Traversal | ||
|
||
Easy | ||
|
||
Given the `root` of a binary tree, return _the inorder traversal of its nodes' values_. | ||
|
||
**Example 1:** | ||
|
||
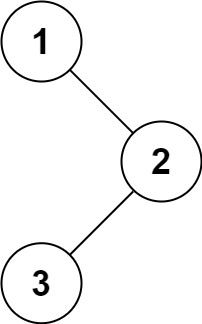 | ||
|
||
**Input:** root = [1,null,2,3] | ||
|
||
**Output:** [1,3,2] | ||
|
||
**Example 2:** | ||
|
||
**Input:** root = [] | ||
|
||
**Output:** [] | ||
|
||
**Example 3:** | ||
|
||
**Input:** root = [1] | ||
|
||
**Output:** [1] | ||
|
||
**Example 4:** | ||
|
||
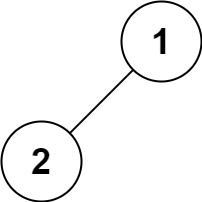 | ||
|
||
**Input:** root = [1,2] | ||
|
||
**Output:** [2,1] | ||
|
||
**Example 5:** | ||
|
||
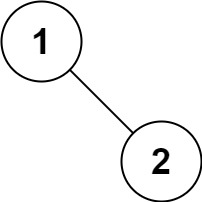 | ||
|
||
**Input:** root = [1,null,2] | ||
|
||
**Output:** [1,2] | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the tree is in the range `[0, 100]`. | ||
* `-100 <= Node.val <= 100` | ||
|
||
**Follow up:** Recursive solution is trivial, could you do it iteratively? |
17 changes: 17 additions & 0 deletions
17
src/main/scala/g0001_0100/s0096_unique_binary_search_trees/Solution.scala
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
package g0001_0100.s0096_unique_binary_search_trees | ||
|
||
// #Medium #Top_100_Liked_Questions #Dynamic_Programming #Math #Tree #Binary_Tree | ||
// #Binary_Search_Tree #Dynamic_Programming_I_Day_11 #Big_O_Time_O(n)_Space_O(1) | ||
// #2023_11_03_Time_403_ms_(66.67%)_Space_51.5_MB_(100.00%) | ||
|
||
object Solution { | ||
def numTrees(n: Int): Int = { | ||
var result: Long = 1L | ||
for (i <- 0 until n) { | ||
result *= 2L * n - i | ||
result /= i + 1 | ||
} | ||
result /= (n + 1) | ||
result.toInt | ||
} | ||
} |
23 changes: 23 additions & 0 deletions
23
src/main/scala/g0001_0100/s0096_unique_binary_search_trees/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,23 @@ | ||
96\. Unique Binary Search Trees | ||
|
||
Medium | ||
|
||
Given an integer `n`, return _the number of structurally unique **BST'**s (binary search trees) which has exactly_ `n` _nodes of unique values from_ `1` _to_ `n`. | ||
|
||
**Example 1:** | ||
|
||
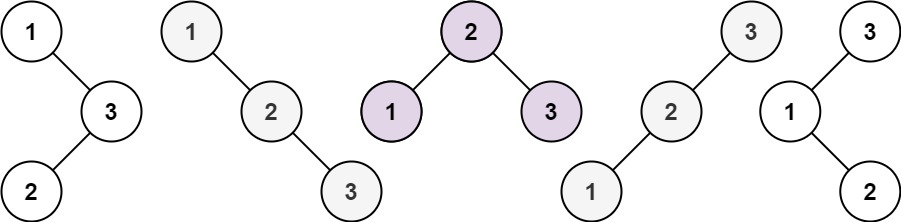 | ||
|
||
**Input:** n = 3 | ||
|
||
**Output:** 5 | ||
|
||
**Example 2:** | ||
|
||
**Input:** n = 1 | ||
|
||
**Output:** 1 | ||
|
||
**Constraints:** | ||
|
||
* `1 <= n <= 19` |
32 changes: 32 additions & 0 deletions
32
src/main/scala/g0001_0100/s0098_validate_binary_search_tree/Solution.scala
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,32 @@ | ||
package g0001_0100.s0098_validate_binary_search_tree | ||
|
||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Depth_First_Search #Tree #Binary_Tree | ||
// #Binary_Search_Tree #Data_Structure_I_Day_14_Tree #Level_1_Day_8_Binary_Search_Tree | ||
// #Udemy_Tree_Stack_Queue #Big_O_Time_O(N)_Space_O(log(N)) | ||
// #2023_11_03_Time_507_ms_(70.21%)_Space_57_MB_(93.62%) | ||
|
||
import com_github_leetcode.TreeNode | ||
|
||
/* | ||
* Definition for a binary tree node. | ||
* class TreeNode(_value: Int = 0, _left: TreeNode = null, _right: TreeNode = null) { | ||
* var value: Int = _value | ||
* var left: TreeNode = _left | ||
* var right: TreeNode = _right | ||
* } | ||
*/ | ||
object Solution { | ||
def isValidBST(root: TreeNode): Boolean = { | ||
def solve(node: TreeNode, left: Long, right: Long): Boolean = { | ||
if (node == null) { | ||
true | ||
} else if (node.value <= left || node.value >= right) { | ||
false | ||
} else { | ||
solve(node.left, left, node.value) && solve(node.right, node.value, right) | ||
} | ||
} | ||
|
||
solve(root, Long.MinValue, Long.MaxValue) | ||
} | ||
} |
Oops, something went wrong.