-
Notifications
You must be signed in to change notification settings - Fork 333
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Adds Random Resized Crop #457
Conversation
Sample code to test it: import matplotlib.pyplot as plt
import tensorflow as tf
from keras_cv.layers.preprocessing.random_resized_crop import RandomResizedCrop
SIZE = (300, 300)
elephants = tf.keras.utils.get_file(
"african_elephant.jpg", "https://i.imgur.com/Bvro0YD.png"
)
elephants = tf.keras.utils.load_img(elephants, target_size=SIZE)
elephants = tf.keras.utils.img_to_array(elephants) / 255
augmenter = RandomResizedCrop(area_factor=0.75, aspect_ratio_factor=0.25)
elephants = augmenter(elephants).numpy()
plt.imsave("aug_image.png", elephants) |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
This looks wonderful! Great work
class RandomResizedCrop(tf.keras.__internal__.layers.BaseImageAugmentationLayer): | ||
"""Randomly crops an image and resizes it to its original resolution. | ||
Args: | ||
TODO |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
lets document these factors, great job!!!
): | ||
super().__init__(seed=seed, **kwargs) | ||
self.area_factor = preprocessing.parse_factor( | ||
area_factor, param_name="area_factor", seed=seed |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
are there high and low bounds on this value? Be sure to document them, and pass them to min
and max
in parse_factor
@beresandras let me know if you need help adding test cases! This is looking fantastic! Thanks for your swift contribution! |
FYI @beresandras I updated the API a bit. |
Some notes:
|
Hey @beresandras are you intending to finish this PR up? If not, we can try to find someone to run it across the finish line! No worries either way. |
Yes, I will finish it in the next few days. |
@LukeWood unfortunately it seems that I won't be able to finish the PR for another week. Please try to find someone to complete it if you can. |
Thanks for letting me know. I will try to do so! We can also pick back up next week if needed |
I would like to work on this one. I'll create a PR asap. |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Minor changes
|
||
|
||
@tf.keras.utils.register_keras_serializable(package="keras_cv") | ||
class RandomResizedCrop(tf.keras.__internal__.layers.BaseImageAugmentationLayer): |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
lets use keras_cv.layers.BaseImageAugmentationLayer going forward
interpolation: interpolation method used in the `ImageProjectiveTransformV3` op. | ||
Supported values are `"nearest"` and `"bilinear"`. | ||
Defaults to `"bilinear"`. | ||
aspect_ratio_factor: (Optional) A tuple of two floats, a single float or |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I think aspect ratios respect the aspect ratio convention, 1.0 does not change the image. aspect_ratio=4/3 shifts aspect ratio by 4/3, etc.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
As such, we should default this ratio to (1, 1) so it performs a no-op. I don't think distorting aspect ratio should be a default behavior.
Perhaps we make this argument required?
Thanks for the contribution! Closing in favor of #499 |
* Created random resized crop files * Added test from #457 * Used `tf.image.crop_and_resize` instead of `ImageProjectionTransformV3` * Minor bug * Formatted * Reformatted * Update keras_cv/layers/preprocessing/random_resized_crop.py Co-authored-by: Sayak Paul <[email protected]> * Apply suggestions from code review Co-authored-by: Sayak Paul <[email protected]> * Doc changes * Doc changes * Made requested changes * Reformatted and tested * Update keras_cv/layers/preprocessing/random_resized_crop.py Co-authored-by: Sayak Paul <[email protected]> * Made requested changes * Created random resized crop files * Added test from #457 * Used `tf.image.crop_and_resize` instead of `ImageProjectionTransformV3` * Minor bug * Formatted * Reformatted * Doc changes * Update keras_cv/layers/preprocessing/random_resized_crop.py Co-authored-by: Sayak Paul <[email protected]> * Apply suggestions from code review Co-authored-by: Sayak Paul <[email protected]> * Doc changes * Made requested changes * Reformatted and tested * Luke edits * remove merge conflict * Fix broken test cases * Made changes to rrc * Minor changes * Added checks * Added checks * Added checks for inputs * Docstring updates * Made requested changes * Minor changes * Added demo * Formatted Co-authored-by: Sayak Paul <[email protected]> Co-authored-by: Luke Wood <[email protected]>
* Created random resized crop files * Added test from keras-team#457 * Used `tf.image.crop_and_resize` instead of `ImageProjectionTransformV3` * Minor bug * Formatted * Reformatted * Update keras_cv/layers/preprocessing/random_resized_crop.py Co-authored-by: Sayak Paul <[email protected]> * Apply suggestions from code review Co-authored-by: Sayak Paul <[email protected]> * Doc changes * Doc changes * Made requested changes * Reformatted and tested * Update keras_cv/layers/preprocessing/random_resized_crop.py Co-authored-by: Sayak Paul <[email protected]> * Made requested changes * Created random resized crop files * Added test from keras-team#457 * Used `tf.image.crop_and_resize` instead of `ImageProjectionTransformV3` * Minor bug * Formatted * Reformatted * Doc changes * Update keras_cv/layers/preprocessing/random_resized_crop.py Co-authored-by: Sayak Paul <[email protected]> * Apply suggestions from code review Co-authored-by: Sayak Paul <[email protected]> * Doc changes * Made requested changes * Reformatted and tested * Luke edits * remove merge conflict * Fix broken test cases * Made changes to rrc * Minor changes * Added checks * Added checks * Added checks for inputs * Docstring updates * Made requested changes * Minor changes * Added demo * Formatted Co-authored-by: Sayak Paul <[email protected]> Co-authored-by: Luke Wood <[email protected]>
* Created random resized crop files * Added test from keras-team#457 * Used `tf.image.crop_and_resize` instead of `ImageProjectionTransformV3` * Minor bug * Formatted * Reformatted * Update keras_cv/layers/preprocessing/random_resized_crop.py Co-authored-by: Sayak Paul <[email protected]> * Apply suggestions from code review Co-authored-by: Sayak Paul <[email protected]> * Doc changes * Doc changes * Made requested changes * Reformatted and tested * Update keras_cv/layers/preprocessing/random_resized_crop.py Co-authored-by: Sayak Paul <[email protected]> * Made requested changes * Created random resized crop files * Added test from keras-team#457 * Used `tf.image.crop_and_resize` instead of `ImageProjectionTransformV3` * Minor bug * Formatted * Reformatted * Doc changes * Update keras_cv/layers/preprocessing/random_resized_crop.py Co-authored-by: Sayak Paul <[email protected]> * Apply suggestions from code review Co-authored-by: Sayak Paul <[email protected]> * Doc changes * Made requested changes * Reformatted and tested * Luke edits * remove merge conflict * Fix broken test cases * Made changes to rrc * Minor changes * Added checks * Added checks * Added checks for inputs * Docstring updates * Made requested changes * Minor changes * Added demo * Formatted Co-authored-by: Sayak Paul <[email protected]> Co-authored-by: Luke Wood <[email protected]>
This PR implements the Random Resized Crop augmentation, currently minimally.
Example augmentations of the elephant image from the keras.io guide:
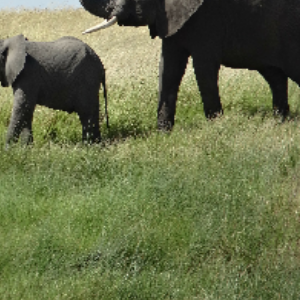
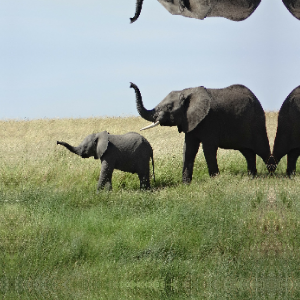
Corresponding issue: #131
Details to discuss:
area_factor
andaspect_ratio_factor
is used, both between 0 and 1, following the API design guidelines. They are translated to new areas and aspect ratios via new_value = 1 +/- factor(). The maximal area_factor of exactly 1.0 can lead to a crop with 0% or 200% area.ImageProjectiveTransformV3
operation. It could be done with tf.image.crop_and_resize() as well, but it does not have afill_mode
parameter.BaseImageAugmentationLayer
API.ImageProjectiveTransformV3
does not have an antialias argument, in contrast to tf.image.resize().TODO: